mirror of
https://github.com/eclipse/upm.git
synced 2025-07-13 07:11:14 +03:00
grove: add grove button support and examples
Signed-off-by: Sarah Knepper <sarah.knepper@intel.com> Signed-off-by: Brendan Le Foll <brendan.le.foll@intel.com>
This commit is contained in:
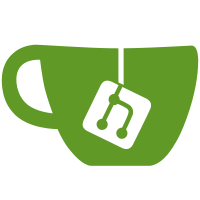
committed by
Brendan Le Foll
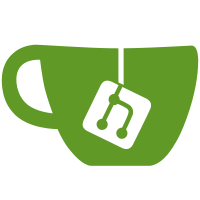
parent
c5369315e9
commit
801209678b
@ -1,6 +1,7 @@
|
|||||||
add_executable (hmc5883l-example hmc5883l.cxx)
|
add_executable (hmc5883l-example hmc5883l.cxx)
|
||||||
add_executable (groveled-example groveled.cxx)
|
add_executable (groveled-example groveled.cxx)
|
||||||
add_executable (grovetemp-example grovetemp.cxx)
|
add_executable (grovetemp-example grovetemp.cxx)
|
||||||
|
add_executable (grovebutton-example grovebutton.cxx)
|
||||||
add_executable (groverotary-example groverotary.cxx)
|
add_executable (groverotary-example groverotary.cxx)
|
||||||
add_executable (groveslide-example groveslide.cxx)
|
add_executable (groveslide-example groveslide.cxx)
|
||||||
add_executable (lcm-lcd-example lcm-lcd.cxx)
|
add_executable (lcm-lcd-example lcm-lcd.cxx)
|
||||||
@ -85,6 +86,7 @@ include_directories (${PROJECT_SOURCE_DIR}/src/ldt0028)
|
|||||||
target_link_libraries (hmc5883l-example hmc5883l ${CMAKE_THREAD_LIBS_INIT})
|
target_link_libraries (hmc5883l-example hmc5883l ${CMAKE_THREAD_LIBS_INIT})
|
||||||
target_link_libraries (groveled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
target_link_libraries (groveled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||||
target_link_libraries (grovetemp-example grove ${CMAKE_THREAD_LIBS_INIT})
|
target_link_libraries (grovetemp-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||||
|
target_link_libraries (grovebutton-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||||
target_link_libraries (groverotary-example grove ${CMAKE_THREAD_LIBS_INIT})
|
target_link_libraries (groverotary-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||||
target_link_libraries (groveslide-example grove ${CMAKE_THREAD_LIBS_INIT})
|
target_link_libraries (groveslide-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||||
target_link_libraries (lcm-lcd-example i2clcd ${CMAKE_THREAD_LIBS_INIT})
|
target_link_libraries (lcm-lcd-example i2clcd ${CMAKE_THREAD_LIBS_INIT})
|
||||||
|
49
examples/grovebutton.cxx
Normal file
49
examples/grovebutton.cxx
Normal file
@ -0,0 +1,49 @@
|
|||||||
|
/*
|
||||||
|
* Author: Sarah Knepper <sarah.knepper@intel.com>
|
||||||
|
* Copyright (c) 2014 Intel Corporation.
|
||||||
|
*
|
||||||
|
* Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
* a copy of this software and associated documentation files (the
|
||||||
|
* "Software"), to deal in the Software without restriction, including
|
||||||
|
* without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
* permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
* the following conditions:
|
||||||
|
*
|
||||||
|
* The above copyright notice and this permission notice shall be
|
||||||
|
* included in all copies or substantial portions of the Software.
|
||||||
|
*
|
||||||
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
*/
|
||||||
|
|
||||||
|
#include <unistd.h>
|
||||||
|
#include <iostream>
|
||||||
|
#include "grove.h"
|
||||||
|
|
||||||
|
int
|
||||||
|
main(int argc, char **argv)
|
||||||
|
{
|
||||||
|
// This example uses GPIO 0
|
||||||
|
//! [Interesting]
|
||||||
|
|
||||||
|
// Create the button object using GPIO pin 0
|
||||||
|
upm::GroveButton* button = new upm::GroveButton(0);
|
||||||
|
|
||||||
|
// Read the input and print, waiting one second between readings
|
||||||
|
while( 1 ) {
|
||||||
|
std::cout << button->name() << " value is " << button->value() << std::endl;
|
||||||
|
sleep(1);
|
||||||
|
}
|
||||||
|
|
||||||
|
// Delete the button object
|
||||||
|
delete button;
|
||||||
|
//! [Interesting]
|
||||||
|
|
||||||
|
return 0;
|
||||||
|
}
|
35
examples/javascript/grovebutton.js
Normal file
35
examples/javascript/grovebutton.js
Normal file
@ -0,0 +1,35 @@
|
|||||||
|
/*
|
||||||
|
* Author: Sarah Knepper <sarah.knepper@intel.com>
|
||||||
|
* Copyright (c) 2014 Intel Corporation.
|
||||||
|
*
|
||||||
|
* Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
* a copy of this software and associated documentation files (the
|
||||||
|
* "Software"), to deal in the Software without restriction, including
|
||||||
|
* without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
* permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
* the following conditions:
|
||||||
|
*
|
||||||
|
* The above copyright notice and this permission notice shall be
|
||||||
|
* included in all copies or substantial portions of the Software.
|
||||||
|
*
|
||||||
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
*/
|
||||||
|
|
||||||
|
// Load Grove module
|
||||||
|
var groveSensor = require('jsupm_grove');
|
||||||
|
|
||||||
|
// Create the button object using GPIO pin 0
|
||||||
|
var button = new groveSensor.GroveButton(0);
|
||||||
|
|
||||||
|
// Read the input and print, waiting one second between readings
|
||||||
|
function readButtonValue() {
|
||||||
|
console.log(button.name() + " value is " + button.value());
|
||||||
|
}
|
||||||
|
setInterval(readButtonValue, 1000);
|
35
examples/python/grovebutton.py
Normal file
35
examples/python/grovebutton.py
Normal file
@ -0,0 +1,35 @@
|
|||||||
|
# Author: Sarah Knepper <sarah.knepper@intel.com>
|
||||||
|
# Copyright (c) 2014 Intel Corporation.
|
||||||
|
#
|
||||||
|
# Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
# a copy of this software and associated documentation files (the
|
||||||
|
# "Software"), to deal in the Software without restriction, including
|
||||||
|
# without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
# distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
# permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
# the following conditions:
|
||||||
|
#
|
||||||
|
# The above copyright notice and this permission notice shall be
|
||||||
|
# included in all copies or substantial portions of the Software.
|
||||||
|
#
|
||||||
|
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
|
||||||
|
import time
|
||||||
|
import pyupm_grove as grove
|
||||||
|
|
||||||
|
# Create the button object using GPIO pin 0
|
||||||
|
button = grove.GroveButton(0)
|
||||||
|
|
||||||
|
# Read the input and print, waiting one second between readings
|
||||||
|
while 1:
|
||||||
|
print button.name(), ' value is ', button.value()
|
||||||
|
time.sleep(1)
|
||||||
|
|
||||||
|
# Delete the button object
|
||||||
|
del button
|
@ -193,3 +193,28 @@ float GroveSlide::ref_voltage()
|
|||||||
{
|
{
|
||||||
return m_ref_voltage;
|
return m_ref_voltage;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
//// GroveButton ////
|
||||||
|
|
||||||
|
GroveButton::GroveButton(unsigned int pin)
|
||||||
|
{
|
||||||
|
mraa_init();
|
||||||
|
m_gpio = mraa_gpio_init(pin);
|
||||||
|
mraa_gpio_dir(m_gpio, MRAA_GPIO_IN);
|
||||||
|
m_name = "Button Sensor";
|
||||||
|
}
|
||||||
|
|
||||||
|
GroveButton::~GroveButton()
|
||||||
|
{
|
||||||
|
mraa_gpio_close(m_gpio);
|
||||||
|
}
|
||||||
|
|
||||||
|
std::string GroveButton::name()
|
||||||
|
{
|
||||||
|
return m_name;
|
||||||
|
}
|
||||||
|
|
||||||
|
int GroveButton::value()
|
||||||
|
{
|
||||||
|
return mraa_gpio_read(m_gpio);
|
||||||
|
}
|
||||||
|
@ -247,4 +247,41 @@ class GroveSlide: public Grove {
|
|||||||
float m_ref_voltage;
|
float m_ref_voltage;
|
||||||
};
|
};
|
||||||
|
|
||||||
|
/**
|
||||||
|
* @brief C++ API for Grove button
|
||||||
|
*
|
||||||
|
* Very basic UPM module for Grove button
|
||||||
|
*
|
||||||
|
* @ingroup grove gpio
|
||||||
|
* @snippet grovebutton.cxx Interesting
|
||||||
|
*/
|
||||||
|
class GroveButton: public Grove {
|
||||||
|
public:
|
||||||
|
/**
|
||||||
|
* Grove button constructor
|
||||||
|
*
|
||||||
|
* @param gpio pin to use
|
||||||
|
*/
|
||||||
|
GroveButton(unsigned int pin);
|
||||||
|
/**
|
||||||
|
* Grove button destructor
|
||||||
|
*/
|
||||||
|
~GroveButton();
|
||||||
|
/**
|
||||||
|
* Get name of sensor
|
||||||
|
*
|
||||||
|
* @return the name of this sensor
|
||||||
|
*/
|
||||||
|
std::string name();
|
||||||
|
/**
|
||||||
|
* Get value from GPIO pin
|
||||||
|
*
|
||||||
|
* @return the value from the GPIO pin
|
||||||
|
*/
|
||||||
|
int value();
|
||||||
|
private:
|
||||||
|
std::string m_name;
|
||||||
|
mraa_gpio_context m_gpio;
|
||||||
|
};
|
||||||
|
|
||||||
}
|
}
|
||||||
|
Reference in New Issue
Block a user