mirror of
https://github.com/eclipse/upm.git
synced 2025-07-13 07:11:14 +03:00
cwlsxxa: Initial implementation
The driver was developed using the Veris CWLSHTA CO2 Gas sensor. The 'T' variant supports a temperature sensor, and the 'H' variant supports a humidity sensor. All 3 signals are provided by the device as analog 0-5Vdc, 0-10Vdc, or 4-20ma loop current outputs. For devices supporting temperature, the valid temperature range is 10C to 50C. The humidity ranges from 0% to 100% (non-condensing). The CO2 sensor ranges from 0 to 2000 ppm. This driver was developed using the 5Vdc outputs and the 4-20ma outputs. For voltage outputs, your MCU must be configured for 5V operation. In addition, you must configure the sensor (via it's configuration switches) to output 0-5VDC only. Using any other analog reference voltage will require the appropriate external circuitry (such as a voltage divider) in order to interface safely with your MCU. In addition, the sensor can be configured for 4-20ma usage, by specifying the correct receiver resistance (in ohms) in the constructor. This sensor was tested with a Cooking Hacks (Libelium) 4-channel 4-20ma Arduino interface shield. For this interface, the receiver resistance was specified as 165.0 ohms. Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Abhishek Malik <abhishek.malik@intel.com>
This commit is contained in:
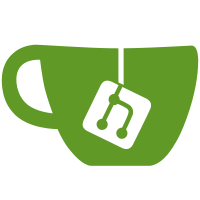
committed by
Abhishek Malik
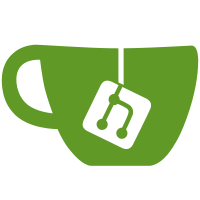
parent
f3a2e0d77c
commit
a8d96bd2d9
@ -248,6 +248,7 @@ add_example (max44009)
|
||||
add_example (si1132)
|
||||
add_example (si7005)
|
||||
add_example (t6713)
|
||||
add_example (cwlsxxa)
|
||||
|
||||
# These are special cases where you specify example binary, source file and module(s)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src)
|
||||
|
82
examples/c++/cwlsxxa.cxx
Normal file
82
examples/c++/cwlsxxa.cxx
Normal file
@ -0,0 +1,82 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2016 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
|
||||
#include "cwlsxxa.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
bool shouldRun = true;
|
||||
|
||||
void sig_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
shouldRun = false;
|
||||
}
|
||||
|
||||
int main(int argc, char **argv)
|
||||
{
|
||||
signal(SIGINT, sig_handler);
|
||||
|
||||
//! [Interesting]
|
||||
|
||||
cout << "Initializing..." << endl;
|
||||
|
||||
// Instantiate an CWLSXXA instance, using A0 for CO2, A1 for
|
||||
// humidity and A2 for temperature
|
||||
upm::CWLSXXA *sensor = new upm::CWLSXXA(0, 1, 2);
|
||||
|
||||
// update and print available values every second
|
||||
while (shouldRun)
|
||||
{
|
||||
// update our values from the sensor
|
||||
sensor->update();
|
||||
|
||||
// we show both C and F for temperature
|
||||
cout << "Temperature: " << sensor->getTemperature()
|
||||
<< " C / " << sensor->getTemperature(true) << " F"
|
||||
<< endl;
|
||||
|
||||
cout << "Humidity: " << sensor->getHumidity()
|
||||
<< " %" << endl;
|
||||
|
||||
cout << "CO2: " << sensor->getCO2()
|
||||
<< " ppm" << endl;
|
||||
|
||||
cout << endl;
|
||||
|
||||
sleep(1);
|
||||
}
|
||||
|
||||
cout << "Exiting..." << endl;
|
||||
|
||||
delete sensor;
|
||||
|
||||
//! [Interesting]
|
||||
|
||||
return 0;
|
||||
}
|
@ -105,6 +105,7 @@ add_example(Ad8232Example ad8232)
|
||||
add_example(Gp2y0aExample gp2y0a)
|
||||
add_example(Th02Example th02)
|
||||
add_example(FlexSensorExample flex)
|
||||
add_example(CWLSXXA_Example cwlsxxa)
|
||||
|
||||
|
||||
add_example_with_path(Jhd1313m1_lcdSample lcd/upm_i2clcd.jar)
|
||||
|
62
examples/java/CWLSXXA_Example.java
Normal file
62
examples/java/CWLSXXA_Example.java
Normal file
@ -0,0 +1,62 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2016 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
import upm_cwlsxxa.CWLSXXA;
|
||||
|
||||
public class CWLSXXA_Example
|
||||
{
|
||||
public static void main(String[] args) throws InterruptedException
|
||||
{
|
||||
// ! [Interesting]
|
||||
|
||||
// Instantiate an CWLSXXA instance, using A0 for CO2, A1 for
|
||||
// humidity and A2 for temperature
|
||||
CWLSXXA sensor = new CWLSXXA(0, 1, 2);
|
||||
|
||||
while (true)
|
||||
{
|
||||
// update our values from the sensor
|
||||
sensor.update();
|
||||
|
||||
// we show both C and F for temperature
|
||||
System.out.println("Temperature: "
|
||||
+ sensor.getTemperature()
|
||||
+ " C / "
|
||||
+ sensor.getTemperature(true)
|
||||
+ " F");
|
||||
|
||||
System.out.println("Humidity: "
|
||||
+ sensor.getHumidity()
|
||||
+ " %");
|
||||
|
||||
System.out.println("CO2: "
|
||||
+ sensor.getCO2()
|
||||
+ " ppm");
|
||||
|
||||
Thread.sleep(1000);
|
||||
}
|
||||
|
||||
// ! [Interesting]
|
||||
}
|
||||
}
|
66
examples/javascript/cwlsxxa.js
Normal file
66
examples/javascript/cwlsxxa.js
Normal file
@ -0,0 +1,66 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2016 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
|
||||
var sensorObj = require('jsupm_cwlsxxa');
|
||||
|
||||
|
||||
/************** Main code **************/
|
||||
|
||||
console.log("Initializing...");
|
||||
|
||||
// Instantiate an CWLSXXA instance, using A0 for CO2, A1 for
|
||||
// humidity and A2 for temperature
|
||||
var sensor = new sensorObj.CWLSXXA(0, 1, 2);
|
||||
|
||||
// update and print available values every second
|
||||
setInterval(function()
|
||||
{
|
||||
// update our values from the sensor
|
||||
sensor.update();
|
||||
|
||||
// we show both C and F for temperature
|
||||
console.log("Temperature:", sensor.getTemperature(),
|
||||
"C /", sensor.getTemperature(true), "F");
|
||||
|
||||
console.log("Humidity:", sensor.getHumidity(), "%");
|
||||
|
||||
console.log("CO2:", sensor.getCO2(), "ppm");
|
||||
|
||||
console.log("");
|
||||
|
||||
}, 1000);
|
||||
|
||||
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
sensor = null;
|
||||
sensorObj.cleanUp();
|
||||
sensorObj = null;
|
||||
console.log("Exiting...");
|
||||
process.exit(0);
|
||||
});
|
61
examples/python/cwlsxxa.py
Normal file
61
examples/python/cwlsxxa.py
Normal file
@ -0,0 +1,61 @@
|
||||
#!/usr/bin/python
|
||||
# Author: Jon Trulson <jtrulson@ics.com>
|
||||
# Copyright (c) 2016 Intel Corporation.
|
||||
#
|
||||
# Permission is hereby granted, free of charge, to any person obtaining
|
||||
# a copy of this software and associated documentation files (the
|
||||
# "Software"), to deal in the Software without restriction, including
|
||||
# without limitation the rights to use, copy, modify, merge, publish,
|
||||
# distribute, sublicense, and/or sell copies of the Software, and to
|
||||
# permit persons to whom the Software is furnished to do so, subject to
|
||||
# the following conditions:
|
||||
#
|
||||
# The above copyright notice and this permission notice shall be
|
||||
# included in all copies or substantial portions of the Software.
|
||||
#
|
||||
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
||||
import time, sys, signal, atexit
|
||||
import pyupm_cwlsxxa as sensorObj
|
||||
|
||||
## Exit handlers ##
|
||||
# This function stops python from printing a stacktrace when you hit control-C
|
||||
def SIGINTHandler(signum, frame):
|
||||
raise SystemExit
|
||||
|
||||
# This function lets you run code on exit
|
||||
def exitHandler():
|
||||
print "Exiting"
|
||||
sys.exit(0)
|
||||
|
||||
# Register exit handlers
|
||||
atexit.register(exitHandler)
|
||||
signal.signal(signal.SIGINT, SIGINTHandler)
|
||||
|
||||
print "Initializing..."
|
||||
|
||||
# Instantiate an CWLSXXA instance, using A0 for CO2, A1 for
|
||||
# humidity and A2 for temperature
|
||||
sensor = sensorObj.CWLSXXA(0, 1, 2)
|
||||
|
||||
# update and print available values every second
|
||||
while (1):
|
||||
# update our values from the sensor
|
||||
sensor.update()
|
||||
|
||||
# we show both C and F for temperature
|
||||
print "Temperature:", sensor.getTemperature(), "C /",
|
||||
print sensor.getTemperature(True), "F"
|
||||
|
||||
print "Humidity:", sensor.getHumidity(), "%"
|
||||
|
||||
print "CO2:", sensor.getCO2(), "ppm"
|
||||
|
||||
print
|
||||
time.sleep(1)
|
5
src/cwlsxxa/CMakeLists.txt
Normal file
5
src/cwlsxxa/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
||||
set (libname "cwlsxxa")
|
||||
set (libdescription "upm Veris CWLSXXA CO2/Temperature/Humidity transmitter")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
183
src/cwlsxxa/cwlsxxa.cxx
Normal file
183
src/cwlsxxa/cwlsxxa.cxx
Normal file
@ -0,0 +1,183 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2016 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
|
||||
#include "cwlsxxa.h"
|
||||
|
||||
using namespace upm;
|
||||
using namespace std;
|
||||
|
||||
// for current loop reads that seems a little noisy, we average over
|
||||
// several aio reads.
|
||||
static const int maxSamples = 50;
|
||||
|
||||
// conversion from celcius to fahrenheit
|
||||
|
||||
static float c2f(float c)
|
||||
{
|
||||
return (c * (9.0 / 5.0) + 32.0);
|
||||
}
|
||||
|
||||
|
||||
CWLSXXA::CWLSXXA(int gPin, int hPin, int tPin, float rResistor, float aref) :
|
||||
m_aioCO2(gPin), m_aioHum(0), m_aioTemp(0)
|
||||
{
|
||||
m_hasHum = (hPin >= 0) ? true : false;
|
||||
m_hasTemp = (tPin >= 0) ? true : false;
|
||||
|
||||
if (m_hasTemp)
|
||||
{
|
||||
m_aioTemp = new mraa::Aio(tPin);
|
||||
m_aResTemp = (1 << m_aioTemp->getBit());
|
||||
}
|
||||
else
|
||||
m_aResTemp = 0;
|
||||
|
||||
if (m_hasHum)
|
||||
{
|
||||
m_aioHum = new mraa::Aio(hPin);
|
||||
m_aResHum = (1 << m_aioHum->getBit());
|
||||
}
|
||||
else
|
||||
m_aResHum = 0;
|
||||
|
||||
m_aResCO2 = (1 << m_aioCO2.getBit());
|
||||
|
||||
m_temperature = 0.0;
|
||||
m_humidity = 0.0;
|
||||
m_co2 = 0.0;
|
||||
|
||||
m_aref = aref;
|
||||
m_rResistor = rResistor;
|
||||
}
|
||||
|
||||
CWLSXXA::~CWLSXXA()
|
||||
{
|
||||
if (m_aioHum)
|
||||
delete m_aioHum;
|
||||
if (m_aioTemp)
|
||||
delete m_aioTemp;
|
||||
}
|
||||
|
||||
void CWLSXXA::update()
|
||||
{
|
||||
// temperature
|
||||
int val;
|
||||
float volts;
|
||||
float milliamps = 0.0;
|
||||
|
||||
int samples;
|
||||
if (m_rResistor)
|
||||
samples = maxSamples;
|
||||
else
|
||||
samples = 1;
|
||||
|
||||
|
||||
// temperature
|
||||
if (m_hasTemp)
|
||||
{
|
||||
// fortunately, this sensor always reports temperatures in C,
|
||||
// regardless of the configuration of the LCD display (for
|
||||
// models that have an LCD display)
|
||||
|
||||
val = average(m_aioTemp, samples);
|
||||
|
||||
volts = (float(val) * (m_aref / m_aResTemp));
|
||||
|
||||
// valid range is 10-35C, current loop range is 16ma (20ma - 4ma)
|
||||
if (!m_rResistor)
|
||||
m_temperature = ((volts / m_aref) * 25.0) + 10.0;
|
||||
else
|
||||
{
|
||||
milliamps = ((volts / m_rResistor * 1000.0) - 4.0);
|
||||
if (milliamps < 0.0) // not connected
|
||||
milliamps = 0.0;
|
||||
m_temperature = (milliamps * (25.0 / 16.0)) + 10.0;
|
||||
}
|
||||
}
|
||||
|
||||
// humidity
|
||||
if (m_hasHum)
|
||||
{
|
||||
val = average(m_aioHum, samples);
|
||||
volts = (float(val) * (m_aref / m_aResHum));
|
||||
|
||||
// range is 0-100
|
||||
if (!m_rResistor)
|
||||
m_humidity = ((volts / m_aref) * 100.0);
|
||||
else
|
||||
{
|
||||
milliamps = ((volts / m_rResistor * 1000.0) - 4.0);
|
||||
if (milliamps < 0.0) // not connected
|
||||
milliamps = 0.0;
|
||||
m_humidity = milliamps * (100.0 / 16.0);
|
||||
}
|
||||
}
|
||||
|
||||
// CO2
|
||||
val = average(&m_aioCO2, samples);
|
||||
volts = (float(val) * (m_aref / m_aResCO2));
|
||||
|
||||
// CO2 range is 0-2000ppm
|
||||
if (!m_rResistor)
|
||||
m_co2 = ((volts / m_aref) * 2000.0);
|
||||
else
|
||||
{
|
||||
milliamps = ((volts / m_rResistor * 1000.0) - 4.0);
|
||||
if (milliamps < 0.0) // not connected
|
||||
milliamps = 0.0;
|
||||
m_co2 = milliamps * (2000.0 / 16.0);
|
||||
}
|
||||
}
|
||||
|
||||
float CWLSXXA::getTemperature(bool fahrenheit)
|
||||
{
|
||||
if (fahrenheit)
|
||||
return c2f(m_temperature);
|
||||
else
|
||||
return m_temperature;
|
||||
}
|
||||
|
||||
float CWLSXXA::getHumidity()
|
||||
{
|
||||
return m_humidity;
|
||||
}
|
||||
|
||||
float CWLSXXA::getCO2()
|
||||
{
|
||||
return m_co2;
|
||||
}
|
||||
|
||||
int CWLSXXA::average(mraa::Aio *aio, int samples)
|
||||
{
|
||||
if (samples <= 0)
|
||||
samples = 1;
|
||||
|
||||
int avg = 0;
|
||||
for (int i=0; i<samples; i++)
|
||||
avg += aio->read();
|
||||
|
||||
return (avg / samples);
|
||||
}
|
189
src/cwlsxxa/cwlsxxa.h
Normal file
189
src/cwlsxxa/cwlsxxa.h
Normal file
@ -0,0 +1,189 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2016 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <iostream>
|
||||
|
||||
#include <mraa/aio.hpp>
|
||||
|
||||
// Unlikey to be changable without external circuitry (voltage divider)
|
||||
#define CWLSXXA_DEFAULT_AREF 5.0
|
||||
|
||||
namespace upm {
|
||||
/**
|
||||
* @brief Veris CWLSXXA CO2 Sensor
|
||||
* @defgroup cwlsxxa libupm-cwlsxxa
|
||||
* @ingroup veris ainput temp gaseous
|
||||
*/
|
||||
|
||||
/**
|
||||
* @library cwlsxxa
|
||||
* @sensor cwlsxxa
|
||||
* @comname Veris CWLSXXA CO2 Sensor Family
|
||||
* @type temp gaseous
|
||||
* @man veris
|
||||
* @con ainput
|
||||
* @web http://www.veris.com/Item/CWLSHTA.aspx
|
||||
*
|
||||
* @brief API for the Veris CWLSXXA CO2 Sensor Family
|
||||
*
|
||||
* The driver was developed using the CWLSHTA CO2 Gas sensor. The
|
||||
* 'T' variant supports a temperature transmitter, and the 'H'
|
||||
* variant supports a humidity sensor. All 3 signals are provided
|
||||
* by the device as analog 0-5Vdc, 0-10Vdc, or 4-20ma loop current
|
||||
* outputs. For devices supporting temperature, the valid
|
||||
* temperature range is 10C to 50C. The humidity ranges from 0%
|
||||
* to 100% (non-condensing). The CO2 sensor ranges from 0 to 2000
|
||||
* ppm.
|
||||
*
|
||||
* This driver was developed using the 5Vdc outputs and the 4-20ma
|
||||
* outputs. For voltage outputs, your MCU must be configured for
|
||||
* 5V operation. In addition, you must configure the sensor (via
|
||||
* it's configuration switches) to output 0-5VDC only. Using any
|
||||
* other analog reference voltage will require the appropriate
|
||||
* external circuitry (such as a voltage divider) in order to
|
||||
* interface safely with your MCU.
|
||||
*
|
||||
* In addition, the sensor can be configured for 4-20ma usage, by
|
||||
* specifying the correct receiver resistance (in ohms) in the
|
||||
* constructor. This sensor was tested with a Cooking Hacks
|
||||
* (Libelium) 4-channel 4-20ma Arduino interface shield. For this
|
||||
* interface, the receiver resistance was specified as 165.0 ohms.
|
||||
*
|
||||
* For devices which do not support temperature, use '-1' as the
|
||||
* temperature pin number in the object constructor. If
|
||||
* temperature measurement is disabled, getTemperature() will always
|
||||
* return 0C/32F.
|
||||
*
|
||||
* For devices which do not support humidity, use '-1' as the
|
||||
* temperature pin number in the object constructor. If
|
||||
* humidity measurement is disabled, getHumidity() will always
|
||||
* return 0.
|
||||
*
|
||||
* @snippet cwlsxxa.cxx Interesting
|
||||
*/
|
||||
|
||||
class CWLSXXA {
|
||||
public:
|
||||
|
||||
/**
|
||||
* CWLSXXA object constructor
|
||||
*
|
||||
* @param gPin Analog pin to use for the CO2 measurement
|
||||
* @param hPin Analog pin to use for the humidity measurement. If
|
||||
* your device does not support humidity, use -1 as the value so
|
||||
* that humidity will not be queried and an analog pin won't be
|
||||
* wasted.
|
||||
* @param tPin Analog pin to use for temperature. If your device
|
||||
* does not support temperature, use -1 as the value so that
|
||||
* temperature will not be queried and an analog pin won't be
|
||||
* wasted.
|
||||
* @param rResistor The receiver resistance in ohms, when using a
|
||||
* 4-20ma current loop interface. When specified, this value will
|
||||
* be used in computing the current based on the voltage read when
|
||||
* scaling the return values. Default is 0.0, for standard
|
||||
* scaling based on voltage output rather than current (4-20ma
|
||||
* mode).
|
||||
* @param aref The analog reference voltage, default 5.0
|
||||
*/
|
||||
CWLSXXA(int gPin, int hPin, int tPin, float rResistor=0.0,
|
||||
float aref=CWLSXXA_DEFAULT_AREF);
|
||||
|
||||
/**
|
||||
* CWLSXXA object destructor
|
||||
*/
|
||||
~CWLSXXA();
|
||||
|
||||
/**
|
||||
* Read current values from the sensor and update internal stored
|
||||
* values. This method must be called prior to querying any
|
||||
* values, such as CO2, temperature, or humidity.
|
||||
*/
|
||||
void update();
|
||||
|
||||
/**
|
||||
* Get the current temperature. update() must have been called
|
||||
* prior to calling this method. If temperature measurement was
|
||||
* disabled (by passing -1 as the temperature pin in the
|
||||
* constructor) then this function will always return 0C/32F.
|
||||
*
|
||||
* @param fahrenheit true to return the temperature in degrees
|
||||
* fahrenheit, false to return the temperature in degrees celcius.
|
||||
* The default is false (degrees Celcius).
|
||||
* @return The last temperature reading in Celcius or Fahrenheit
|
||||
*/
|
||||
float getTemperature(bool fahrenheit=false);
|
||||
|
||||
/**
|
||||
* Get the current relative humidity. update() must have been called
|
||||
* prior to calling this method. If humidity measurement was
|
||||
* disabled (by passing -1 as the te pin in the
|
||||
* constructor) then this function will always return 0.
|
||||
*
|
||||
* @return The last humidity reading
|
||||
*/
|
||||
float getHumidity();
|
||||
|
||||
/**
|
||||
* Get the current CO2 concentration in Parts Per Million (PPM).
|
||||
* update() must have been called prior to calling this method.
|
||||
*
|
||||
* @return The last CO2 reading
|
||||
*/
|
||||
float getCO2();
|
||||
|
||||
|
||||
protected:
|
||||
// temperature and humidity are optional features of this transmitter
|
||||
mraa::Aio *m_aioTemp;
|
||||
mraa::Aio *m_aioHum;
|
||||
|
||||
// CO2 reporting is always supported
|
||||
mraa::Aio m_aioCO2;
|
||||
|
||||
private:
|
||||
float m_aref;
|
||||
float m_rResistor;
|
||||
int m_aResTemp;
|
||||
int m_aResHum;
|
||||
int m_aResCO2;
|
||||
|
||||
// does this sensor support temperature and/or humidity reporting?
|
||||
bool m_hasTemp;
|
||||
bool m_hasHum;
|
||||
|
||||
// in Celcius
|
||||
float m_temperature;
|
||||
|
||||
float m_humidity;
|
||||
|
||||
// in PPM
|
||||
float m_co2;
|
||||
|
||||
int average(mraa::Aio *aio, int samples);
|
||||
};
|
||||
}
|
||||
|
||||
|
19
src/cwlsxxa/javaupm_cwlsxxa.i
Normal file
19
src/cwlsxxa/javaupm_cwlsxxa.i
Normal file
@ -0,0 +1,19 @@
|
||||
%module javaupm_cwlsxxa
|
||||
%include "../upm.i"
|
||||
%include "std_string.i"
|
||||
|
||||
%include "cwlsxxa.h"
|
||||
%{
|
||||
#include "cwlsxxa.h"
|
||||
%}
|
||||
|
||||
%pragma(java) jniclasscode=%{
|
||||
static {
|
||||
try {
|
||||
System.loadLibrary("javaupm_cwlsxxa");
|
||||
} catch (UnsatisfiedLinkError e) {
|
||||
System.err.println("Native code library failed to load. \n" + e);
|
||||
System.exit(1);
|
||||
}
|
||||
}
|
||||
%}
|
10
src/cwlsxxa/jsupm_cwlsxxa.i
Normal file
10
src/cwlsxxa/jsupm_cwlsxxa.i
Normal file
@ -0,0 +1,10 @@
|
||||
%module jsupm_cwlsxxa
|
||||
%include "../upm.i"
|
||||
%include "std_string.i"
|
||||
|
||||
%{
|
||||
#include "cwlsxxa.h"
|
||||
%}
|
||||
|
||||
%include "cwlsxxa.h"
|
||||
|
13
src/cwlsxxa/pyupm_cwlsxxa.i
Normal file
13
src/cwlsxxa/pyupm_cwlsxxa.i
Normal file
@ -0,0 +1,13 @@
|
||||
// Include doxygen-generated documentation
|
||||
%include "pyupm_doxy2swig.i"
|
||||
%module pyupm_cwlsxxa
|
||||
%include "../upm.i"
|
||||
%include "std_string.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%{
|
||||
#include "cwlsxxa.h"
|
||||
%}
|
||||
%include "cwlsxxa.h"
|
||||
|
Reference in New Issue
Block a user