mirror of
https://github.com/eclipse/upm.git
synced 2025-07-01 09:21:12 +03:00
ADXL345: Add string based constructor
Signed-off-by: Adelin Dobre <adelin.dobre@rinftech.com>
This commit is contained in:
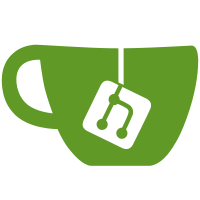
committed by
Stefan Andritoiu
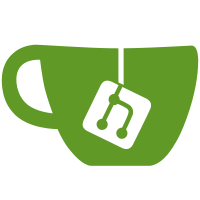
parent
ae55df8b75
commit
c7cca92fa7
@ -41,7 +41,6 @@ AD8232::AD8232(int loPlus, int loMinus, int output, float aref) {
|
||||
|
||||
m_aref = aref;
|
||||
m_ares = (1 << m_aioOUT->getBit());
|
||||
m_callcons = 0;
|
||||
}
|
||||
|
||||
AD8232::AD8232(std::string initStr)
|
||||
@ -89,21 +88,20 @@ AD8232::AD8232(std::string initStr)
|
||||
}
|
||||
}
|
||||
m_ares = (1 << m_aioOUT->getBit());
|
||||
m_callcons = 1;
|
||||
}
|
||||
|
||||
AD8232::~AD8232()
|
||||
{
|
||||
if(!m_callcons)
|
||||
if(mraaIo != NULL)
|
||||
{
|
||||
delete mraaIo;
|
||||
}
|
||||
else
|
||||
{
|
||||
delete m_gpioLOPlus;
|
||||
delete m_gpioLOMinus;
|
||||
delete m_aioOUT;
|
||||
}
|
||||
else
|
||||
{
|
||||
delete mraaIo;
|
||||
}
|
||||
}
|
||||
|
||||
int AD8232::value()
|
||||
|
@ -112,7 +112,6 @@ namespace upm {
|
||||
|
||||
float m_aref;
|
||||
int m_ares;
|
||||
int m_callcons;
|
||||
};
|
||||
}
|
||||
|
||||
|
@ -26,6 +26,7 @@
|
||||
#include <string>
|
||||
#include <stdexcept>
|
||||
#include <unistd.h>
|
||||
#include <utility>
|
||||
#include "math.h"
|
||||
#include "adxl345.hpp"
|
||||
|
||||
@ -87,10 +88,10 @@
|
||||
|
||||
using namespace upm;
|
||||
|
||||
Adxl345::Adxl345(int bus) : m_i2c(bus)
|
||||
Adxl345::Adxl345(int bus) : m_i2c(new mraa::I2c(bus))
|
||||
{
|
||||
//init bus and reset chip
|
||||
if ( m_i2c.address(ADXL345_I2C_ADDR) != mraa::SUCCESS ){
|
||||
if ( m_i2c->address(ADXL345_I2C_ADDR) != mraa::SUCCESS ){
|
||||
throw std::invalid_argument(std::string(__FUNCTION__) +
|
||||
": i2c.address() failed");
|
||||
return;
|
||||
@ -98,7 +99,7 @@ Adxl345::Adxl345(int bus) : m_i2c(bus)
|
||||
|
||||
m_buffer[0] = ADXL345_POWER_CTL;
|
||||
m_buffer[1] = ADXL345_POWER_ON;
|
||||
if( m_i2c.write(m_buffer, 2) != mraa::SUCCESS){
|
||||
if( m_i2c->write(m_buffer, 2) != mraa::SUCCESS){
|
||||
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||
": i2c.write() control register failed");
|
||||
return;
|
||||
@ -106,7 +107,7 @@ Adxl345::Adxl345(int bus) : m_i2c(bus)
|
||||
|
||||
m_buffer[0] = ADXL345_DATA_FORMAT;
|
||||
m_buffer[1] = ADXL345_16G | ADXL345_FULL_RES;
|
||||
if( m_i2c.write(m_buffer, 2) != mraa::SUCCESS){
|
||||
if( m_i2c->write(m_buffer, 2) != mraa::SUCCESS){
|
||||
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||
": i2c.write() mode register failed");
|
||||
return;
|
||||
@ -122,6 +123,58 @@ Adxl345::Adxl345(int bus) : m_i2c(bus)
|
||||
Adxl345::update();
|
||||
}
|
||||
|
||||
Adxl345::Adxl345(std::string initStr) : mraaIo(new mraa::MraaIo(initStr))
|
||||
{
|
||||
if(mraaIo == NULL)
|
||||
{
|
||||
throw std::invalid_argument(std::string(__FUNCTION__) +
|
||||
": Failed to allocate memory for internal member");
|
||||
}
|
||||
|
||||
if(!mraaIo->i2cs.empty())
|
||||
{
|
||||
m_i2c = &mraaIo->i2cs[0];
|
||||
}
|
||||
else
|
||||
{
|
||||
throw std::invalid_argument(std::string(__FUNCTION__) +
|
||||
": mraa_i2c_init() failed");
|
||||
}
|
||||
|
||||
m_buffer[0] = ADXL345_POWER_CTL;
|
||||
m_buffer[1] = ADXL345_POWER_ON;
|
||||
if( m_i2c->write(m_buffer, 2) != mraa::SUCCESS){
|
||||
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||
": i2c.write() control register failed");
|
||||
return;
|
||||
}
|
||||
|
||||
m_buffer[0] = ADXL345_DATA_FORMAT;
|
||||
m_buffer[1] = ADXL345_16G | ADXL345_FULL_RES;
|
||||
if( m_i2c->write(m_buffer, 2) != mraa::SUCCESS){
|
||||
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||
": i2c.write() mode register failed");
|
||||
return;
|
||||
}
|
||||
|
||||
//2.5V sensitivity is 256 LSB/g = 0.00390625 g/bit
|
||||
//3.3V x and y sensitivity is 265 LSB/g = 0.003773584 g/bit, z is the same
|
||||
|
||||
m_offsets[0] = 0.003773584;
|
||||
m_offsets[1] = 0.003773584;
|
||||
m_offsets[2] = 0.00390625;
|
||||
|
||||
Adxl345::update();
|
||||
}
|
||||
|
||||
Adxl345::~Adxl345()
|
||||
{
|
||||
if(mraaIo != NULL)
|
||||
delete mraaIo;
|
||||
else
|
||||
delete m_i2c;
|
||||
}
|
||||
|
||||
float*
|
||||
Adxl345::getAcceleration()
|
||||
{
|
||||
@ -142,9 +195,9 @@ Adxl345::getScale(){
|
||||
|
||||
uint8_t result;
|
||||
|
||||
m_i2c.writeByte(ADXL345_DATA_FORMAT);
|
||||
m_i2c->writeByte(ADXL345_DATA_FORMAT);
|
||||
|
||||
result = m_i2c.readByte();
|
||||
result = m_i2c->readByte();
|
||||
|
||||
return pow(2, (result & 0x03) + 1);
|
||||
}
|
||||
@ -152,9 +205,9 @@ Adxl345::getScale(){
|
||||
mraa::Result
|
||||
Adxl345::update(void)
|
||||
{
|
||||
m_i2c.writeByte(ADXL345_XOUT_L);
|
||||
m_i2c->writeByte(ADXL345_XOUT_L);
|
||||
|
||||
m_i2c.read(m_buffer, DATA_REG_SIZE);
|
||||
m_i2c->read(m_buffer, DATA_REG_SIZE);
|
||||
|
||||
// x
|
||||
m_rawaccel[0] = ((m_buffer[1] << 8 ) | m_buffer[0]);
|
||||
|
@ -24,6 +24,7 @@
|
||||
#pragma once
|
||||
|
||||
#include <mraa/i2c.hpp>
|
||||
#include <mraa/initio.hpp>
|
||||
|
||||
#define READ_BUFFER_LENGTH 6
|
||||
|
||||
@ -66,10 +67,18 @@ public:
|
||||
*/
|
||||
Adxl345(int bus);
|
||||
|
||||
/**
|
||||
* Instantiates ADXL345 Accelerometer based on a given string.
|
||||
*
|
||||
* @param initStr string containing specific information for ADXL345 initialization.
|
||||
*/
|
||||
Adxl345(std::string initStr);
|
||||
|
||||
/**
|
||||
* there is no need for a ADXL345 object destructor
|
||||
* ~Adxl345();
|
||||
*/
|
||||
~Adxl345();
|
||||
|
||||
/**
|
||||
* Returns a pointer to a float[3] that contains acceleration (g) forces
|
||||
@ -105,7 +114,8 @@ private:
|
||||
float m_offsets[3];
|
||||
int16_t m_rawaccel[3];
|
||||
uint8_t m_buffer[READ_BUFFER_LENGTH];
|
||||
mraa::I2c m_i2c;
|
||||
mraa::I2c* m_i2c = NULL;
|
||||
mraa::MraaIo* mraaIo = NULL;
|
||||
};
|
||||
|
||||
}
|
||||
|
Reference in New Issue
Block a user