mirror of
https://github.com/eclipse/upm.git
synced 2025-07-02 01:41:12 +03:00
adxl335: python example (and JS example modification) for adxl335 analog accelerometer
Signed-off-by: Zion Orent <zorent@ics.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
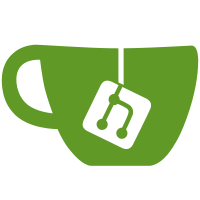
committed by
Mihai Tudor Panu
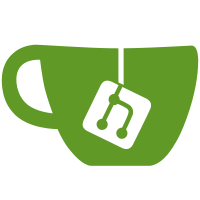
parent
60815f034d
commit
c95af93490
@ -47,30 +47,34 @@ setTimeout(function()
|
||||
g_myInterval = setInterval(runAccelerometer, 200);
|
||||
}, 2000);
|
||||
|
||||
var x = new analogGyro3Axis.intPointer(0);
|
||||
var y = new analogGyro3Axis.intPointer(0);
|
||||
var z = new analogGyro3Axis.intPointer(0);
|
||||
|
||||
var aX = new analogGyro3Axis.floatPointer(0);
|
||||
var aY = new analogGyro3Axis.floatPointer(0);
|
||||
var aZ = new analogGyro3Axis.floatPointer(0);
|
||||
var x = new analogGyro3Axis.new_intPointer();
|
||||
var y = new analogGyro3Axis.new_intPointer();
|
||||
var z = new analogGyro3Axis.new_intPointer();
|
||||
|
||||
var aX = new analogGyro3Axis.new_floatPointer();
|
||||
var aY = new analogGyro3Axis.new_floatPointer();
|
||||
var aZ = new analogGyro3Axis.new_floatPointer();
|
||||
|
||||
var outputStr;
|
||||
|
||||
function runAccelerometer()
|
||||
{
|
||||
|
||||
myAnalogGyro3Axis.values(x, y, z);
|
||||
outputStr = "Raw Values: X: " + x.getitem(0) +
|
||||
" Y: " + y.getitem(0) +
|
||||
" Z: " + z.getitem(0);
|
||||
outputStr = "Raw Values: X: " +
|
||||
analogGyro3Axis.intPointer_value(x) +
|
||||
" Y: " + analogGyro3Axis.intPointer_value(y) +
|
||||
" Z: " + analogGyro3Axis.intPointer_value(z);
|
||||
console.log(outputStr);
|
||||
|
||||
|
||||
myAnalogGyro3Axis.acceleration(aX, aY, aZ);
|
||||
console.log("Acceleration: X: " + aX.getitem(0) + "g");
|
||||
console.log("Acceleration: Y: " + aY.getitem(0) + "g");
|
||||
console.log("Acceleration: Z: " + aZ.getitem(0) + "g");
|
||||
outputStr = "Acceleration: X: " +
|
||||
analogGyro3Axis.floatPointer_value(aX) + "g\n" +
|
||||
"Acceleration: Y: " +
|
||||
analogGyro3Axis.floatPointer_value(aY) + "g\n" +
|
||||
"Acceleration: Z: " +
|
||||
analogGyro3Axis.floatPointer_value(aZ) + "g";
|
||||
console.log(outputStr);
|
||||
|
||||
console.log(" ");
|
||||
}
|
||||
|
78
examples/python/adxl335.py
Normal file
78
examples/python/adxl335.py
Normal file
@ -0,0 +1,78 @@
|
||||
#!/usr/bin/python
|
||||
# Author: Zion Orent <zorent@ics.com>
|
||||
# Copyright (c) 2015 Intel Corporation.
|
||||
#
|
||||
# Permission is hereby granted, free of charge, to any person obtaining
|
||||
# a copy of this software and associated documentation files (the
|
||||
# "Software"), to deal in the Software without restriction, including
|
||||
# without limitation the rights to use, copy, modify, merge, publish,
|
||||
# distribute, sublicense, and/or sell copies of the Software, and to
|
||||
# permit persons to whom the Software is furnished to do so, subject to
|
||||
# the following conditions:
|
||||
#
|
||||
# The above copyright notice and this permission notice shall be
|
||||
# included in all copies or substantial portions of the Software.
|
||||
#
|
||||
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
||||
import time, sys, signal, atexit
|
||||
import pyupm_adxl335 as upmAdxl335
|
||||
|
||||
myAnalogAccel = upmAdxl335.ADXL335(0, 1, 2)
|
||||
|
||||
print "Please make sure the sensor is completely still."
|
||||
print "Sleeping for 2 seconds"
|
||||
time.sleep(2)
|
||||
|
||||
|
||||
## Exit handlers ##
|
||||
# This stops python from printing a stacktrace when you hit control-C
|
||||
def SIGINTHandler(signum, frame):
|
||||
raise SystemExit
|
||||
|
||||
# This function lets you run code on exit,
|
||||
# including functions from myAnalogAccel
|
||||
def exitHandler():
|
||||
print "Exiting"
|
||||
sys.exit(0)
|
||||
|
||||
# Register exit handlers
|
||||
atexit.register(exitHandler)
|
||||
signal.signal(signal.SIGINT, SIGINTHandler)
|
||||
|
||||
|
||||
print "Calibrating..."
|
||||
myAnalogAccel.calibrate()
|
||||
|
||||
x = upmAdxl335.new_intPointer()
|
||||
y = upmAdxl335.new_intPointer()
|
||||
z = upmAdxl335.new_intPointer()
|
||||
|
||||
aX = upmAdxl335.new_floatPointer()
|
||||
aY = upmAdxl335.new_floatPointer()
|
||||
aZ = upmAdxl335.new_floatPointer()
|
||||
|
||||
while (1):
|
||||
myAnalogAccel.values(x, y, z)
|
||||
outputStr = "Raw Values: X: {0} Y: {1} Z: {2}".format(
|
||||
upmAdxl335.intPointer_value(x), upmAdxl335.intPointer_value(y),
|
||||
upmAdxl335.intPointer_value(z))
|
||||
print outputStr
|
||||
|
||||
myAnalogAccel.acceleration(aX, aY, aZ)
|
||||
outputStr = ("Acceleration: X: {0}g\n"
|
||||
"Acceleration: Y: {1}g\n"
|
||||
"Acceleration: Z: {2}g").format(upmAdxl335.floatPointer_value(aX),
|
||||
upmAdxl335.floatPointer_value(aY),
|
||||
upmAdxl335.floatPointer_value(aZ))
|
||||
print outputStr
|
||||
|
||||
print " "
|
||||
|
||||
time.sleep(.2)
|
@ -1,10 +1,9 @@
|
||||
%module jsupm_adxl335
|
||||
%include "../upm.i"
|
||||
%include "stdint.i"
|
||||
%include "carrays.i"
|
||||
%include "cpointer.i"
|
||||
|
||||
%array_class(int, intPointer);
|
||||
%array_class(float, floatPointer);
|
||||
%pointer_functions(int, intPointer);
|
||||
%pointer_functions(float, floatPointer);
|
||||
|
||||
%{
|
||||
#include "adxl335.h"
|
||||
|
@ -1,5 +1,9 @@
|
||||
%module pyupm_adxl335
|
||||
%include "../upm.i"
|
||||
%include "cpointer.i"
|
||||
|
||||
%pointer_functions(int, intPointer);
|
||||
%pointer_functions(float, floatPointer);
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
|
Reference in New Issue
Block a user