mirror of
https://github.com/eclipse/upm.git
synced 2025-07-19 02:01:15 +03:00
nlgpio16: Initial implementation
This is a USB device from Numato Labs that is accessed via a UART. It provides 16 GPIO's, 7 of which can be configured as analog inputs. Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
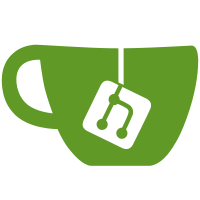
committed by
Mihai Tudor Panu
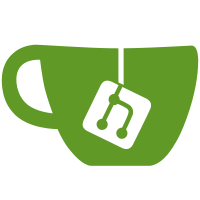
parent
4dc679af70
commit
d0cb2a032c
@ -14,7 +14,6 @@ macro(get_module_name example_name module_name)
|
|||||||
endif()
|
endif()
|
||||||
endmacro()
|
endmacro()
|
||||||
|
|
||||||
|
|
||||||
# Set source file, include and linker settings for an example
|
# Set source file, include and linker settings for an example
|
||||||
# If example cannot be built, example_bin is cleared
|
# If example cannot be built, example_bin is cleared
|
||||||
macro(add_custom_example example_bin example_src example_module_list)
|
macro(add_custom_example example_bin example_src example_module_list)
|
||||||
@ -231,6 +230,7 @@ if (OPENZWAVE_FOUND)
|
|||||||
include_directories(${OPENZWAVE_INCLUDE_DIRS})
|
include_directories(${OPENZWAVE_INCLUDE_DIRS})
|
||||||
add_example (ozw)
|
add_example (ozw)
|
||||||
endif()
|
endif()
|
||||||
|
add_example (nlgpio16)
|
||||||
|
|
||||||
# These are special cases where you specify example binary, source file and module(s)
|
# These are special cases where you specify example binary, source file and module(s)
|
||||||
include_directories (${PROJECT_SOURCE_DIR}/src)
|
include_directories (${PROJECT_SOURCE_DIR}/src)
|
||||||
|
52
examples/c++/nlgpio16.cxx
Normal file
52
examples/c++/nlgpio16.cxx
Normal file
@ -0,0 +1,52 @@
|
|||||||
|
/*
|
||||||
|
* Author: Jon Trulson <jtrulson@ics.com>
|
||||||
|
* Copyright (c) 2015 Intel Corporation.
|
||||||
|
*
|
||||||
|
* Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
* a copy of this software and associated documentation files (the
|
||||||
|
* "Software"), to deal in the Software without restriction, including
|
||||||
|
* without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
* permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
* the following conditions:
|
||||||
|
*
|
||||||
|
* The above copyright notice and this permission notice shall be
|
||||||
|
* included in all copies or substantial portions of the Software.
|
||||||
|
*
|
||||||
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
*/
|
||||||
|
|
||||||
|
#include <unistd.h>
|
||||||
|
#include <iostream>
|
||||||
|
#include <signal.h>
|
||||||
|
#include <stdio.h>
|
||||||
|
#include "nlgpio16.h"
|
||||||
|
|
||||||
|
using namespace std;
|
||||||
|
using namespace upm;
|
||||||
|
|
||||||
|
int main(int argc, char **argv)
|
||||||
|
{
|
||||||
|
// Instantiate a NLGPIO16 Module on the default UART (/dev/ttyACM0)
|
||||||
|
upm::NLGPIO16* sensor = new upm::NLGPIO16();
|
||||||
|
|
||||||
|
// get the Version
|
||||||
|
cout << "Device Version: " << sensor->getVersion() << endl;
|
||||||
|
// read the gpio at pin 3
|
||||||
|
cout << "GPIO 3 Value: " << sensor->gpioRead(3) << endl;
|
||||||
|
// read the analog voltage at pin 5
|
||||||
|
cout << "Analog 5 Voltage: " << sensor->analogReadVolts(5) << endl;
|
||||||
|
// set the gpio at pin 14 to HIGH
|
||||||
|
sensor->gpioSet(14);
|
||||||
|
|
||||||
|
delete sensor;
|
||||||
|
return 0;
|
||||||
|
}
|
||||||
|
|
||||||
|
//! [Interesting]
|
51
examples/javascript/nlgpio16.js
Normal file
51
examples/javascript/nlgpio16.js
Normal file
@ -0,0 +1,51 @@
|
|||||||
|
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||||
|
/*jshint unused:true */
|
||||||
|
|
||||||
|
/*
|
||||||
|
* Author: Jon Trulson <jtrulson@ics.com>
|
||||||
|
* Copyright (c) 2015 Intel Corporation.
|
||||||
|
*
|
||||||
|
* Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
* a copy of this software and associated documentation files (the
|
||||||
|
* "Software"), to deal in the Software without restriction, including
|
||||||
|
* without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
* permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
* the following conditions:
|
||||||
|
*
|
||||||
|
* The above copyright notice and this permission notice shall be
|
||||||
|
* included in all copies or substantial portions of the Software.
|
||||||
|
*
|
||||||
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
*/
|
||||||
|
|
||||||
|
|
||||||
|
var sensorObj = require('jsupm_nlgpio16');
|
||||||
|
|
||||||
|
// Instantiate a NLGPIO16 Module on the default UART (/dev/ttyACM0)
|
||||||
|
var sensor = new sensorObj.NLGPIO16();
|
||||||
|
|
||||||
|
// get the Version
|
||||||
|
console.log("Device Version:", sensor.getVersion());
|
||||||
|
// read the gpio at pin 3
|
||||||
|
console.log("GPIO 3 Value:", sensor.gpioRead(3));
|
||||||
|
// read the analog voltage at pin 5
|
||||||
|
console.log("Analog 5 Voltage:", sensor.analogReadVolts(5));
|
||||||
|
// set the gpio at pin 14 to HIGH
|
||||||
|
sensor.gpioSet(14);
|
||||||
|
|
||||||
|
/************** Exit code **************/
|
||||||
|
process.on('SIGINT', function()
|
||||||
|
{
|
||||||
|
sensor = null;
|
||||||
|
sensorObj.cleanUp();
|
||||||
|
sensorObj = null;
|
||||||
|
console.log("Exiting...");
|
||||||
|
process.exit(0);
|
||||||
|
});
|
51
examples/python/nlgpio16.py
Normal file
51
examples/python/nlgpio16.py
Normal file
@ -0,0 +1,51 @@
|
|||||||
|
#!/usr/bin/python
|
||||||
|
# Author: Jon Trulson <jtrulson@ics.com>
|
||||||
|
# Copyright (c) 2015 Intel Corporation.
|
||||||
|
#
|
||||||
|
# Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
# a copy of this software and associated documentation files (the
|
||||||
|
# "Software"), to deal in the Software without restriction, including
|
||||||
|
# without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
# distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
# permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
# the following conditions:
|
||||||
|
#
|
||||||
|
# The above copyright notice and this permission notice shall be
|
||||||
|
# included in all copies or substantial portions of the Software.
|
||||||
|
#
|
||||||
|
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
|
||||||
|
import sys, signal, atexit
|
||||||
|
import pyupm_nlgpio16 as sensorObj
|
||||||
|
|
||||||
|
# Instantiate a NLGPIO16 Module on the default UART (/dev/ttyACM0)
|
||||||
|
sensor = sensorObj.NLGPIO16()
|
||||||
|
|
||||||
|
## Exit handlers ##
|
||||||
|
# This stops python from printing a stacktrace when you hit control-C
|
||||||
|
def SIGINTHandler(signum, frame):
|
||||||
|
raise SystemExit
|
||||||
|
|
||||||
|
# This function lets you run code on exit
|
||||||
|
def exitHandler():
|
||||||
|
print "Exiting"
|
||||||
|
sys.exit(0)
|
||||||
|
|
||||||
|
# Register exit handlers
|
||||||
|
atexit.register(exitHandler)
|
||||||
|
signal.signal(signal.SIGINT, SIGINTHandler)
|
||||||
|
|
||||||
|
# get the Version
|
||||||
|
print "Device Version:", sensor.getVersion()
|
||||||
|
# read the gpio at pin 3
|
||||||
|
print "GPIO 3 Value:", sensor.gpioRead(3)
|
||||||
|
# read the analog voltage at pin 5
|
||||||
|
print "Analog 5 Voltage:", sensor.analogReadVolts(5)
|
||||||
|
# set the gpio at pin 14 to HIGH
|
||||||
|
sensor.gpioSet(14)
|
5
src/nlgpio16/CMakeLists.txt
Normal file
5
src/nlgpio16/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
|||||||
|
set (libname "nlgpio16")
|
||||||
|
set (libdescription "upm module for the Numato Labs GPIO 16 USB")
|
||||||
|
set (module_src ${libname}.cxx)
|
||||||
|
set (module_h ${libname}.h)
|
||||||
|
upm_module_init()
|
22
src/nlgpio16/javaupm_nlgpio16.i
Normal file
22
src/nlgpio16/javaupm_nlgpio16.i
Normal file
@ -0,0 +1,22 @@
|
|||||||
|
%module javaupm_nlgpio16
|
||||||
|
%include "../upm.i"
|
||||||
|
%include "carrays.i"
|
||||||
|
%include "std_string.i"
|
||||||
|
|
||||||
|
%{
|
||||||
|
#include "nlgpio16.h"
|
||||||
|
%}
|
||||||
|
|
||||||
|
%include "nlgpio16.h"
|
||||||
|
%array_class(char, charArray);
|
||||||
|
|
||||||
|
%pragma(java) jniclasscode=%{
|
||||||
|
static {
|
||||||
|
try {
|
||||||
|
System.loadLibrary("javaupm_nlgpio16");
|
||||||
|
} catch (UnsatisfiedLinkError e) {
|
||||||
|
System.err.println("Native code library failed to load. \n" + e);
|
||||||
|
System.exit(1);
|
||||||
|
}
|
||||||
|
}
|
||||||
|
%}
|
11
src/nlgpio16/jsupm_nlgpio16.i
Normal file
11
src/nlgpio16/jsupm_nlgpio16.i
Normal file
@ -0,0 +1,11 @@
|
|||||||
|
%module jsupm_nlgpio16
|
||||||
|
%include "../upm.i"
|
||||||
|
%include "carrays.i"
|
||||||
|
%include "std_string.i"
|
||||||
|
|
||||||
|
%{
|
||||||
|
#include "nlgpio16.h"
|
||||||
|
%}
|
||||||
|
|
||||||
|
%include "nlgpio16.h"
|
||||||
|
%array_class(char, charArray);
|
344
src/nlgpio16/nlgpio16.cxx
Normal file
344
src/nlgpio16/nlgpio16.cxx
Normal file
@ -0,0 +1,344 @@
|
|||||||
|
/*
|
||||||
|
* Author: Jon Trulson <jtrulson@ics.com>
|
||||||
|
* Copyright (c) 2015 Intel Corporation.
|
||||||
|
*
|
||||||
|
* Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
* a copy of this software and associated documentation files (the
|
||||||
|
* "Software"), to deal in the Software without restriction, including
|
||||||
|
* without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
* permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
* the following conditions:
|
||||||
|
*
|
||||||
|
* The above copyright notice and this permission notice shall be
|
||||||
|
* included in all copies or substantial portions of the Software.
|
||||||
|
*
|
||||||
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
*/
|
||||||
|
|
||||||
|
#include <iostream>
|
||||||
|
#include <time.h>
|
||||||
|
|
||||||
|
#include "nlgpio16.h"
|
||||||
|
|
||||||
|
using namespace upm;
|
||||||
|
using namespace std;
|
||||||
|
|
||||||
|
static const int maxBuffer = 1024;
|
||||||
|
static const int baudRate = 115200;
|
||||||
|
|
||||||
|
static char num2Hex(int i, bool upcase)
|
||||||
|
{
|
||||||
|
char temp;
|
||||||
|
|
||||||
|
switch (i)
|
||||||
|
{
|
||||||
|
case 0: return '0';
|
||||||
|
case 1: return '1';
|
||||||
|
case 2: return '2';
|
||||||
|
case 3: return '3';
|
||||||
|
case 4: return '4';
|
||||||
|
case 5: return '5';
|
||||||
|
case 6: return '6';
|
||||||
|
case 7: return '7';
|
||||||
|
case 8: return '8';
|
||||||
|
case 9: return '9';
|
||||||
|
case 10: temp = (upcase) ? 'A' : 'a'; return temp;
|
||||||
|
case 11: temp = (upcase) ? 'B' : 'b'; return temp;
|
||||||
|
case 12: temp = (upcase) ? 'C' : 'c'; return temp;
|
||||||
|
case 13: temp = (upcase) ? 'D' : 'd'; return temp;
|
||||||
|
case 14: temp = (upcase) ? 'E' : 'e'; return temp;
|
||||||
|
case 15: temp = (upcase) ? 'F' : 'f'; return temp;
|
||||||
|
|
||||||
|
default:
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": number must be between 0 and 15");
|
||||||
|
return '0';
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
NLGPIO16::NLGPIO16(string uart) :
|
||||||
|
m_uart(uart)
|
||||||
|
{
|
||||||
|
m_uart.setBaudRate(baudRate);
|
||||||
|
}
|
||||||
|
|
||||||
|
NLGPIO16::~NLGPIO16()
|
||||||
|
{
|
||||||
|
}
|
||||||
|
|
||||||
|
bool NLGPIO16::dataAvailable(unsigned int millis)
|
||||||
|
{
|
||||||
|
return m_uart.dataAvailable(millis);
|
||||||
|
}
|
||||||
|
|
||||||
|
std::string NLGPIO16::readStr(int len)
|
||||||
|
{
|
||||||
|
return m_uart.readStr(len);
|
||||||
|
}
|
||||||
|
|
||||||
|
int NLGPIO16::writeStr(std::string data)
|
||||||
|
{
|
||||||
|
m_uart.flush();
|
||||||
|
return m_uart.writeStr(data);
|
||||||
|
}
|
||||||
|
|
||||||
|
string NLGPIO16::sendCommand(string cmd)
|
||||||
|
{
|
||||||
|
// make sure we got a command
|
||||||
|
if (cmd.empty())
|
||||||
|
{
|
||||||
|
throw std::invalid_argument(std::string(__FUNCTION__) +
|
||||||
|
": cmd is empty!");
|
||||||
|
return "";
|
||||||
|
}
|
||||||
|
|
||||||
|
// make sure string is CR terminated
|
||||||
|
if (cmd.at(cmd.size() - 1) != '\r')
|
||||||
|
cmd.append("\r");
|
||||||
|
|
||||||
|
writeStr(cmd);
|
||||||
|
|
||||||
|
string resp;
|
||||||
|
while (dataAvailable(10))
|
||||||
|
{
|
||||||
|
resp += readStr(maxBuffer);
|
||||||
|
}
|
||||||
|
|
||||||
|
if (resp.empty())
|
||||||
|
{
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
": timed out waiting for response");
|
||||||
|
return "";
|
||||||
|
}
|
||||||
|
|
||||||
|
// check that the last character is the prompt
|
||||||
|
if (resp.at(resp.size() - 1) != '>')
|
||||||
|
{
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
": read from device corrupted");
|
||||||
|
return "";
|
||||||
|
}
|
||||||
|
|
||||||
|
// delete the last 3 characters, which should be '\n\r>'
|
||||||
|
resp.erase(resp.size() - 3, 3);
|
||||||
|
|
||||||
|
// find first delimeter (second should already be erased)
|
||||||
|
size_t pos = resp.find("\n\r");
|
||||||
|
|
||||||
|
// if we didn't find one, then the command was invalid. This
|
||||||
|
// shouldn't happen, but we check for it anyway
|
||||||
|
if (pos == string::npos)
|
||||||
|
return "";
|
||||||
|
|
||||||
|
pos += 2; // skip past them.
|
||||||
|
|
||||||
|
// now we should have the value (or an empty string in the case of a
|
||||||
|
// write command). Erase everything else and return the result.
|
||||||
|
resp.erase(0, pos);
|
||||||
|
|
||||||
|
return resp;
|
||||||
|
}
|
||||||
|
|
||||||
|
void NLGPIO16::gpioSet(int gpio)
|
||||||
|
{
|
||||||
|
if (gpio < 0 || gpio > 15)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": gpio must be between 0 and 15");
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("gpio set ");
|
||||||
|
cmd += num2Hex(gpio, true);
|
||||||
|
|
||||||
|
// set commands don't return anything useful
|
||||||
|
sendCommand(cmd);
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
void NLGPIO16::gpioClear(int gpio)
|
||||||
|
{
|
||||||
|
if (gpio < 0 || gpio > 15)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": gpio must be between 0 and 15");
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("gpio clear ");
|
||||||
|
cmd += num2Hex(gpio, true);
|
||||||
|
|
||||||
|
// set commands don't return anything useful
|
||||||
|
sendCommand(cmd);
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
bool NLGPIO16::gpioRead(int gpio)
|
||||||
|
{
|
||||||
|
if (gpio < 0 || gpio > 15)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": gpio must be between 0 and 15");
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("gpio read ");
|
||||||
|
cmd += num2Hex(gpio, true);
|
||||||
|
|
||||||
|
string resp = sendCommand(cmd);
|
||||||
|
if (resp.empty())
|
||||||
|
{
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
": invalid empty response from device");
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
|
||||||
|
// c++11 toString() would be handy...
|
||||||
|
return ( (atoi(resp.c_str()) == 0) ? false : true );
|
||||||
|
}
|
||||||
|
|
||||||
|
unsigned int NLGPIO16::gpioReadAll()
|
||||||
|
{
|
||||||
|
string cmd("gpio readall");
|
||||||
|
|
||||||
|
string resp = sendCommand(cmd);
|
||||||
|
if (resp.empty())
|
||||||
|
{
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
": invalid empty response from device");
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
|
||||||
|
// Convert to unsigned int (but mask out everything but the lower 16bits)
|
||||||
|
unsigned int value = strtoul(resp.c_str(), NULL, 16);
|
||||||
|
value &= 0xffff;
|
||||||
|
|
||||||
|
return value;
|
||||||
|
}
|
||||||
|
|
||||||
|
void NLGPIO16::gpioSetIOMask(int mask)
|
||||||
|
{
|
||||||
|
if (mask < 0 || mask > 65535)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": mask must be between 0 and 65535");
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("gpio iomask ");
|
||||||
|
cmd += num2Hex( ((mask & 0xf000) >> 12), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x0f00) >> 8), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x00f0) >> 4), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x000f) >> 0), false);
|
||||||
|
|
||||||
|
sendCommand(cmd);
|
||||||
|
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
void NLGPIO16::gpioSetIODir(int mask)
|
||||||
|
{
|
||||||
|
if (mask < 0 || mask > 65535)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": mask must be between 0 and 65535");
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("gpio iodir ");
|
||||||
|
cmd += num2Hex( ((mask & 0xf000) >> 12), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x0f00) >> 8), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x00f0) >> 4), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x000f) >> 0), false);
|
||||||
|
|
||||||
|
sendCommand(cmd);
|
||||||
|
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
void NLGPIO16::gpioWriteAll(int mask)
|
||||||
|
{
|
||||||
|
if (mask < 0 || mask > 65535)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": mask must be between 0 and 65535");
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("gpio writeall ");
|
||||||
|
cmd += num2Hex( ((mask & 0xf000) >> 12), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x0f00) >> 8), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x00f0) >> 4), false);
|
||||||
|
cmd += num2Hex( ((mask & 0x000f) >> 0), false);
|
||||||
|
|
||||||
|
sendCommand(cmd);
|
||||||
|
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
string NLGPIO16::getVersion()
|
||||||
|
{
|
||||||
|
return sendCommand("vers");
|
||||||
|
}
|
||||||
|
|
||||||
|
string NLGPIO16::getID()
|
||||||
|
{
|
||||||
|
return sendCommand("id get");
|
||||||
|
}
|
||||||
|
|
||||||
|
void NLGPIO16::setID(string id)
|
||||||
|
{
|
||||||
|
if (id.size() != 8)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": ID must be exactly 8 characters in length");
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("id set ");
|
||||||
|
cmd += id;
|
||||||
|
|
||||||
|
sendCommand(cmd);
|
||||||
|
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
int NLGPIO16::analogReadValue(int adc)
|
||||||
|
{
|
||||||
|
// Only ports 0-6 are ADC capable
|
||||||
|
if (adc < 0 || adc > 6)
|
||||||
|
{
|
||||||
|
throw std::out_of_range(std::string(__FUNCTION__) +
|
||||||
|
": adc must be between 0 and 6");
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
|
||||||
|
string cmd("adc read ");
|
||||||
|
cmd += num2Hex(adc, true);
|
||||||
|
|
||||||
|
string resp = sendCommand(cmd);
|
||||||
|
if (resp.empty())
|
||||||
|
{
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
": invalid empty response from device");
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
|
||||||
|
// c++11 stoi() would be handy...
|
||||||
|
return atoi(resp.c_str());
|
||||||
|
}
|
||||||
|
|
||||||
|
float NLGPIO16::analogReadVolts(int adc)
|
||||||
|
{
|
||||||
|
float value = float(analogReadValue(adc));
|
||||||
|
|
||||||
|
return ( value * (ADC_AREF / float(1 << ADC_PRECISION)) );
|
||||||
|
}
|
||||||
|
|
238
src/nlgpio16/nlgpio16.h
Normal file
238
src/nlgpio16/nlgpio16.h
Normal file
@ -0,0 +1,238 @@
|
|||||||
|
/*
|
||||||
|
* Author: Jon Trulson <jtrulson@ics.com>
|
||||||
|
* Copyright (c) 2015 Intel Corporation.
|
||||||
|
*
|
||||||
|
* Thanks to Adafruit for supplying a google translated version of the
|
||||||
|
* Chinese datasheet and some clues in their code.
|
||||||
|
*
|
||||||
|
* Permission is hereby granted, free of charge, to any person obtaining
|
||||||
|
* a copy of this software and associated documentation files (the
|
||||||
|
* "Software"), to deal in the Software without restriction, including
|
||||||
|
* without limitation the rights to use, copy, modify, merge, publish,
|
||||||
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||||
|
* permit persons to whom the Software is furnished to do so, subject to
|
||||||
|
* the following conditions:
|
||||||
|
*
|
||||||
|
* The above copyright notice and this permission notice shall be
|
||||||
|
* included in all copies or substantial portions of the Software.
|
||||||
|
*
|
||||||
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||||
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||||
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||||
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||||
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||||
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||||
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||||
|
*/
|
||||||
|
#pragma once
|
||||||
|
|
||||||
|
#include <string>
|
||||||
|
#include <iostream>
|
||||||
|
|
||||||
|
#include <stdlib.h>
|
||||||
|
#include <unistd.h>
|
||||||
|
#include <string.h>
|
||||||
|
|
||||||
|
#include <mraa/common.hpp>
|
||||||
|
#include <mraa/uart.hpp>
|
||||||
|
|
||||||
|
#define NLGPIO16_DEFAULT_UART_DEV "/dev/ttyACM0"
|
||||||
|
|
||||||
|
namespace upm {
|
||||||
|
/**
|
||||||
|
* @brief NLGPIO16 module
|
||||||
|
* @defgroup nlgpio16 libupm-nlgpio16
|
||||||
|
* @ingroup sparkfun uart gpio ainput
|
||||||
|
*/
|
||||||
|
|
||||||
|
/**
|
||||||
|
* @library nlgpio16
|
||||||
|
* @sensor nlgpio16
|
||||||
|
* @comname NLGPIO16 16 channel USB GPIO Module
|
||||||
|
* @type other
|
||||||
|
* @man numatolabs
|
||||||
|
* @con uart
|
||||||
|
* @web http://numato.com/16-channel-usb-gpio-module-with-analog-inputs/
|
||||||
|
*
|
||||||
|
* @brief API for the NLGPIO16 16 channel USB GPIO Module
|
||||||
|
*
|
||||||
|
* The NLGPIO16 is a USB adapter providing access to 16 GPIO's, 7
|
||||||
|
* of which can be used as analog inputs. The GPIO's are 3.3v
|
||||||
|
* only. An external power supply can be connected to provide
|
||||||
|
* more current if the need arises.
|
||||||
|
*
|
||||||
|
* It is recommended to use a series resistor with the GPIO/ADC
|
||||||
|
* pins when interfacing with other circuits. In output mode,
|
||||||
|
* GPIOs can source up to 8mA (gpio8-gpio15). So no additional
|
||||||
|
* circuitry is needed to drive regular LEDs. A 470 Ohms series
|
||||||
|
* resistor is recommended for current limiting when connecting an
|
||||||
|
* LED to a GPIO. In contrast to GPIOs, analog inputs can read
|
||||||
|
* voltages at any level between 0 to 3.3V volts. It is
|
||||||
|
* recommended to use a series resistor to protect the input from
|
||||||
|
* stray voltages and spikes. The internal Analog to Digital
|
||||||
|
* converter supports 10 bits resolution which is adequate for
|
||||||
|
* most applications.
|
||||||
|
*
|
||||||
|
* Maximum IO source/sink current on GPIO 0-7 is 2mA
|
||||||
|
* Maximum IO source/sink current on GPIO 8-15 is 8mA
|
||||||
|
*
|
||||||
|
* @snippet nlgpio16.cxx Interesting
|
||||||
|
*/
|
||||||
|
|
||||||
|
class NLGPIO16 {
|
||||||
|
public:
|
||||||
|
|
||||||
|
// ADC analog ref voltage is 3.3v
|
||||||
|
static const float ADC_AREF = 3.3;
|
||||||
|
// 10-bit precision (0-1023)
|
||||||
|
static const int ADC_PRECISION = 10;
|
||||||
|
|
||||||
|
/**
|
||||||
|
* NLGPIO16 object constructor
|
||||||
|
*
|
||||||
|
* @param uart UART device path to use. Default is /dev/ttyACM0.
|
||||||
|
*/
|
||||||
|
NLGPIO16(std::string uart=NLGPIO16_DEFAULT_UART_DEV);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* NLGPIO16 object destructor
|
||||||
|
*/
|
||||||
|
~NLGPIO16();
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Get the version of the device
|
||||||
|
*
|
||||||
|
* @return String containing device revision
|
||||||
|
*/
|
||||||
|
std::string getVersion();
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Get the device ID. The device ID is an 8 character
|
||||||
|
* alpha-numeric string.
|
||||||
|
*
|
||||||
|
* @return String containing device ID
|
||||||
|
*/
|
||||||
|
std::string getID();
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Set the device ID. The device ID is an 8 character
|
||||||
|
* alpha-numeric string. The supplied ID must be exactly 8
|
||||||
|
* characters in length.
|
||||||
|
*
|
||||||
|
* @param id String containing a new 8 character device ID
|
||||||
|
*/
|
||||||
|
void setID(std::string id);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Set a gpio output to the HIGH (1) state. The gpio is
|
||||||
|
* automatically set as an output when this call is made,
|
||||||
|
* regardless of it's previous mode.
|
||||||
|
*
|
||||||
|
* @param gpio The gpio to set. Valid values are between 0-15
|
||||||
|
*/
|
||||||
|
void gpioSet(int gpio);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Set a gpio output to the LOW (0) state. The gpio is
|
||||||
|
* automatically set as an output when this call is made,
|
||||||
|
* regardless of it's previous mode.
|
||||||
|
*
|
||||||
|
* @param gpio The gpio to clear. Valid values are between 0-15
|
||||||
|
*/
|
||||||
|
void gpioClear(int gpio);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Read the state of a gpio. The gpio is automatically set as an
|
||||||
|
* input when this call is made, regardless of it's previous
|
||||||
|
* mode.
|
||||||
|
*
|
||||||
|
* @param gpio The gpio to read. Valid values are between 0-15
|
||||||
|
* @return true if the gpio is in the HIGH state, false otherwise
|
||||||
|
*/
|
||||||
|
bool gpioRead(int gpio);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Read the state of all gpios. The returned integer is a bitmask
|
||||||
|
* of all 16 gpios, where a 0 bit means the gpio is in the LOW
|
||||||
|
* state, whereas a 1 bit means the gpio is in a HIGH state.
|
||||||
|
*
|
||||||
|
* @return bitmask of the state of all 16 gpios. The LSB is gpio0.
|
||||||
|
*/
|
||||||
|
unsigned int gpioReadAll();
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Set a mask for selectively updating multiple gpios with the
|
||||||
|
* gpioIODir() and gpioWriteAll() methods. Each bit set in the 16
|
||||||
|
* bit argument (LSB = gpio0) represents whether the two previously
|
||||||
|
* mentioned methods will act on a given gpio or not. A 0 in a
|
||||||
|
* given bit position will cause any update to that gpio via
|
||||||
|
* gpioIODir() and gpioWriteAll() to be ignored, while a 1 bit
|
||||||
|
* enables that gpio to be affected by those two methods.
|
||||||
|
*
|
||||||
|
* @param mask A bitmask of the 16 gpios affected by gpioIODir() and
|
||||||
|
* gpioWriteAll()
|
||||||
|
*/
|
||||||
|
void gpioSetIOMask(int mask);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Set the driection mode (input or output) for all gpios enabled
|
||||||
|
* by gpioSetIOMask(). A 0 in a given bit postion (LSB = gpio0)
|
||||||
|
* configures the gpio as an ouput, and a 1 bit configures the
|
||||||
|
* gpio as an input. Only the gpios enabled by gpioSetMask() are
|
||||||
|
* affected by this call.
|
||||||
|
*
|
||||||
|
* @param mask A bitmask of the 16 gpios whose direction mode is
|
||||||
|
* to be set
|
||||||
|
*/
|
||||||
|
void gpioSetIODir(int mask);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Write all enabled gpios (set via gpioSetIOMask()) to a given
|
||||||
|
* value. A 1 bit (LSB = gpio0) sets the given output to HIGH, a
|
||||||
|
* zero sets the given output to LOW. Only the gpios enabled by
|
||||||
|
* gpioSetMask() are affected by this call.
|
||||||
|
*
|
||||||
|
* @param mask The values to set for the 16 gpios (LSB = gpio0)
|
||||||
|
*/
|
||||||
|
void gpioWriteAll(int mask);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Read the raw analog input value present at the given gpio. The
|
||||||
|
* gpio is switched to analog input mode by this call, regardless of
|
||||||
|
* any previous mode. The returned value will be a number between
|
||||||
|
* 0-1023 (10 bit resolution). Only the first 7 gpios (0-6) can be
|
||||||
|
* used for analog input.
|
||||||
|
*
|
||||||
|
* @param adc The gpio number to read (0-6)
|
||||||
|
* @return The raw integer value from the ADC (0-1023)
|
||||||
|
*/
|
||||||
|
int analogReadValue(int adc);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Read the raw analog input value present at the given gpio and
|
||||||
|
* return the coresponding voltage value at the pin. The gpio is
|
||||||
|
* switched to analog input mode by this call, regardless of any
|
||||||
|
* previous mode. The returned value will be a number between
|
||||||
|
* 0.0-3.3, depending on the voltage present at the pin. Only the
|
||||||
|
* first 7 gpios (0-6) can be used for analog input.
|
||||||
|
*
|
||||||
|
* @param adc The gpio number to read (0-6)
|
||||||
|
* @return The voltage present at the pin
|
||||||
|
*/
|
||||||
|
float analogReadVolts(int adc);
|
||||||
|
|
||||||
|
|
||||||
|
protected:
|
||||||
|
mraa::Uart m_uart;
|
||||||
|
|
||||||
|
// UART helpers
|
||||||
|
bool dataAvailable(unsigned int millis);
|
||||||
|
std::string readStr(int len);
|
||||||
|
int writeStr(std::string data);
|
||||||
|
|
||||||
|
// does most of the work for sending commands and getting responses
|
||||||
|
std::string sendCommand(std::string cmd);
|
||||||
|
|
||||||
|
private:
|
||||||
|
};
|
||||||
|
}
|
12
src/nlgpio16/pyupm_nlgpio16.i
Normal file
12
src/nlgpio16/pyupm_nlgpio16.i
Normal file
@ -0,0 +1,12 @@
|
|||||||
|
%module pyupm_nlgpio16
|
||||||
|
%include "../upm.i"
|
||||||
|
%include "carrays.i"
|
||||||
|
%include "std_string.i"
|
||||||
|
|
||||||
|
%feature("autodoc", "3");
|
||||||
|
|
||||||
|
%{
|
||||||
|
#include "nlgpio16.h"
|
||||||
|
%}
|
||||||
|
%include "nlgpio16.h"
|
||||||
|
%array_class(char, charArray);
|
@ -314,6 +314,12 @@
|
|||||||
* @ingroup byman
|
* @ingroup byman
|
||||||
*/
|
*/
|
||||||
|
|
||||||
|
/**
|
||||||
|
* @brief Numato Labs
|
||||||
|
* @defgroup numatolabs Numato Labs
|
||||||
|
* @ingroup byman
|
||||||
|
*/
|
||||||
|
|
||||||
/**
|
/**
|
||||||
* @brief SeeedStudio - Grove Sensors
|
* @brief SeeedStudio - Grove Sensors
|
||||||
* @defgroup seeed SeeedStudio
|
* @defgroup seeed SeeedStudio
|
||||||
|
Reference in New Issue
Block a user