mirror of
https://github.com/eclipse/upm.git
synced 2025-07-13 07:11:14 +03:00
BH1792: Add string based constructor
Signed-off-by: Adelin Dobre <adelin.dobre@rinftech.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
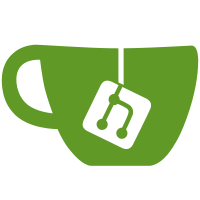
committed by
Mihai Tudor Panu
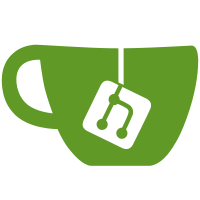
parent
bd445385a7
commit
d2428ca5da
@ -25,6 +25,7 @@
|
|||||||
#include <iostream>
|
#include <iostream>
|
||||||
#include <stdexcept>
|
#include <stdexcept>
|
||||||
#include "bh1792.hpp"
|
#include "bh1792.hpp"
|
||||||
|
#include "upm_string_parser.hpp"
|
||||||
|
|
||||||
using namespace upm;
|
using namespace upm;
|
||||||
|
|
||||||
@ -35,6 +36,85 @@ BH1792::BH1792(int bus, int addr) : m_bh1792(bh1792_init(bus, addr))
|
|||||||
"bh1792_init() failed");
|
"bh1792_init() failed");
|
||||||
}
|
}
|
||||||
|
|
||||||
|
BH1792::BH1792(std::string initStr) : mraaIo(initStr)
|
||||||
|
{
|
||||||
|
mraa_io_descriptor* descs = mraaIo.getMraaDescriptors();
|
||||||
|
std::vector<std::string> upmTokens;
|
||||||
|
|
||||||
|
if(!mraaIo.getLeftoverStr().empty()) {
|
||||||
|
upmTokens = UpmStringParser::parse(mraaIo.getLeftoverStr());
|
||||||
|
}
|
||||||
|
|
||||||
|
m_bh1792 = (bh1792_context)malloc(sizeof(struct _bh1792_context));
|
||||||
|
if(!m_bh1792) {
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
"bh1792_init() failed");
|
||||||
|
}
|
||||||
|
|
||||||
|
m_bh1792->i2c = NULL;
|
||||||
|
m_bh1792->interrupt = NULL;
|
||||||
|
|
||||||
|
if(mraa_init() != MRAA_SUCCESS) {
|
||||||
|
bh1792_close(m_bh1792);
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
"bh1792_init() failed");
|
||||||
|
}
|
||||||
|
|
||||||
|
if(!descs->i2cs) {
|
||||||
|
bh1792_close(m_bh1792);
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
": mraa_i2c_init() failed");
|
||||||
|
} else {
|
||||||
|
if( !(m_bh1792->i2c = descs->i2cs[0]) ) {
|
||||||
|
bh1792_close(m_bh1792);
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
": mraa_i2c_init() failed");
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
if(bh1792_check_who_am_i(m_bh1792) != UPM_SUCCESS)
|
||||||
|
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||||
|
"bh1792_init() failed");
|
||||||
|
|
||||||
|
m_bh1792->enabled = false;
|
||||||
|
m_bh1792->isrEnabled = false;
|
||||||
|
m_bh1792->sync_thread_alive = false;
|
||||||
|
|
||||||
|
std::string::size_type sz;
|
||||||
|
for(std::string tok : upmTokens) {
|
||||||
|
if(tok.substr(0, 20) == "SetGreenLedsCurrent:") {
|
||||||
|
uint16_t current = std::stoul(tok.substr(20), &sz, 0);
|
||||||
|
SetGreenLedsCurrent(current);
|
||||||
|
}
|
||||||
|
if(tok.substr(0, 16) == "SetIrLedCurrent:") {
|
||||||
|
uint16_t current = std::stoul(tok.substr(16), &sz, 0);
|
||||||
|
SetIrLedCurrent(current);
|
||||||
|
}
|
||||||
|
if(tok.substr(0, 15) == "SetIrThreshold:") {
|
||||||
|
uint16_t current = std::stoul(tok.substr(15), &sz, 0);
|
||||||
|
SetIrThreshold(current);
|
||||||
|
}
|
||||||
|
if(tok.substr(0, 15) == "EnableSyncMode:") {
|
||||||
|
uint16_t measFreq = std::stoul(tok.substr(15), &sz, 0);
|
||||||
|
tok = tok.substr(15);
|
||||||
|
uint16_t green_current = std::stoul(tok.substr(sz+1), nullptr, 0);
|
||||||
|
EnableSyncMode(measFreq, green_current);
|
||||||
|
}
|
||||||
|
if(tok.substr(0, 18) == "EnableNonSyncMode:") {
|
||||||
|
uint16_t ir_current = std::stoul(tok.substr(18), &sz, 0);
|
||||||
|
tok = tok.substr(18);
|
||||||
|
uint16_t threshold = std::stoul(tok.substr(sz+1), nullptr, 0);
|
||||||
|
EnableNonSyncMode(ir_current, threshold);
|
||||||
|
}
|
||||||
|
if(tok.substr(0, 17) == "EnableSingleMode:") {
|
||||||
|
LED_TYPES led_type = (LED_TYPES)std::stoi(tok.substr(17), &sz, 0);
|
||||||
|
tok = tok.substr(17);
|
||||||
|
uint16_t current = std::stoul(tok.substr(sz+1), nullptr, 0);
|
||||||
|
EnableSingleMode(led_type, current);
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
BH1792::~BH1792()
|
BH1792::~BH1792()
|
||||||
{
|
{
|
||||||
bh1792_close(m_bh1792);
|
bh1792_close(m_bh1792);
|
||||||
|
@ -27,6 +27,7 @@
|
|||||||
#include <vector>
|
#include <vector>
|
||||||
#include <string>
|
#include <string>
|
||||||
#include "bh1792.h"
|
#include "bh1792.h"
|
||||||
|
#include <mraa/initio.hpp>
|
||||||
|
|
||||||
/**
|
/**
|
||||||
* @brief BH1792 Heart Rate Sensor
|
* @brief BH1792 Heart Rate Sensor
|
||||||
@ -61,6 +62,14 @@ namespace upm {
|
|||||||
*/
|
*/
|
||||||
BH1792(int bus = 0, int addr = 0x5b);
|
BH1792(int bus = 0, int addr = 0x5b);
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Instantiates BH1792 Heart Rate Sensor based on a given string.
|
||||||
|
*
|
||||||
|
* @param initStr string containing specific information for BH1792 initialization.
|
||||||
|
*/
|
||||||
|
BH1792(std::string initStr);
|
||||||
|
|
||||||
|
|
||||||
/**
|
/**
|
||||||
* @brief Close and free sensor
|
* @brief Close and free sensor
|
||||||
*/
|
*/
|
||||||
@ -316,6 +325,7 @@ namespace upm {
|
|||||||
|
|
||||||
private:
|
private:
|
||||||
bh1792_context m_bh1792;
|
bh1792_context m_bh1792;
|
||||||
|
mraa::MraaIo mraaIo;
|
||||||
|
|
||||||
/* Disable implicit copy and assignment operators */
|
/* Disable implicit copy and assignment operators */
|
||||||
BH1792(const BH1792 &) = delete;
|
BH1792(const BH1792 &) = delete;
|
||||||
|
Reference in New Issue
Block a user