mirror of
https://github.com/eclipse/upm.git
synced 2025-07-11 22:31:14 +03:00
groveehr: Initial implementation
This module implements support for the Grove Ear-clip heart rate sensor. Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Zion Orent <zorent@ics.com> Signed-off-by: Sarah Knepper <sarah.knepper@intel.com>
This commit is contained in:
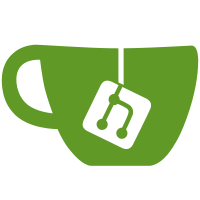
committed by
Sarah Knepper
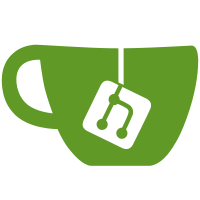
parent
6e8b0dd038
commit
ed15b1042d
@ -58,6 +58,7 @@ add_executable (ds1307-example ds1307.cxx)
|
||||
add_executable (a110x-example a110x.cxx)
|
||||
add_executable (gp2y0a21yk-example gp2y0a21yk.cxx)
|
||||
add_executable (grovemoisture-example grovemoisture.cxx)
|
||||
add_executable (groveehr-example groveehr.cxx)
|
||||
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/hmc5883l)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grove)
|
||||
@ -103,6 +104,7 @@ include_directories (${PROJECT_SOURCE_DIR}/src/ds1307)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/a110x)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/gp2y0a21yk)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grovemoisture)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/groveehr)
|
||||
|
||||
target_link_libraries (hmc5883l-example hmc5883l ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (groveled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||
@ -164,3 +166,4 @@ target_link_libraries (ds1307-example ds1307 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (a110x-example a110x ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (gp2y0a21yk-example gp2y0a21yk ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (grovemoisture-example grovemoisture ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (groveehr-example groveehr ${CMAKE_THREAD_LIBS_INIT})
|
||||
|
78
examples/groveehr.cxx
Normal file
78
examples/groveehr.cxx
Normal file
@ -0,0 +1,78 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
#include "groveehr.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
int shouldRun = true;
|
||||
|
||||
void sig_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
shouldRun = false;
|
||||
}
|
||||
|
||||
|
||||
int main()
|
||||
{
|
||||
signal(SIGINT, sig_handler);
|
||||
|
||||
//! [Interesting]
|
||||
// Instantiate a Grove Ear-clip Heart Rate sensor on digital pin D2
|
||||
upm::GroveEHR* heart = new upm::GroveEHR(2);
|
||||
|
||||
// set the beat counter to 0, init the clock and start counting beats
|
||||
heart->clearBeatCounter();
|
||||
heart->initClock();
|
||||
heart->startBeatCounter();
|
||||
|
||||
while (shouldRun)
|
||||
{
|
||||
// we grab these just for display purposes in this example
|
||||
uint32_t millis = heart->getMillis();
|
||||
uint32_t beats = heart->beatCounter();
|
||||
|
||||
// heartRate() requires that at least 5 seconds pass before
|
||||
// returning anything other than 0
|
||||
int hr = heart->heartRate();
|
||||
|
||||
// output milliseconds passed, beat count, and computed heart rate
|
||||
cout << "Millis: " << millis << " Beats: " << beats;
|
||||
cout << " Heart Rate: " << hr << endl;
|
||||
|
||||
sleep(1);
|
||||
}
|
||||
|
||||
heart->stopBeatCounter();
|
||||
//! [Interesting]
|
||||
|
||||
cout << "Exiting..." << endl;
|
||||
|
||||
delete heart;
|
||||
return 0;
|
||||
}
|
60
examples/javascript/groveehr.js
Normal file
60
examples/javascript/groveehr.js
Normal file
@ -0,0 +1,60 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
/*global */
|
||||
/*
|
||||
* Author: Zion Orent <zorent@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
// Load heart rate sensor module
|
||||
var heartRateSensor = require('jsupm_groveehr');
|
||||
// Instantiate a Grove Ear-clip Heart Rate sensor on digital pin D2
|
||||
var myHeartRateSensor = new heartRateSensor.GroveEHR(2);
|
||||
|
||||
// set the beat counter to 0, init the clock and start counting beats
|
||||
myHeartRateSensor.clearBeatCounter();
|
||||
myHeartRateSensor.initClock();
|
||||
myHeartRateSensor.startBeatCounter();
|
||||
|
||||
setInterval(readHeartRate, 1000);
|
||||
|
||||
function readHeartRate()
|
||||
{
|
||||
// we grab these just for display purposes in this example
|
||||
var millis = myHeartRateSensor.getMillis();
|
||||
var beats = myHeartRateSensor.beatCounter();
|
||||
|
||||
// heartRate() requires that at least 5 seconds pass before
|
||||
// returning anything other than 0
|
||||
var hr = myHeartRateSensor.heartRate();
|
||||
|
||||
// output milliseconds passed, beat count, and computed heart rate
|
||||
console.log("Millis: " + millis + " Beats: " + beats +
|
||||
" Heart Rate: " + hr);
|
||||
}
|
||||
|
||||
// Print message when exiting
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
console.log("Exiting...");
|
||||
process.exit(0);
|
||||
});
|
5
src/groveehr/CMakeLists.txt
Normal file
5
src/groveehr/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
||||
set (libname "groveehr")
|
||||
set (libdescription "upm grove ear-clip heart rate sensor module")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
129
src/groveehr/groveehr.cxx
Normal file
129
src/groveehr/groveehr.cxx
Normal file
@ -0,0 +1,129 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
|
||||
#include "groveehr.h"
|
||||
|
||||
using namespace upm;
|
||||
using namespace std;
|
||||
|
||||
GroveEHR::GroveEHR(int pin)
|
||||
{
|
||||
mraa_init();
|
||||
|
||||
if ( !(m_gpio = mraa_gpio_init(pin)) )
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_gpio_init() failed" << endl;
|
||||
return;
|
||||
}
|
||||
|
||||
mraa_gpio_dir(m_gpio, MRAA_GPIO_IN);
|
||||
|
||||
initClock();
|
||||
m_beatCounter = 0;
|
||||
}
|
||||
|
||||
GroveEHR::~GroveEHR()
|
||||
{
|
||||
mraa_gpio_close(m_gpio);
|
||||
}
|
||||
|
||||
void GroveEHR::initClock()
|
||||
{
|
||||
gettimeofday(&m_startTime, NULL);
|
||||
}
|
||||
|
||||
uint32_t GroveEHR::getMillis()
|
||||
{
|
||||
struct timeval elapsed, now;
|
||||
uint32_t elapse;
|
||||
|
||||
// get current time
|
||||
gettimeofday(&now, NULL);
|
||||
|
||||
// compute the delta since m_startTime
|
||||
if( (elapsed.tv_usec = now.tv_usec - m_startTime.tv_usec) < 0 )
|
||||
{
|
||||
elapsed.tv_usec += 1000000;
|
||||
elapsed.tv_sec = now.tv_sec - m_startTime.tv_sec - 1;
|
||||
}
|
||||
else
|
||||
{
|
||||
elapsed.tv_sec = now.tv_sec - m_startTime.tv_sec;
|
||||
}
|
||||
|
||||
elapse = (uint32_t)((elapsed.tv_sec * 1000) + (elapsed.tv_usec / 1000));
|
||||
|
||||
// never return 0
|
||||
if (elapse == 0)
|
||||
elapse = 1;
|
||||
|
||||
return elapse;
|
||||
}
|
||||
|
||||
void GroveEHR::clearBeatCounter()
|
||||
{
|
||||
m_beatCounter = 0;
|
||||
}
|
||||
|
||||
void GroveEHR::startBeatCounter()
|
||||
{
|
||||
// install our interrupt handler
|
||||
mraa_gpio_isr(m_gpio, MRAA_GPIO_EDGE_RISING,
|
||||
&beatISR, this);
|
||||
}
|
||||
|
||||
void GroveEHR::stopBeatCounter()
|
||||
{
|
||||
// remove the interrupt handler
|
||||
mraa_gpio_isr_exit(m_gpio);
|
||||
}
|
||||
|
||||
uint32_t GroveEHR::beatCounter()
|
||||
{
|
||||
return m_beatCounter;
|
||||
}
|
||||
|
||||
void GroveEHR::beatISR(void *ctx)
|
||||
{
|
||||
upm::GroveEHR *This = (upm::GroveEHR *)ctx;
|
||||
This->m_beatCounter++;
|
||||
}
|
||||
|
||||
int GroveEHR::heartRate()
|
||||
{
|
||||
uint32_t millis = getMillis();
|
||||
uint32_t beats = beatCounter();
|
||||
|
||||
float heartRate = 0;
|
||||
// wait at least 5 seconds before attempting to compute the
|
||||
// heart rate
|
||||
if (millis > 5000)
|
||||
{
|
||||
heartRate = (float(beats) / (float(millis) / 1000.0)) * 60.0;
|
||||
}
|
||||
|
||||
return int(heartRate);
|
||||
}
|
114
src/groveehr/groveehr.h
Normal file
114
src/groveehr/groveehr.h
Normal file
@ -0,0 +1,114 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <stdint.h>
|
||||
#include <sys/time.h>
|
||||
#include <mraa/gpio.h>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
* @brief C++ API for the Grove Ear-clip Heart Rate sensor
|
||||
*
|
||||
* UPM module for the GroveEHR sensor. It is used to measure your
|
||||
* heartbeat.
|
||||
*
|
||||
* @ingroup gpio
|
||||
* @snippet groveehr.cxx Interesting
|
||||
*/
|
||||
class GroveEHR {
|
||||
public:
|
||||
/**
|
||||
* GroveEHR constructor
|
||||
*
|
||||
* @param pin digital pin to use
|
||||
*/
|
||||
GroveEHR(int pin);
|
||||
/**
|
||||
* GroveEHR Destructor
|
||||
*/
|
||||
~GroveEHR();
|
||||
/**
|
||||
* Return the number of milliseconds elapsed since initClock()
|
||||
* was last called.
|
||||
*
|
||||
* @return elapsed milliseconds
|
||||
*/
|
||||
uint32_t getMillis();
|
||||
|
||||
/**
|
||||
* Reset the Clock
|
||||
*
|
||||
*/
|
||||
void initClock();
|
||||
|
||||
/**
|
||||
* Reset the beat counter to 0. The beat Counter should be
|
||||
* stopped via stopBeatCounter() prior to calling this function.
|
||||
*
|
||||
*/
|
||||
void clearBeatCounter();
|
||||
|
||||
/**
|
||||
* Start the beat counter
|
||||
*
|
||||
*/
|
||||
void startBeatCounter();
|
||||
|
||||
/**
|
||||
* Stop the beat counter
|
||||
*
|
||||
*/
|
||||
void stopBeatCounter();
|
||||
|
||||
/**
|
||||
* Get the beat Counter
|
||||
*
|
||||
* @return the beat counter
|
||||
*/
|
||||
uint32_t beatCounter();
|
||||
|
||||
/**
|
||||
* Beat Interrupt Service Routine
|
||||
*
|
||||
*/
|
||||
static void beatISR(void *ctx);
|
||||
|
||||
/**
|
||||
* Compute the heart rate
|
||||
*
|
||||
* @return the computed heart rate
|
||||
*/
|
||||
int heartRate();
|
||||
|
||||
private:
|
||||
volatile uint32_t m_beatCounter;
|
||||
struct timeval m_startTime;
|
||||
mraa_gpio_context m_gpio;
|
||||
};
|
||||
}
|
||||
|
||||
|
8
src/groveehr/jsupm_groveehr.i
Normal file
8
src/groveehr/jsupm_groveehr.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module jsupm_groveehr
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "groveehr.h"
|
||||
%}
|
||||
|
||||
%include "groveehr.h"
|
9
src/groveehr/pyupm_groveehr.i
Normal file
9
src/groveehr/pyupm_groveehr.i
Normal file
@ -0,0 +1,9 @@
|
||||
%module pyupm_groveehr
|
||||
%include "../upm.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%include "groveehr.h"
|
||||
%{
|
||||
#include "groveehr.h"
|
||||
%}
|
Reference in New Issue
Block a user