mirror of
https://github.com/eclipse/upm.git
synced 2025-03-15 04:57:30 +03:00
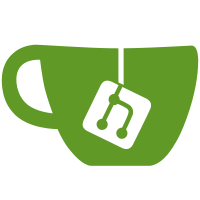
Many of the UPM libraries allocate space on the heap but do not explicitly handle copying and assignment. This commit uses C++11 delete to forbit both the copy and assignment operator for these classes. The C++ examples which used assignment operators to initialize class instances were also updated since it did not appear necessary in those cases to use the assignment operator. Signed-off-by: Noel Eck <noel.eck@intel.com>
137 lines
4.7 KiB
C++
137 lines
4.7 KiB
C++
/*
|
|
* Author: Jon Trulson <jtrulson@ics.com>
|
|
* Copyright (c) 2017 Intel Corporation.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining
|
|
* a copy of this software and associated documentation files (the
|
|
* "Software"), to deal in the Software without restriction, including
|
|
* without limitation the rights to use, copy, modify, merge, publish,
|
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
|
* permit persons to whom the Software is furnished to do so, subject to
|
|
* the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be
|
|
* included in all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
*/
|
|
|
|
#include <unistd.h>
|
|
#include <iostream>
|
|
#include <string>
|
|
|
|
#include "rn2903.hpp"
|
|
#include "upm_utilities.h"
|
|
|
|
using namespace std;
|
|
|
|
int main(int argc, char **argv)
|
|
{
|
|
//! [Interesting]
|
|
|
|
string defaultDev = "/dev/ttyUSB0";
|
|
if (argc > 1)
|
|
defaultDev = argv[1];
|
|
|
|
cout << "Using device: " << defaultDev << endl;
|
|
|
|
// Instantiate a RN2903 sensor on defaultDev at 57600 baud.
|
|
upm::RN2903 sensor(defaultDev, RN2903_DEFAULT_BAUDRATE);
|
|
|
|
// To use an internal UART understood by MRAA, use the following
|
|
// to inititialize rather than the above, which by default uses a
|
|
// tty path.
|
|
//
|
|
// upm::RN2903 sensor = upm::RN2903(0, RN2903_DEFAULT_BAUDRATE);
|
|
|
|
// enable debugging
|
|
// sensor.setDebug(true);
|
|
|
|
// get version
|
|
if (sensor.command("sys get ver"))
|
|
{
|
|
cout << "Failed to retrieve device version string" << endl;
|
|
return 1;
|
|
}
|
|
cout << "Firmware version: " << sensor.getResponse() << endl;
|
|
|
|
cout << "Hardware EUI: " << sensor.getHardwareEUI() << endl;
|
|
|
|
// we can support two types of join, OTAA and ABP. Each requires
|
|
// that certain parameters be set first. We will only attempt ABP
|
|
// joining with this example since it's the only one that can
|
|
// succeed without actual configuration. In both cases, if you
|
|
// are actually attempting to join a real LoRaWAN network, you
|
|
// must change the parameters below to match the network you are
|
|
// attempting to join.
|
|
|
|
// For OTAA, you need to supply valid Device EUI, Application EUI,
|
|
// and Application key:
|
|
//
|
|
// sensor.setDeviceEUI("0011223344556677");
|
|
// sensor.setApplicationEUI("0011223344556677");
|
|
// sensor.setApplicationKey("01234567012345670123456701234567");
|
|
//
|
|
// RN2903_JOIN_STATUS_T rv = sensor.join(RN2903_JOIN_TYPE_OTAA);
|
|
// A successful join will return RN2903_JOIN_STATUS_ACCEPTED (0).
|
|
// cout << "JOIN: got rv " << int(rv) << endl;
|
|
|
|
// Try an ABP join. Note, these parameters are made up. For a
|
|
// real network, you will want to use the correct values
|
|
// obviously. For an ABP join, you need to supply the Device
|
|
// Address, Network Session Key, and the Application Session Key.
|
|
|
|
sensor.setDeviceAddr("00112233");
|
|
sensor.setNetworkSessionKey("00112233001122330011223300112233");
|
|
sensor.setApplicationSessionKey("00112233001122330011223300112233");
|
|
|
|
RN2903_JOIN_STATUS_T rv = sensor.join(RN2903_JOIN_TYPE_ABP);
|
|
if (rv == RN2903_JOIN_STATUS_ACCEPTED)
|
|
{
|
|
cout << "Join successful." << endl;
|
|
|
|
// All transmit payloads must be hex encoded strings, so
|
|
// pretend we have a temperature sensor that gave us a value
|
|
// of 25.6 C, and we want to transmit it.
|
|
string faketemp = "25.6";
|
|
cout << "Transmitting a packet..." << endl;
|
|
|
|
RN2903_MAC_TX_STATUS_T trv;
|
|
trv = sensor.macTx(RN2903_MAC_MSG_TYPE_UNCONFIRMED,
|
|
1, // port number
|
|
sensor.toHex(faketemp));
|
|
|
|
if (trv == RN2903_MAC_TX_STATUS_TX_OK)
|
|
cout << "Transmit successful." << endl;
|
|
else
|
|
{
|
|
// check to see if we got a downlink packet
|
|
if (trv == RN2903_MAC_TX_STATUS_RX_RECEIVED)
|
|
{
|
|
cout << "Transmit successful, downlink packet received: "
|
|
<< sensor.getResponse();
|
|
}
|
|
else
|
|
{
|
|
cout << "Transmit failed with code " << int(trv) << endl;
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
cout << "Join failed with code " << int(rv) << endl;
|
|
}
|
|
|
|
cout << "Exiting" << endl;
|
|
|
|
//! [Interesting]
|
|
|
|
return 0;
|
|
}
|