mirror of
https://github.com/eclipse/upm.git
synced 2025-03-15 04:57:30 +03:00
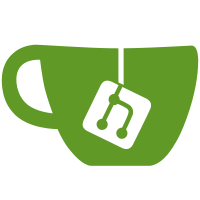
* Updated pom file generation: Generate pom files after all sensor library targets have been created - allows for dependencies * Changes for compiling on Android * Check for mraa build options: Look at symbols in mraa library to determine UPM build options (example: mraa_iio_init, mraa_firmata_init) * Add per target summary for C/C++/java/nodejs/python * Added hierarchy to fti include directory... old: #include "upm_voltage.h" new: #include "fti/upm_voltage.h" * Removed unimplemented methods from mpu9150 library and java example * Add utilities-c target for all c examples. Most of the C examples rely on the upm_delay methods. Add a dependency on the utilities-c target for all c examples. * Updated the examples/CMakeLists.txt to add dependencies passed via TARGETS to the target name parsed from the example name. Also updated the interface example names to start with 'interfaces'. * Updated src/examples/CMakeLists.txt to ALWAYS remove examples from the example_src_list (moved this from end of function to beginning). Signed-off-by: Noel Eck <noel.eck@intel.com>
204 lines
7.0 KiB
CMake
204 lines
7.0 KiB
CMake
find_package(Java REQUIRED)
|
|
include(UseJava)
|
|
|
|
macro(add_example example_name jar_name)
|
|
set(example_src "${example_name}.java")
|
|
set(example_jar "${CMAKE_CURRENT_BINARY_DIR}/../../src/${jar_name}/upm_${jar_name}.jar")
|
|
|
|
add_jar(${example_name} SOURCES ${example_src} INCLUDE_JARS ${example_jar})
|
|
add_dependencies(${example_name} javaupm_${jar_name})
|
|
endmacro()
|
|
|
|
macro(add_example_with_path example_name jar_path jar_name)
|
|
set(example_src "${example_name}.java")
|
|
set(example_jar "${CMAKE_CURRENT_BINARY_DIR}/../../src/${jar_path}/upm_${jar_name}.jar")
|
|
|
|
add_jar(${example_name} SOURCES ${example_src} INCLUDE_JARS ${example_jar})
|
|
add_dependencies(${example_name} javaupm_${jar_name})
|
|
endmacro()
|
|
|
|
add_example(A110X_intrSample a110x)
|
|
add_example(A110XSample a110x)
|
|
add_example(ADC121C021Sample adc121c021)
|
|
add_example(Adxl345Sample adxl345)
|
|
add_example(Apds9002 apds9002)
|
|
add_example(BISS0001Sample biss0001)
|
|
add_example(BMPX8XSample bmpx8x)
|
|
add_example(BuzzerSample buzzer)
|
|
add_example(CJQ4435Sample cjq4435)
|
|
add_example(DS1307Sample ds1307)
|
|
add_example(ENC03RSample enc03r)
|
|
add_example(ES08ASample servo)
|
|
add_example(ButtonSample button)
|
|
add_example(GroveButtonSample grove)
|
|
add_example(Button_intrSample button)
|
|
add_example(GroveButton_intrSample grove)
|
|
add_example(Collision collision)
|
|
add_example(EHRSample ehr)
|
|
add_example(GroveEHRSample groveehr)
|
|
add_example(Emg emg)
|
|
add_example(GroveEmg groveemg)
|
|
add_example(Gsr gsr)
|
|
add_example(GroveGsr grovegsr)
|
|
add_example(LEDSample led)
|
|
add_example(LightSample light)
|
|
add_example(GroveLightSample grove)
|
|
add_example(GroveLineFinderSample grovelinefinder)
|
|
add_example(LineFinderSample linefinder)
|
|
add_example(GroveLed_multiSample grove)
|
|
add_example(GroveLEDSample grove)
|
|
add_example(GroveMDSample grovemd)
|
|
add_example(MDSample md)
|
|
add_example(MoistureSample moisture)
|
|
add_example(GroveMoistureSample grovemoisture)
|
|
add_example(GroveMQ3 gas)
|
|
add_example(GroveMQ9 gas)
|
|
add_example(O2Example o2)
|
|
add_example(GroveO2Example groveo2)
|
|
add_example(GroveQTouch at42qt1070)
|
|
add_example(RelaySample relay)
|
|
add_example(GroveRelaySample grove)
|
|
add_example(RotarySample rotary)
|
|
add_example(GroveRotarySample grove)
|
|
add_example(GROVESCAMSample grovescam)
|
|
add_example(SCAMSample scam)
|
|
add_example(SlideSample slide)
|
|
add_example(GroveSlideSample grove)
|
|
add_example(SpeakerSample speaker)
|
|
add_example(GroveSpeakerSample grovespeaker)
|
|
add_example(TemperatureSample temperature)
|
|
add_example(GroveTempSample grove)
|
|
add_example(VDivSample vdiv)
|
|
add_example(GroveVDivSample grovevdiv)
|
|
add_example(WaterSample water)
|
|
add_example(GroveWaterSample grovewater)
|
|
add_example(GroveWFSSample grovewfs)
|
|
add_example(WFSSample wfs)
|
|
add_example(GUVAS12DSample guvas12d)
|
|
add_example(H3LIS331DLSample h3lis331dl)
|
|
add_example(HCSR04Sample hcsr04)
|
|
add_example(HM11Sample hm11)
|
|
add_example(Hmc5883lSample hmc5883l)
|
|
add_example(HMTRPSample hmtrp)
|
|
add_example(HP20xExample hp20x)
|
|
add_example(HTU21DSample htu21d)
|
|
add_example(Itg3200Sample itg3200)
|
|
add_example(Joystick12Sample joystick12)
|
|
add_example(LDT0028Sample ldt0028)
|
|
add_example(LoLSample lol)
|
|
add_example(LSM303DLHSample lsm303dlh)
|
|
add_example(M24LR64ESample m24lr64e)
|
|
add_example(MAX44000Sample max44000)
|
|
add_example(MHZ16Sample mhz16)
|
|
add_example(MicrophoneSample mic)
|
|
add_example(MMA7455Sample mma7455)
|
|
add_example(MMA7660Sample mma7660)
|
|
add_example(MPL3115A2Sample mpl3115a2)
|
|
add_example(MPR121Sample mpr121)
|
|
add_example(MPU9150Sample mpu9150)
|
|
add_example(MQ2Sample gas)
|
|
add_example(MQ303ASample mq303a)
|
|
add_example(MQ5Sample gas)
|
|
add_example(GroveLEDBar my9221)
|
|
add_example(NRF24L01_receiverSample nrf24l01)
|
|
add_example(NRF24L01_transmitterSample nrf24l01)
|
|
add_example(NUNCHUCKSample nunchuck)
|
|
add_example(OTP538USample otp538u)
|
|
add_example(PPD42NSSample ppd42ns)
|
|
add_example(PulsensorSample pulsensor)
|
|
add_example(RFR359FSample rfr359f)
|
|
add_example(RotaryEncoderSample rotaryencoder)
|
|
add_example(RPR220_intrSample rpr220)
|
|
add_example(RPR220Sample rpr220)
|
|
add_example(ST7735Sample st7735)
|
|
if (NOT ANDROID)
|
|
add_example(StepMotorSample stepmotor)
|
|
endif ()
|
|
add_example(TM1637Sample tm1637)
|
|
add_example(TP401Sample gas)
|
|
add_example(TSL2561Sample tsl2561)
|
|
add_example(TTP223Sample ttp223)
|
|
add_example(ULN200XASample uln200xa)
|
|
add_example(WaterLevelSensor waterlevel)
|
|
add_example(WT5001Sample wt5001)
|
|
add_example(YG1006Sample yg1006)
|
|
add_example(ZFM20Sample zfm20)
|
|
add_example(Ad8232Example ad8232)
|
|
add_example(Gp2y0aExample gp2y0a)
|
|
add_example(Th02Example th02)
|
|
add_example(FlexSensorExample flex)
|
|
add_example(CWLSXXA_Example cwlsxxa)
|
|
add_example(TEAMS_Example teams)
|
|
add_example(APA102Sample apa102)
|
|
add_example(TEX00_Example tex00)
|
|
add_example(BMI160_Example bmi160)
|
|
add_example(Tsl2561 tsl2561)
|
|
add_example(AM2315Example am2315)
|
|
add_example(MAX31855Example max31855)
|
|
add_example(MAX5487Example max5487)
|
|
add_example(MAXds3231mExample maxds3231m)
|
|
add_example(ECS1030Example ecs1030)
|
|
add_example(SM130Example sm130)
|
|
if (MODBUS_FOUND)
|
|
add_example(H803X_Example h803x)
|
|
endif()
|
|
if (BACNET_FOUND)
|
|
add_example(E50HX_Example e50hx)
|
|
add_example(T8100_Example t8100)
|
|
add_example(TB7300_Example tb7300)
|
|
endif()
|
|
if (JPEG_FOUND)
|
|
add_example(VCAP_Example vcap)
|
|
endif()
|
|
add_example(BMP280_Example bmp280)
|
|
add_example(BNO055_Example bno055)
|
|
add_example(BMX055_Example bmx055)
|
|
add_example(NMEAGPS_Example nmea_gps)
|
|
add_example(MMA7361_Example mma7361)
|
|
add_example(BH1750_Example bh1750)
|
|
add_example(HKA5_Example hka5)
|
|
add_example(DFRORP_Example dfrorp)
|
|
add_example(DFREC_Example dfrec)
|
|
add_example(SHT1X_Example sht1x)
|
|
add_example(MS5803_Example ms5803)
|
|
add_example(ECEZO_Example ecezo)
|
|
add_example(IMS_Example ims)
|
|
add_example(MB704X_Example mb704x)
|
|
add_example(MCP2515_Example mcp2515)
|
|
add_example(Ads1015Sample ads1x15)
|
|
add_example(MAX30100_Example max30100)
|
|
add_example(Ads1115Sample ads1x15)
|
|
add_example(SensorTemplateSample sensortemplate)
|
|
add_example(P9813Sample p9813)
|
|
add_example(BMG160_Example bmg160)
|
|
add_example(BMA250E_Example bma250e)
|
|
add_example(BMM150_Example bmm150)
|
|
add_example(LSM303AGR_Example lsm303agr)
|
|
add_example(LSM303D_Example lsm303d)
|
|
|
|
add_example_with_path(Jhd1313m1_lcdSample jhd1313m1 jhd1313m1)
|
|
add_example_with_path(Jhd1313m1Sample jhd1313m1 jhd1313m1)
|
|
add_example_with_path(Lcm1602_i2cSample lcm1602 lcm1602)
|
|
add_example_with_path(Lcm1602_parallelSample lcm1602 lcm1602)
|
|
add_example_with_path(SSD1308_oledSample lcd i2clcd)
|
|
add_example_with_path(SSD1327_oledSample lcd i2clcd)
|
|
add_example_with_path(BME280_Example bmp280 bmp280)
|
|
|
|
if(SWIG_VERSION VERSION_GREATER 3.0.8)
|
|
add_example_with_path(BME280_InterfaceExample bmp280 bmp280)
|
|
endif()
|
|
|
|
add_example_with_path(BMC150_Example bmx055 bmx055)
|
|
add_example_with_path(BMI055_Example bmx055 bmx055)
|
|
if (OPENZWAVE_FOUND)
|
|
add_example_with_path(AeotecSS6_Example ozw ozw)
|
|
add_example_with_path(AeotecSDG2_Example ozw ozw)
|
|
add_example_with_path(AeotecDW2E_Example ozw ozw)
|
|
add_example_with_path(AeotecDSB09104_Example ozw ozw)
|
|
add_example_with_path(TZEMT400_Example ozw ozw)
|
|
endif()
|
|
add_example_with_path(NMEAGPS_I2C_Example nmea_gps nmea_gps)
|
|
add_example_with_path(MCP2515_TXRX_Example mcp2515 mcp2515)
|
|
add_example_with_path(LE910_Example uartat uartat)
|
|
add_example_with_path(SpeakerPWMSample speaker speaker)
|