mirror of
https://github.com/eclipse/upm.git
synced 2025-03-15 04:57:30 +03:00
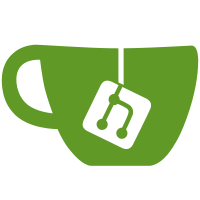
The API has been changed in some cases - see the apichanges.md document. In addition, this driver uses a new upm_vectortypes.i SWIG interface file to provide a mechanism for methods that return a vector of floats and ints instead of a pointer to an array. This works much nicer than C array pointers, and results in Python/JS/Java code that looks much more "natural" to the language in use. The Python, JS, and Java examples have been changed to use these methods. Support for the "old" C-style pointer methods are still provided for backward compatibility with existing code. As an example - to retrieve the x, y, and z data for Euler Angles from the bno055, the original python code would look something like: ... x = sensorObj.new_floatp() y = sensorObj.new_floatp() z = sensorObj.new_floatp() ... sensor.getEulerAngles(x, y, z) ... print("Euler: Heading:", sensorObj.floatp_value(x), end=' ') print(" Roll:", sensorObj.floatp_value(y), end=' ') ... Now the equivalent code is simply: floatData = sensor.getEulerAngles() print("Euler: Heading:", floatData[0], ... print(" Roll:", floatData[1], end=' ') ... Additionally, interrupt handling for Java is now implemented completely in the C++ header file now rather than the .cxx file, so no special SWIG processing is required anymore. See Issue #518 . Signed-off-by: Jon Trulson <jtrulson@ics.com>
102 lines
3.9 KiB
Java
102 lines
3.9 KiB
Java
/*
|
|
* Author: Jon Trulson <jtrulson@ics.com>
|
|
* Copyright (c) 2016-2017 Intel Corporation.
|
|
*
|
|
* The MIT License
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining
|
|
* a copy of this software and associated documentation files (the
|
|
* "Software"), to deal in the Software without restriction, including
|
|
* without limitation the rights to use, copy, modify, merge, publish,
|
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
|
* permit persons to whom the Software is furnished to do so, subject to
|
|
* the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be
|
|
* included in all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
*/
|
|
|
|
import upm_bno055.BNO055;
|
|
|
|
public class BNO055_Example
|
|
{
|
|
public static void main(String[] args) throws InterruptedException
|
|
{
|
|
// ! [Interesting]
|
|
System.out.println("Initializing...");
|
|
|
|
// Instantiate an BNO055 using default parameters (bus 0, addr
|
|
// 0x28). The default running mode is NDOF absolute orientation
|
|
// mode.
|
|
BNO055 sensor = new BNO055();
|
|
|
|
System.out.println("First we need to calibrate. 4 numbers will be output every");
|
|
System.out.println("second for each sensor. 0 means uncalibrated, and 3 means");
|
|
System.out.println("fully calibrated.");
|
|
System.out.println("See the UPM documentation on this sensor for instructions on");
|
|
System.out.println("what actions are required to calibrate.");
|
|
System.out.println("");
|
|
|
|
while (!sensor.isFullyCalibrated())
|
|
{
|
|
upm_bno055.intVector calData = sensor.getCalibrationStatus();
|
|
|
|
System.out.println("Magnetometer: " + calData.get(0)
|
|
+ " Accelerometer: " + calData.get(1)
|
|
+ " Gyroscope: " + calData.get(2)
|
|
+ " System: " + calData.get(3));
|
|
|
|
Thread.sleep(1000);
|
|
|
|
}
|
|
|
|
System.out.println("");
|
|
System.out.println("Calibration complete.");
|
|
System.out.println("");
|
|
|
|
while (true)
|
|
{
|
|
// update our values from the sensor
|
|
sensor.update();
|
|
|
|
upm_bno055.floatVector data = sensor.getEulerAngles();
|
|
|
|
System.out.println("Euler: Heading: " + data.get(0)
|
|
+ " Roll: " + data.get(1)
|
|
+ " Pitch: " + data.get(2)
|
|
+ " degrees");
|
|
|
|
data = sensor.getQuaternions();
|
|
System.out.println("Quaternion: W: " + data.get(0)
|
|
+ " X: " + data.get(1)
|
|
+ " Y: " + data.get(2)
|
|
+ " Z: " + data.get(3));
|
|
|
|
data = sensor.getLinearAcceleration();
|
|
System.out.println("Linear Acceleration: X: " + data.get(0)
|
|
+ " Y: " + data.get(1)
|
|
+ " Z: " + data.get(2)
|
|
+ " m/s^2");
|
|
|
|
data = sensor.getGravityVectors();
|
|
System.out.println("Gravity Vector: X: " + data.get(0)
|
|
+ " Y: " + data.get(1)
|
|
+ " Z: " + data.get(2)
|
|
+ " m/s^2");
|
|
|
|
System.out.println();
|
|
Thread.sleep(250);
|
|
}
|
|
|
|
// ! [Interesting]
|
|
}
|
|
}
|