mirror of
https://github.com/eclipse/upm.git
synced 2025-07-19 02:01:15 +03:00
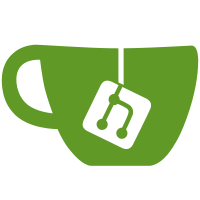
There are a variety of LSM303 devices out there with various incompatibilities and differing capabilities. The current lsm303 driver in UPM only supports the LSM303DLH variant, so it has been renamed to lsm303dlh to avoid confusion and to make it clear which variant is actually supported. All examples and source files have been renamed, including header files. In addition, the class name, LSM303, has been renamed to LSM303DLH. No other functionality or behavior has been changed. Signed-off-by: Jon Trulson <jtrulson@ics.com>
77 lines
2.8 KiB
JavaScript
77 lines
2.8 KiB
JavaScript
/*
|
|
* Author: Zion Orent <zorent@ics.com>
|
|
* Copyright (c) 2014 Intel Corporation.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining
|
|
* a copy of this software and associated documentation files (the
|
|
* "Software"), to deal in the Software without restriction, including
|
|
* without limitation the rights to use, copy, modify, merge, publish,
|
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
|
* permit persons to whom the Software is furnished to do so, subject to
|
|
* the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be
|
|
* included in all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
*/
|
|
|
|
var accelrCompassSensor = require('jsupm_lsm303dlh');
|
|
|
|
// Instantiate LSM303DLH compass on I2C
|
|
var myAccelrCompass = new accelrCompassSensor.LSM303DLH(0);
|
|
|
|
var successFail, coords, outputStr, accel;
|
|
var myInterval = setInterval(function()
|
|
{
|
|
// Load coordinates into LSM303DLH object
|
|
successFail = myAccelrCompass.getCoordinates();
|
|
// in XYZ order. The sensor returns XZY,
|
|
// but the driver compensates and makes it XYZ
|
|
coords = myAccelrCompass.getRawCoorData();
|
|
|
|
// Print out the X, Y, and Z coordinate data using two different methods
|
|
outputStr = "coor: rX " + coords.getitem(0)
|
|
+ " - rY " + coords.getitem(1)
|
|
+ " - rZ " + coords.getitem(2);
|
|
console.log(outputStr);
|
|
outputStr = "coor: gX " + myAccelrCompass.getCoorX()
|
|
+ " - gY " + myAccelrCompass.getCoorY()
|
|
+ " - gZ " + myAccelrCompass.getCoorZ();
|
|
console.log(outputStr);
|
|
|
|
// Get and print out the heading
|
|
console.log("heading: " + myAccelrCompass.getHeading());
|
|
|
|
// Get the acceleration
|
|
myAccelrCompass.getAcceleration();
|
|
accel = myAccelrCompass.getRawAccelData();
|
|
// Print out the X, Y, and Z acceleration data using two different methods
|
|
outputStr = "acc: rX " + accel.getitem(0)
|
|
+ " - rY " + accel.getitem(1)
|
|
+ " - Z " + accel.getitem(2);
|
|
console.log(outputStr);
|
|
outputStr = "acc: gX " + myAccelrCompass.getAccelX()
|
|
+ " - gY " + myAccelrCompass.getAccelY()
|
|
+ " - gZ " + myAccelrCompass.getAccelZ();
|
|
console.log(outputStr);
|
|
console.log(" ");
|
|
}, 1000);
|
|
|
|
// Print message when exiting
|
|
process.on('SIGINT', function()
|
|
{
|
|
clearInterval(myInterval);
|
|
myAccelrCompass = null;
|
|
accelrCompassSensor.cleanUp();
|
|
accelrCompassSensor = null;
|
|
console.log("Exiting");
|
|
process.exit(0);
|
|
});
|