mirror of
https://github.com/eclipse/upm.git
synced 2025-07-02 01:41:12 +03:00
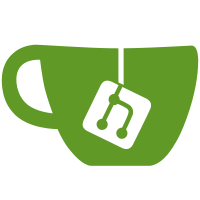
This module (bmx055) implements support for the following core Bosch chipsets: bma250e - accelerometer, 3 variants (chip id's 0x03, 0xf9, and 0xfa) bmm150 - magnetometer bmg160 - gyroscope The other 3 devices are combinations of the above: bmx055 - accel/gyro/mag bmc160 - accel/mag bmi055 - accel/gyro ...for 6 devices total. For the combination devices, all of the sub-devices appear as individual independent devices on the I2C/SPI bus. The combination drivers provide basic configuration and data output. For more detailed control as well as interrupt support, you should use the core device drivers (accel/gyro/mag) directly. These devices support both I2C and SPI communications. They must be powered at 3.3vdc. Signed-off-by: Jon Trulson <jtrulson@ics.com>
65 lines
2.3 KiB
Java
65 lines
2.3 KiB
Java
/*
|
|
* Author: Jon Trulson <jtrulson@ics.com>
|
|
* Copyright (c) 2016 Intel Corporation.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining
|
|
* a copy of this software and associated documentation files (the
|
|
* "Software"), to deal in the Software without restriction, including
|
|
* without limitation the rights to use, copy, modify, merge, publish,
|
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
|
* permit persons to whom the Software is furnished to do so, subject to
|
|
* the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be
|
|
* included in all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
*/
|
|
|
|
import upm_bmx055.BMG160;
|
|
|
|
public class BMG160_Example
|
|
{
|
|
public static void main(String[] args) throws InterruptedException
|
|
{
|
|
// ! [Interesting]
|
|
|
|
// Instantiate a BMG160 instance using default i2c bus and address
|
|
BMG160 sensor = new BMG160();
|
|
|
|
// For SPI, bus 0, you would pass -1 as the address, and a
|
|
// valid pin for CS:
|
|
// BMG160(0, -1, 10);
|
|
|
|
while (true)
|
|
{
|
|
// update our values from the sensor
|
|
sensor.update();
|
|
|
|
float dataA[] = sensor.getGyroscope();
|
|
|
|
System.out.println("Gyroscope x: " + dataA[0]
|
|
+ " y: " + dataA[1]
|
|
+ " z: " + dataA[2]
|
|
+ " degrees/s");
|
|
|
|
System.out.println("Compensation Temperature: "
|
|
+ sensor.getTemperature()
|
|
+ " C / "
|
|
+ sensor.getTemperature(true)
|
|
+ " F");
|
|
|
|
System.out.println();
|
|
Thread.sleep(250);
|
|
}
|
|
|
|
// ! [Interesting]
|
|
}
|
|
}
|