mirror of
https://github.com/eclipse/upm.git
synced 2025-03-19 23:17:29 +03:00
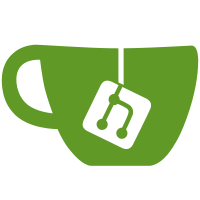
To make room for UPM C and C++ sensor code to coexist, all UPM C++ headers have been renamed from h -> hpp. This commit contains updates to documentation, includes, cmake collateral, examples, and swig interface files. * Renamed all cxx/cpp header files which contain the string 'copyright intel' from .h -> .hpp (if not already hpp). * Replaced all references to .h with .hpp in documentation, source files, cmake collateral, example code, and swig interface files. * Replaced cmake variable module_h with module_hpp. * Intentionally left upm.h since this file currently does not contain code (documentation only). Signed-off-by: Noel Eck <noel.eck@intel.com>
81 lines
1.7 KiB
C++
81 lines
1.7 KiB
C++
#include <iostream>
|
|
#include <stdexcept>
|
|
#include <unistd.h>
|
|
#include "hlg150h.hpp"
|
|
#include "mraa-utils.hpp"
|
|
|
|
#define PWM_PERIOD 3333
|
|
|
|
using namespace upm;
|
|
|
|
HLG150H::HLG150H(int pinRelay, int pinPWM)
|
|
{
|
|
int dutyPercent = 0;
|
|
status = mraa::SUCCESS;
|
|
this->pinRelay = pinRelay;
|
|
isPoweredShadow = false;
|
|
pwmBrightness = new mraa::Pwm(pinPWM);
|
|
status = pwmBrightness->enable(true);
|
|
status = pwmBrightness->period_us(PWM_PERIOD);
|
|
if (status != mraa::SUCCESS)
|
|
UPM_THROW("pwm config failed.");
|
|
dutyPercent = getBrightness();
|
|
isPoweredShadow = dutyPercent > 10;
|
|
}
|
|
|
|
HLG150H::~HLG150H()
|
|
{
|
|
delete pwmBrightness;
|
|
}
|
|
|
|
void HLG150H::setPowerOn()
|
|
{
|
|
isPoweredShadow = true;
|
|
MraaUtils::setGpio(pinRelay, 0);
|
|
}
|
|
|
|
void HLG150H::setPowerOff()
|
|
{
|
|
isPoweredShadow = false;
|
|
MraaUtils::setGpio(pinRelay, 1);
|
|
}
|
|
|
|
bool HLG150H::isPowered()
|
|
{
|
|
// Can't read GPIO state as setting in to input mode turns off relay
|
|
// Instead we return a shadow variable
|
|
/*
|
|
int level;
|
|
if (MraaUtils::getGpio(pinRelay, &level) == MRAA_SUCCESS)
|
|
return level == 1;
|
|
else
|
|
return false;
|
|
*/
|
|
return isPoweredShadow;
|
|
}
|
|
|
|
|
|
// If duty is less than 10% light will flicker
|
|
void HLG150H::setBrightness(int dutyPercent)
|
|
{
|
|
if (dutyPercent < 10)
|
|
dutyPercent = 10;
|
|
int dutyUs = (PWM_PERIOD * dutyPercent) / 100;
|
|
dutyUs = PWM_PERIOD - dutyUs;
|
|
status = pwmBrightness->pulsewidth_us(dutyUs);
|
|
// std::cout << "Brightness = " << dutyPercent << "%, duty = " << dutyUs << "us" << std::endl;
|
|
if (status != mraa::SUCCESS)
|
|
UPM_THROW("setBrightness failed");
|
|
|
|
}
|
|
|
|
|
|
int HLG150H::getBrightness()
|
|
{
|
|
float duty = pwmBrightness->read();
|
|
int dutyPercent = static_cast<int>(100.0 * (1.0 - duty) + 0.5);
|
|
return dutyPercent;
|
|
}
|
|
|
|
|