mirror of
https://github.com/eclipse/upm.git
synced 2025-07-02 01:41:12 +03:00
at42qt1070: Add additional functionality
Add functionality to read and set the low-power mode settings Add functions to read and set the averaging factor per key Add functions to read and set the adjecent key suppression (AKS) groups Add a function to read the ID register Add a sanity check to the constructor Signed-off-by: Wouter van Verre <wouter.van.verre@intel.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
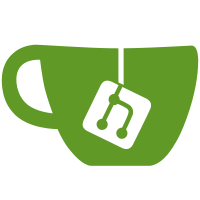
committed by
Mihai Tudor Panu
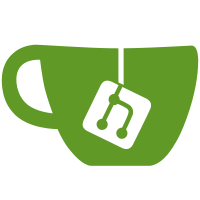
parent
f64c710c12
commit
057fa03723
@ -26,6 +26,7 @@
|
||||
#include <math.h>
|
||||
#include <iostream>
|
||||
#include <string>
|
||||
#include <stdexcept>
|
||||
|
||||
#include "at42qt1070.h"
|
||||
|
||||
@ -51,6 +52,10 @@ AT42QT1070::AT42QT1070(int bus, uint8_t address)
|
||||
return;
|
||||
}
|
||||
|
||||
if (readChipID() != 0x2E) {
|
||||
throw std::runtime_error("Chip ID does not match the expected value (2Eh)");
|
||||
}
|
||||
|
||||
m_buttonStates = false;
|
||||
m_calibrating = false;
|
||||
m_overflow = false;
|
||||
@ -101,6 +106,12 @@ AT42QT1070::readWord(uint8_t reg)
|
||||
return mraa_i2c_read_word_data(m_i2c, reg);
|
||||
}
|
||||
|
||||
uint8_t
|
||||
AT42QT1070::readChipID(void)
|
||||
{
|
||||
return readByte(REG_CHIPID);
|
||||
}
|
||||
|
||||
void
|
||||
AT42QT1070::updateState()
|
||||
{
|
||||
@ -129,6 +140,102 @@ AT42QT1070::updateState()
|
||||
}
|
||||
}
|
||||
|
||||
uint8_t
|
||||
AT42QT1070::getLPMode(void)
|
||||
{
|
||||
return readByte(REG_LP);
|
||||
}
|
||||
|
||||
uint8_t
|
||||
AT42QT1070::setLPMode(uint8_t mode)
|
||||
{
|
||||
writeByte(REG_LP, mode);
|
||||
|
||||
return getLPMode();
|
||||
}
|
||||
|
||||
uint8_t
|
||||
AT42QT1070::getAVE(uint8_t key)
|
||||
{
|
||||
uint8_t value, ave;
|
||||
|
||||
if (key > 6) {
|
||||
throw std::invalid_argument("Only keys 0-6 are allowed");
|
||||
}
|
||||
|
||||
value = readByte(REG_AVE0 + key);
|
||||
ave = (value & 0xFC) >> 2;
|
||||
|
||||
return ave;
|
||||
}
|
||||
|
||||
uint8_t
|
||||
AT42QT1070::setAVE(uint8_t key, uint8_t ave)
|
||||
{
|
||||
uint8_t value;
|
||||
|
||||
if (key > 6) {
|
||||
throw std::invalid_argument("Only keys 0-6 are allowed");
|
||||
}
|
||||
|
||||
switch (ave) {
|
||||
case 1:
|
||||
case 2:
|
||||
case 4:
|
||||
case 8:
|
||||
case 16:
|
||||
case 32:
|
||||
break;
|
||||
|
||||
default:
|
||||
throw std::invalid_argument("Invalid averaging factor");
|
||||
}
|
||||
|
||||
value = readByte(REG_AVE0 + key);
|
||||
value = value & 0x03;
|
||||
value = value | (ave << 2);
|
||||
writeByte(REG_AVE0 + key, value);
|
||||
|
||||
return getAVE(key);
|
||||
}
|
||||
|
||||
uint8_t
|
||||
AT42QT1070::getAKSGroup(uint8_t key)
|
||||
{
|
||||
uint8_t value, aks;
|
||||
|
||||
if (key > 6) {
|
||||
throw std::invalid_argument("Only keys 0-6 are allowed");
|
||||
}
|
||||
|
||||
value = readByte(REG_AVE0 + key);
|
||||
aks = value & 0x03;
|
||||
|
||||
return aks;
|
||||
}
|
||||
|
||||
uint8_t
|
||||
AT42QT1070::setAKSGroup(uint8_t key, uint8_t group)
|
||||
{
|
||||
uint8_t value;
|
||||
|
||||
if (key > 6) {
|
||||
throw std::invalid_argument("Only keys 0-6 are allowed");
|
||||
}
|
||||
|
||||
if (group > 3) {
|
||||
throw std::invalid_argument("Only groups 0-3 are allowed");
|
||||
}
|
||||
|
||||
value = readByte(REG_AVE0 + key);
|
||||
value = value & 0xFC;
|
||||
value = value | group;
|
||||
|
||||
writeByte(REG_AVE0 + key, value);
|
||||
|
||||
return getAKSGroup(key);
|
||||
}
|
||||
|
||||
bool
|
||||
AT42QT1070::reset()
|
||||
{
|
||||
|
@ -189,6 +189,14 @@ class AT42QT1070
|
||||
*/
|
||||
uint16_t readWord(uint8_t reg);
|
||||
|
||||
|
||||
/**
|
||||
* Read the Chip ID register on the sensor
|
||||
*
|
||||
* @return the value of the Chip ID register
|
||||
*/
|
||||
uint8_t readChipID(void);
|
||||
|
||||
/**
|
||||
* Read the current touch status and detection state
|
||||
*
|
||||
@ -196,6 +204,57 @@ class AT42QT1070
|
||||
*/
|
||||
void updateState();
|
||||
|
||||
|
||||
/**
|
||||
* Read the current low-power mode setting
|
||||
*
|
||||
* @return return low-power mode setting from sensor
|
||||
*/
|
||||
uint8_t getLPMode(void);
|
||||
|
||||
/**
|
||||
* Change the low-pomer mode setting on the sensor
|
||||
*
|
||||
* @param mode desired new mode
|
||||
* @return new setting on sensor
|
||||
*/
|
||||
uint8_t setLPMode(uint8_t mode);
|
||||
|
||||
|
||||
/**
|
||||
* Read the current averaging factor setting for a key
|
||||
*
|
||||
* @param key the key being read
|
||||
* @return the averaging factor
|
||||
*/
|
||||
uint8_t getAVE(uint8_t key);
|
||||
|
||||
/**
|
||||
* Change the averaging factor setting for a key
|
||||
*
|
||||
* @param key the key being changed
|
||||
* @param ave the new averaging factor
|
||||
* @return the new averaging factor as read from the device
|
||||
*/
|
||||
uint8_t setAVE(uint8_t key, uint8_t ave);
|
||||
|
||||
/**
|
||||
* Read the AKS group a key is part of
|
||||
*
|
||||
* @param key the key (0-6) being queried
|
||||
* @return AKS group the key is part of
|
||||
*/
|
||||
uint8_t getAKSGroup(uint8_t key);
|
||||
|
||||
/**
|
||||
* Change the AKS group that a key is part of
|
||||
*
|
||||
* @param key the key (0-6) being changed
|
||||
* @param group the new group for the key
|
||||
* @return return the new value on the sensor
|
||||
*/
|
||||
uint8_t setAKSGroup(uint8_t key, uint8_t group);
|
||||
|
||||
/**
|
||||
* return the overflow indicator
|
||||
*
|
||||
|
Reference in New Issue
Block a user