mirror of
https://github.com/eclipse/upm.git
synced 2025-07-05 19:31:15 +03:00
at42qt1070: code style changes
Run .clang-format on the header and source files Add missing braces Signed-off-by: Wouter van Verre <wouter.van.verre@intel.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
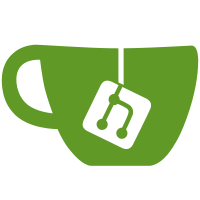
committed by
Mihai Tudor Panu
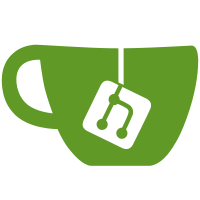
parent
32921ff2b1
commit
f64c710c12
@ -35,111 +35,110 @@ using namespace std;
|
||||
|
||||
AT42QT1070::AT42QT1070(int bus, uint8_t address)
|
||||
{
|
||||
m_addr = address;
|
||||
m_addr = address;
|
||||
|
||||
// setup our i2c link
|
||||
if ( !(m_i2c = mraa_i2c_init(bus)) )
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_init() failed." << endl;
|
||||
return;
|
||||
}
|
||||
|
||||
mraa_result_t rv;
|
||||
|
||||
if ( (rv = mraa_i2c_address(m_i2c, m_addr)) != MRAA_SUCCESS)
|
||||
{
|
||||
cerr << __FUNCTION__ << ": Could not initialize i2c bus. " << endl;
|
||||
mraa_result_print(rv);
|
||||
return;
|
||||
// setup our i2c link
|
||||
if (!(m_i2c = mraa_i2c_init(bus))) {
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_init() failed." << endl;
|
||||
return;
|
||||
}
|
||||
|
||||
m_buttonStates = false;
|
||||
m_calibrating = false;
|
||||
m_overflow = false;
|
||||
mraa_result_t rv;
|
||||
|
||||
if ((rv = mraa_i2c_address(m_i2c, m_addr)) != MRAA_SUCCESS) {
|
||||
cerr << __FUNCTION__ << ": Could not initialize i2c bus. " << endl;
|
||||
mraa_result_print(rv);
|
||||
return;
|
||||
}
|
||||
|
||||
m_buttonStates = false;
|
||||
m_calibrating = false;
|
||||
m_overflow = false;
|
||||
}
|
||||
|
||||
AT42QT1070::~AT42QT1070()
|
||||
{
|
||||
mraa_i2c_stop(m_i2c);
|
||||
mraa_i2c_stop(m_i2c);
|
||||
}
|
||||
|
||||
bool AT42QT1070::writeByte(uint8_t reg, uint8_t byte)
|
||||
bool
|
||||
AT42QT1070::writeByte(uint8_t reg, uint8_t byte)
|
||||
{
|
||||
mraa_result_t rv = mraa_i2c_write_byte_data(m_i2c, byte, reg);
|
||||
mraa_result_t rv = mraa_i2c_write_byte_data(m_i2c, byte, reg);
|
||||
|
||||
if (rv != MRAA_SUCCESS)
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_write_byte() failed." << endl;
|
||||
mraa_result_print(rv);
|
||||
return false;
|
||||
if (rv != MRAA_SUCCESS) {
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_write_byte() failed." << endl;
|
||||
mraa_result_print(rv);
|
||||
return false;
|
||||
}
|
||||
|
||||
return true;
|
||||
return true;
|
||||
}
|
||||
|
||||
bool AT42QT1070::writeWord(uint8_t reg, uint16_t word)
|
||||
bool
|
||||
AT42QT1070::writeWord(uint8_t reg, uint16_t word)
|
||||
{
|
||||
mraa_result_t rv = mraa_i2c_write_word_data(m_i2c, word, reg);
|
||||
mraa_result_t rv = mraa_i2c_write_word_data(m_i2c, word, reg);
|
||||
|
||||
if (rv != MRAA_SUCCESS)
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_write_word() failed." << endl;
|
||||
mraa_result_print(rv);
|
||||
return false;
|
||||
if (rv != MRAA_SUCCESS) {
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_write_word() failed." << endl;
|
||||
mraa_result_print(rv);
|
||||
return false;
|
||||
}
|
||||
|
||||
return true;
|
||||
return true;
|
||||
}
|
||||
|
||||
uint8_t AT42QT1070::readByte(uint8_t reg)
|
||||
uint8_t
|
||||
AT42QT1070::readByte(uint8_t reg)
|
||||
{
|
||||
return mraa_i2c_read_byte_data(m_i2c, reg);
|
||||
return mraa_i2c_read_byte_data(m_i2c, reg);
|
||||
}
|
||||
|
||||
uint16_t AT42QT1070::readWord(uint8_t reg)
|
||||
uint16_t
|
||||
AT42QT1070::readWord(uint8_t reg)
|
||||
{
|
||||
return mraa_i2c_read_word_data(m_i2c, reg);
|
||||
return mraa_i2c_read_word_data(m_i2c, reg);
|
||||
}
|
||||
|
||||
void AT42QT1070::updateState()
|
||||
void
|
||||
AT42QT1070::updateState()
|
||||
{
|
||||
uint8_t stat = readByte(REG_DETSTATUS);
|
||||
uint8_t stat = readByte(REG_DETSTATUS);
|
||||
|
||||
// if we are calibrating, don't change anything
|
||||
if (stat & DET_CALIBRATE)
|
||||
{
|
||||
m_calibrating = true;
|
||||
return;
|
||||
// if we are calibrating, don't change anything
|
||||
if (stat & DET_CALIBRATE) {
|
||||
m_calibrating = true;
|
||||
return;
|
||||
} else {
|
||||
m_calibrating = false;
|
||||
}
|
||||
else
|
||||
m_calibrating = false;
|
||||
|
||||
if (stat & DET_OVERFLOW)
|
||||
m_overflow = true;
|
||||
else
|
||||
m_overflow = false;
|
||||
if (stat & DET_OVERFLOW)
|
||||
m_overflow = true;
|
||||
else
|
||||
m_overflow = false;
|
||||
|
||||
// if a touch is occurring, read the button states
|
||||
if (stat & DET_TOUCH)
|
||||
{
|
||||
uint8_t keys = readByte(REG_KEYSTATUS);
|
||||
// high bit is reserved, filter it out
|
||||
m_buttonStates = keys & ~0x80;
|
||||
// if a touch is occurring, read the button states
|
||||
if (stat & DET_TOUCH) {
|
||||
uint8_t keys = readByte(REG_KEYSTATUS);
|
||||
// high bit is reserved, filter it out
|
||||
m_buttonStates = keys & ~0x80;
|
||||
} else {
|
||||
m_buttonStates = 0;
|
||||
}
|
||||
else
|
||||
m_buttonStates = 0;
|
||||
}
|
||||
|
||||
bool AT42QT1070::reset()
|
||||
bool
|
||||
AT42QT1070::reset()
|
||||
{
|
||||
// write a non-zero value to the reset register
|
||||
return writeByte(REG_RESET, 0xff);
|
||||
// write a non-zero value to the reset register
|
||||
return writeByte(REG_RESET, 0xff);
|
||||
}
|
||||
|
||||
bool AT42QT1070::calibrate()
|
||||
bool
|
||||
AT42QT1070::calibrate()
|
||||
{
|
||||
// write a non-zero value to the calibrate register
|
||||
return writeByte(REG_CALIBRATE, 0xff);
|
||||
// write a non-zero value to the calibrate register
|
||||
return writeByte(REG_CALIBRATE, 0xff);
|
||||
}
|
||||
|
||||
|
@ -32,109 +32,111 @@
|
||||
#define AT42QT1070_I2C_BUS 0
|
||||
#define AT42QT1070_DEFAULT_I2C_ADDR 0x1b
|
||||
|
||||
namespace upm {
|
||||
namespace upm
|
||||
{
|
||||
/**
|
||||
* @brief Atmel AT42QT1070 QTouch sensor library
|
||||
* @defgroup at42qt1070 libupm-at42qt1070
|
||||
* @ingroup seeed i2c touch
|
||||
*/
|
||||
|
||||
/**
|
||||
* @brief Atmel AT42QT1070 QTouch sensor library
|
||||
* @defgroup at42qt1070 libupm-at42qt1070
|
||||
* @ingroup seeed i2c touch
|
||||
*/
|
||||
|
||||
/**
|
||||
* @library at42qt1070
|
||||
* @sensor at42qt1070
|
||||
* @comname AT42QT1070 QTouch Sensor
|
||||
* @altname Grove QTouch Sensor
|
||||
* @type touch
|
||||
* @man seeed
|
||||
* @con i2c
|
||||
*
|
||||
* @brief API for the Atmel AT42QT1070 QTouch sensor
|
||||
*
|
||||
* This class implements support for the Atmel AT42QT1070 QTouch
|
||||
* sensor, which supports 7 capacitive buttons.
|
||||
*
|
||||
* It was developed using the Grove Q Touch Sensor board.
|
||||
*
|
||||
* @image html at42qt1070.jpg
|
||||
* @snippet at42qt1070.cxx Interesting
|
||||
*/
|
||||
class AT42QT1070 {
|
||||
/**
|
||||
* @library at42qt1070
|
||||
* @sensor at42qt1070
|
||||
* @comname AT42QT1070 QTouch Sensor
|
||||
* @altname Grove QTouch Sensor
|
||||
* @type touch
|
||||
* @man seeed
|
||||
* @con i2c
|
||||
*
|
||||
* @brief API for the Atmel AT42QT1070 QTouch sensor
|
||||
*
|
||||
* This class implements support for the Atmel AT42QT1070 QTouch
|
||||
* sensor, which supports 7 capacitive buttons.
|
||||
*
|
||||
* It was developed using the Grove Q Touch Sensor board.
|
||||
*
|
||||
* @image html at42qt1070.jpg
|
||||
* @snippet at42qt1070.cxx Interesting
|
||||
*/
|
||||
class AT42QT1070
|
||||
{
|
||||
public:
|
||||
|
||||
// registers
|
||||
typedef enum { REG_CHIPID = 0,
|
||||
REG_FWVERS = 1,
|
||||
typedef enum {
|
||||
REG_CHIPID = 0,
|
||||
REG_FWVERS = 1,
|
||||
|
||||
REG_DETSTATUS = 2, // detection status
|
||||
REG_KEYSTATUS = 3, // key status
|
||||
REG_DETSTATUS = 2, // detection status
|
||||
REG_KEYSTATUS = 3, // key status
|
||||
|
||||
REG_KEYSIG0_H = 4, // key signal
|
||||
REG_KEYSIG0_L = 5,
|
||||
REG_KEYSIG1_H = 6,
|
||||
REG_KEYSIG1_L = 7,
|
||||
REG_KEYSIG2_H = 8,
|
||||
REG_KEYSIG2_L = 9,
|
||||
REG_KEYSIG3_H = 10,
|
||||
REG_KEYSIG3_L = 11,
|
||||
REG_KEYSIG4_H = 12,
|
||||
REG_KEYSIG4_L = 13,
|
||||
REG_KEYSIG5_H = 14,
|
||||
REG_KEYSIG5_L = 15,
|
||||
REG_KEYSIG6_H = 16,
|
||||
REG_KEYSIG6_L = 17,
|
||||
REG_KEYSIG0_H = 4, // key signal
|
||||
REG_KEYSIG0_L = 5,
|
||||
REG_KEYSIG1_H = 6,
|
||||
REG_KEYSIG1_L = 7,
|
||||
REG_KEYSIG2_H = 8,
|
||||
REG_KEYSIG2_L = 9,
|
||||
REG_KEYSIG3_H = 10,
|
||||
REG_KEYSIG3_L = 11,
|
||||
REG_KEYSIG4_H = 12,
|
||||
REG_KEYSIG4_L = 13,
|
||||
REG_KEYSIG5_H = 14,
|
||||
REG_KEYSIG5_L = 15,
|
||||
REG_KEYSIG6_H = 16,
|
||||
REG_KEYSIG6_L = 17,
|
||||
|
||||
REG_REFDATA0_H = 18, // key reference data
|
||||
REG_REFDATA0_L = 19,
|
||||
REG_REFDATA1_H = 20,
|
||||
REG_REFDATA1_L = 21,
|
||||
REG_REFDATA2_H = 22,
|
||||
REG_REFDATA2_L = 23,
|
||||
REG_REFDATA3_H = 24,
|
||||
REG_REFDATA3_L = 25,
|
||||
REG_REFDATA4_H = 26,
|
||||
REG_REFDATA4_L = 27,
|
||||
REG_REFDATA5_H = 28,
|
||||
REG_REFDATA5_L = 29,
|
||||
REG_REFDATA6_H = 30,
|
||||
REG_REFDATA6_L = 31,
|
||||
REG_REFDATA0_H = 18, // key reference data
|
||||
REG_REFDATA0_L = 19,
|
||||
REG_REFDATA1_H = 20,
|
||||
REG_REFDATA1_L = 21,
|
||||
REG_REFDATA2_H = 22,
|
||||
REG_REFDATA2_L = 23,
|
||||
REG_REFDATA3_H = 24,
|
||||
REG_REFDATA3_L = 25,
|
||||
REG_REFDATA4_H = 26,
|
||||
REG_REFDATA4_L = 27,
|
||||
REG_REFDATA5_H = 28,
|
||||
REG_REFDATA5_L = 29,
|
||||
REG_REFDATA6_H = 30,
|
||||
REG_REFDATA6_L = 31,
|
||||
|
||||
REG_NTHR0 = 32, // negative threshold level
|
||||
REG_NTHR1 = 33,
|
||||
REG_NTHR2 = 34,
|
||||
REG_NTHR3 = 35,
|
||||
REG_NTHR4 = 36,
|
||||
REG_NTHR5 = 37,
|
||||
REG_NTHR6 = 38,
|
||||
REG_NTHR0 = 32, // negative threshold level
|
||||
REG_NTHR1 = 33,
|
||||
REG_NTHR2 = 34,
|
||||
REG_NTHR3 = 35,
|
||||
REG_NTHR4 = 36,
|
||||
REG_NTHR5 = 37,
|
||||
REG_NTHR6 = 38,
|
||||
|
||||
REG_AVE0 = 39, // key suppression
|
||||
REG_AVE1 = 40,
|
||||
REG_AVE2 = 41,
|
||||
REG_AVE3 = 42,
|
||||
REG_AVE4 = 43,
|
||||
REG_AVE5 = 44,
|
||||
REG_AVE6 = 45,
|
||||
REG_AVE0 = 39, // key suppression
|
||||
REG_AVE1 = 40,
|
||||
REG_AVE2 = 41,
|
||||
REG_AVE3 = 42,
|
||||
REG_AVE4 = 43,
|
||||
REG_AVE5 = 44,
|
||||
REG_AVE6 = 45,
|
||||
|
||||
REG_DI0 = 46, // detection integrator
|
||||
REG_DI1 = 47,
|
||||
REG_DI2 = 48,
|
||||
REG_DI3 = 49,
|
||||
REG_DI4 = 50,
|
||||
REG_DI5 = 51,
|
||||
REG_DI6 = 52,
|
||||
REG_DI0 = 46, // detection integrator
|
||||
REG_DI1 = 47,
|
||||
REG_DI2 = 48,
|
||||
REG_DI3 = 49,
|
||||
REG_DI4 = 50,
|
||||
REG_DI5 = 51,
|
||||
REG_DI6 = 52,
|
||||
|
||||
REG_GUARD = 53, // FastOutDI/Max Cal/Guard channel
|
||||
REG_LP = 54, // low power
|
||||
REG_MAXON = 55, // max on duration
|
||||
REG_CALIBRATE = 56,
|
||||
REG_RESET = 57
|
||||
REG_GUARD = 53, // FastOutDI/Max Cal/Guard channel
|
||||
REG_LP = 54, // low power
|
||||
REG_MAXON = 55, // max on duration
|
||||
REG_CALIBRATE = 56,
|
||||
REG_RESET = 57
|
||||
} AT42QT1070_REG_T;
|
||||
|
||||
// detection register bits
|
||||
typedef enum { DET_TOUCH = 0x01,
|
||||
// 0x02-0x20 reserved
|
||||
DET_OVERFLOW = 0x40,
|
||||
DET_CALIBRATE = 0x80
|
||||
typedef enum {
|
||||
DET_TOUCH = 0x01,
|
||||
// 0x02-0x20 reserved
|
||||
DET_OVERFLOW = 0x40,
|
||||
DET_CALIBRATE = 0x80
|
||||
} AT42QT1070_DET_T;
|
||||
|
||||
|
||||
@ -199,14 +201,22 @@ namespace upm {
|
||||
*
|
||||
* @return true if overflow indicated
|
||||
*/
|
||||
bool isOverflowed() { return m_overflow; };
|
||||
bool
|
||||
isOverflowed()
|
||||
{
|
||||
return m_overflow;
|
||||
};
|
||||
|
||||
/**
|
||||
* return the calibrating indicator
|
||||
*
|
||||
* @return true if calibration is in progress
|
||||
*/
|
||||
bool isCalibrating() { return m_calibrating; };
|
||||
bool
|
||||
isCalibrating()
|
||||
{
|
||||
return m_calibrating;
|
||||
};
|
||||
|
||||
/**
|
||||
* Issue a reset command
|
||||
@ -227,7 +237,11 @@ namespace upm {
|
||||
*
|
||||
* @returns the button states
|
||||
*/
|
||||
uint8_t getButtons() { return m_buttonStates; };
|
||||
uint8_t
|
||||
getButtons()
|
||||
{
|
||||
return m_buttonStates;
|
||||
};
|
||||
|
||||
private:
|
||||
uint8_t m_buttonStates;
|
||||
@ -236,7 +250,5 @@ namespace upm {
|
||||
|
||||
mraa_i2c_context m_i2c;
|
||||
uint8_t m_addr;
|
||||
};
|
||||
};
|
||||
}
|
||||
|
||||
|
||||
|
Reference in New Issue
Block a user