mirror of
https://github.com/eclipse/upm.git
synced 2025-07-15 16:21:11 +03:00
nunchuck: Initial implementation
This was tested with the Grove Nunchuck adapter. Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Zion Orent <zorent@ics.com> Signed-off-by: John Van Drasek <john.r.van.drasek@intel.com>
This commit is contained in:
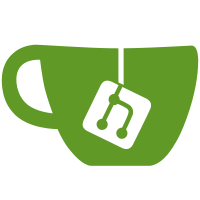
committed by
John Van Drasek
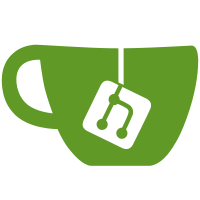
parent
780500ac0a
commit
1f3d074261
@ -84,6 +84,7 @@ add_executable (mma7660-example mma7660.cxx)
|
||||
add_executable (cjq4435-example cjq4435.cxx)
|
||||
add_executable (adxl335-example adxl335.cxx)
|
||||
add_executable (hmtrp-example hmtrp.cxx)
|
||||
add_executable (nunchuck-example nunchuck.cxx)
|
||||
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/hmc5883l)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grove)
|
||||
@ -153,6 +154,7 @@ include_directories (${PROJECT_SOURCE_DIR}/src/mma7660)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/cjq4435)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/adxl335)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/hmtrp)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/nunchuck)
|
||||
|
||||
target_link_libraries (hmc5883l-example hmc5883l ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (groveled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||
@ -240,3 +242,4 @@ target_link_libraries (mma7660-example mma7660 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (cjq4435-example cjq4435 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (adxl335-example adxl335 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (hmtrp-example hmtrp ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (nunchuck-example nunchuck ${CMAKE_THREAD_LIBS_INIT})
|
||||
|
66
examples/javascript/nunchuck.js
Normal file
66
examples/javascript/nunchuck.js
Normal file
@ -0,0 +1,66 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
|
||||
/*
|
||||
* Author: Zion Orent <zorent@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
var nunchuck_lib = require('jsupm_nunchuck');
|
||||
|
||||
// Instantiate a nunchuck controller bus 0
|
||||
var nunchuck_obj = new nunchuck_lib.NUNCHUCK(0);
|
||||
|
||||
// always do this first
|
||||
console.log("Initializing... ");
|
||||
if (!nunchuck_obj.init())
|
||||
{
|
||||
console.log("nunchuck->init() failed.");
|
||||
process.exit(0);
|
||||
}
|
||||
|
||||
setInterval(function()
|
||||
{
|
||||
nunchuck_obj.update();
|
||||
|
||||
var outputStr = "stickX: " + nunchuck_obj.stickX +
|
||||
", stickY: " + nunchuck_obj.stickY;
|
||||
console.log(outputStr);
|
||||
outputStr = "accelX: " + nunchuck_obj.accelX +
|
||||
", accelY: " + nunchuck_obj.accelY +
|
||||
", accelZ: " + nunchuck_obj.accelZ;
|
||||
console.log(outputStr);
|
||||
|
||||
outputStr = "button C: " +
|
||||
((nunchuck_obj.buttonC) ? "pressed" : "not pressed");
|
||||
console.log(outputStr);
|
||||
outputStr = "button Z: " +
|
||||
((nunchuck_obj.buttonZ) ? "pressed" : "not pressed");
|
||||
console.log(outputStr);
|
||||
}, 100);
|
||||
|
||||
// Print message when exiting
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
console.log("Exiting...");
|
||||
process.exit(0);
|
||||
});
|
79
examples/nunchuck.cxx
Normal file
79
examples/nunchuck.cxx
Normal file
@ -0,0 +1,79 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
|
||||
#include "nunchuck.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
bool shouldRun = true;
|
||||
|
||||
void sig_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
shouldRun = false;
|
||||
}
|
||||
|
||||
int main(int argc, char **argv)
|
||||
{
|
||||
signal(SIGINT, sig_handler);
|
||||
|
||||
//! [Interesting]
|
||||
// Instantiate a nunchuck controller bus 0
|
||||
upm::NUNCHUCK *nunchuck = new upm::NUNCHUCK(0);
|
||||
|
||||
// always do this first
|
||||
cout << "Initializing... " << endl;
|
||||
if (!nunchuck->init())
|
||||
{
|
||||
cerr << "nunchuck->init() failed." << endl;
|
||||
return 0;
|
||||
}
|
||||
|
||||
while (shouldRun)
|
||||
{
|
||||
nunchuck->update();
|
||||
|
||||
cout << "stickX: " << nunchuck->stickX
|
||||
<< ", stickY: " << nunchuck->stickY << endl;
|
||||
cout << "accelX: " << nunchuck->accelX
|
||||
<< ", accelY: " << nunchuck->accelY
|
||||
<< ", accelZ: " << nunchuck->accelZ << endl;
|
||||
|
||||
cout << "button C: "
|
||||
<< ((nunchuck->buttonC) ? "pressed" : "not pressed") << endl;
|
||||
cout << "button Z: "
|
||||
<< ((nunchuck->buttonZ) ? "pressed" : "not pressed") << endl;
|
||||
cout << endl;
|
||||
|
||||
usleep(100000);
|
||||
}
|
||||
//! [Interesting]
|
||||
|
||||
delete nunchuck;
|
||||
return 0;
|
||||
}
|
5
src/nunchuck/CMakeLists.txt
Normal file
5
src/nunchuck/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
||||
set (libname "nunchuck")
|
||||
set (libdescription "upm wii nunchuck module")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
8
src/nunchuck/jsupm_nunchuck.i
Normal file
8
src/nunchuck/jsupm_nunchuck.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module jsupm_nunchuck
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "nunchuck.h"
|
||||
%}
|
||||
|
||||
%include "nunchuck.h"
|
143
src/nunchuck/nunchuck.cxx
Normal file
143
src/nunchuck/nunchuck.cxx
Normal file
@ -0,0 +1,143 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
#include <string>
|
||||
#include <unistd.h>
|
||||
|
||||
#include "nunchuck.h"
|
||||
|
||||
using namespace upm;
|
||||
using namespace std;
|
||||
|
||||
|
||||
NUNCHUCK::NUNCHUCK(int bus, uint8_t addr)
|
||||
{
|
||||
stickX = 0;
|
||||
stickY = 0;
|
||||
accelX = 0;
|
||||
accelY = 0;
|
||||
accelZ = 0;
|
||||
buttonC = false;
|
||||
buttonZ = false;
|
||||
|
||||
// setup our i2c link
|
||||
m_i2c = mraa_i2c_init(bus);
|
||||
|
||||
mraa_result_t rv;
|
||||
|
||||
if ( (rv = mraa_i2c_address(m_i2c, addr)) != MRAA_SUCCESS )
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_address() failed." << endl;
|
||||
mraa_result_print(rv);
|
||||
}
|
||||
}
|
||||
|
||||
NUNCHUCK::~NUNCHUCK()
|
||||
{
|
||||
mraa_i2c_stop(m_i2c);
|
||||
}
|
||||
|
||||
bool NUNCHUCK::writeByte(uint8_t reg, uint8_t byte)
|
||||
{
|
||||
mraa_result_t rv;
|
||||
|
||||
if ( (rv = mraa_i2c_write_byte_data(m_i2c, byte, reg)) != MRAA_SUCCESS )
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_i2c_write_byte_data() failed." << endl;
|
||||
mraa_result_print(rv);
|
||||
return false;
|
||||
}
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
uint8_t NUNCHUCK::readBytes(uint8_t reg, uint8_t *buffer, unsigned int len)
|
||||
{
|
||||
if (!len || !buffer)
|
||||
return 0;
|
||||
|
||||
mraa_i2c_address(m_i2c, NUNCHUCK_I2C_ADDR);
|
||||
mraa_i2c_write_byte(m_i2c, reg);
|
||||
|
||||
return mraa_i2c_read(m_i2c, buffer, len);
|
||||
}
|
||||
|
||||
bool NUNCHUCK::init()
|
||||
{
|
||||
usleep(1000000);
|
||||
|
||||
// disable encryption
|
||||
if (!writeByte(0xf0, 0x55))
|
||||
return false;
|
||||
|
||||
if (!writeByte(0xfb, 0x00))
|
||||
return false;
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
void NUNCHUCK::update()
|
||||
{
|
||||
const int bufsize = 6;
|
||||
uint8_t buf[bufsize];
|
||||
int rv;
|
||||
|
||||
rv = readBytes(0x00, buf, bufsize);
|
||||
|
||||
if (rv != bufsize)
|
||||
{
|
||||
cerr << __FUNCTION__ << "read failed, expected " << bufsize
|
||||
<< "bytes, got " << rv << endl;
|
||||
return;
|
||||
}
|
||||
|
||||
// analog stick X
|
||||
stickX = buf[0];
|
||||
|
||||
// analog stick Y
|
||||
stickY = buf[1];
|
||||
|
||||
// accelerometer X
|
||||
accelX = ( (buf[2] << 2) | ((buf[5] & 0x0c) >> 2) );
|
||||
|
||||
// accelerometer Y
|
||||
accelY = ( (buf[3] << 2) | ((buf[5] & 0x30) >> 4) );
|
||||
|
||||
// accelerometer Z
|
||||
accelZ = ( (buf[4] << 2) | ((buf[5] & 0xc0) >> 6) );
|
||||
|
||||
// buttonC
|
||||
if (buf[5] & 0x02)
|
||||
buttonC = false;
|
||||
else
|
||||
buttonC = true;
|
||||
|
||||
// buttonZ
|
||||
if (buf[5] & 0x01)
|
||||
buttonZ = false;
|
||||
else
|
||||
buttonZ = true;
|
||||
}
|
||||
|
148
src/nunchuck/nunchuck.h
Normal file
148
src/nunchuck/nunchuck.h
Normal file
@ -0,0 +1,148 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <mraa/i2c.h>
|
||||
|
||||
#define NUNCHUCK_I2C_ADDR 0x52
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
* @brief C++ API for the Wii Nunchuck
|
||||
*
|
||||
* UPM module for the Wii Nunchuck. This module was tested with the
|
||||
* Wii Nunchuck connected to I2C via a Grove Wii Nunchuck adapter.
|
||||
*
|
||||
* See http://wiibrew.org/wiki/Wiimote/Extension_Controllers and
|
||||
* http://wiibrew.org/wiki/Wiimote/Extension_Controllers/Nunchuck
|
||||
* for more detailed information on the controller and it's protocol.
|
||||
*
|
||||
* A warning for the Grove nunchuck adapter: It has 2 traces on one
|
||||
* side, and 3 traces on the other. Do not match these up with the
|
||||
* nunchuck connector's traces. The nunchuck connector 'groove'
|
||||
* should be on the same side as the grove interface socket on the
|
||||
* adapter.
|
||||
*
|
||||
* @ingroup nunchuck
|
||||
* @defgroup nunchuck libupm-nunchuck
|
||||
* @snippet nunchuck.cxx Interesting
|
||||
*/
|
||||
class NUNCHUCK {
|
||||
public:
|
||||
/**
|
||||
* NUNCHUCK constructor
|
||||
*
|
||||
* @param bus i2c bus to use
|
||||
* @param addr i2c address to use
|
||||
*/
|
||||
NUNCHUCK(int bus, uint8_t addr=NUNCHUCK_I2C_ADDR);
|
||||
|
||||
/**
|
||||
* NUNCHUCK destructor
|
||||
*/
|
||||
~NUNCHUCK();
|
||||
|
||||
/**
|
||||
* Write value(s) into registers
|
||||
*
|
||||
* @param reg register location to start writing into
|
||||
* @param byte byte to write
|
||||
* @return true if successful
|
||||
*/
|
||||
bool writeByte(uint8_t reg, uint8_t byte);
|
||||
|
||||
/**
|
||||
* Read value(s) from registers
|
||||
*
|
||||
* @param reg register location to start reading from
|
||||
* @param buffer buffer for data storage
|
||||
* @param len number of bytes to read
|
||||
* @return number of bytes read
|
||||
*/
|
||||
uint8_t readBytes(uint8_t reg, uint8_t *buffer, unsigned int len);
|
||||
|
||||
/**
|
||||
* Initialize the controller. Here, we disable encryption after
|
||||
* delaying for a time to ensure the controller is ready.
|
||||
*
|
||||
* @return true if initialized successfully
|
||||
*/
|
||||
bool init();
|
||||
|
||||
/**
|
||||
* Read and update the current state of the controller.
|
||||
*
|
||||
*/
|
||||
void update();
|
||||
|
||||
/**
|
||||
* Current analog stick X position
|
||||
*
|
||||
*/
|
||||
int stickX;
|
||||
|
||||
/**
|
||||
* Current analog stick Y position
|
||||
*
|
||||
*/
|
||||
int stickY;
|
||||
|
||||
/**
|
||||
* Current accelerometer X value
|
||||
*
|
||||
*/
|
||||
int accelX;
|
||||
|
||||
/**
|
||||
* Current accelerometer Y value
|
||||
*
|
||||
*/
|
||||
int accelY;
|
||||
|
||||
/**
|
||||
* Current accelerometer Z value
|
||||
*
|
||||
*/
|
||||
int accelZ;
|
||||
|
||||
/**
|
||||
* Button C pressed?
|
||||
*
|
||||
*/
|
||||
bool buttonC;
|
||||
|
||||
/**
|
||||
* Button Z pressed?
|
||||
*
|
||||
*/
|
||||
bool buttonZ;
|
||||
|
||||
private:
|
||||
mraa_i2c_context m_i2c;
|
||||
};
|
||||
}
|
||||
|
||||
|
9
src/nunchuck/pyupm_nunchuck.i
Normal file
9
src/nunchuck/pyupm_nunchuck.i
Normal file
@ -0,0 +1,9 @@
|
||||
%module pyupm_nunchuck
|
||||
%include "../upm.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%include "nunchuck.h"
|
||||
%{
|
||||
#include "nunchuck.h"
|
||||
%}
|
Reference in New Issue
Block a user