mirror of
https://github.com/eclipse/upm.git
synced 2025-07-01 01:11:10 +03:00
examples: Added c++ interface based sensor/actuator examples
Signed-off-by: Henry Bruce <henry.bruce@intel.com> Signed-off-by: Abhishek Malik <abhishek.malik@intel.com>
This commit is contained in:
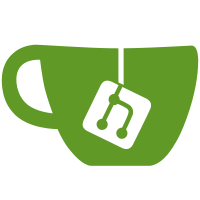
committed by
Abhishek Malik
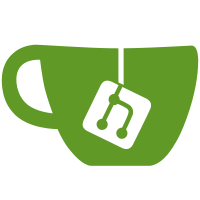
parent
f2b8921f1e
commit
389bd32f81
@ -258,3 +258,10 @@ add_custom_example (ak8975-example ak8975.cxx mpu9150)
|
||||
add_custom_example (mpu9250-example mpu9250.cxx mpu9150)
|
||||
add_custom_example (groveledbar-example groveledbar.cxx my9221)
|
||||
add_custom_example (grovecircularled-example grovecircularled.cxx my9221)
|
||||
add_custom_example (temperature-sensor-example temperature-sensor.cxx "si7005;bmp180;bme280")
|
||||
add_custom_example (humidity-sensor-example humidity-sensor.cxx "si7005;bme280")
|
||||
add_custom_example (pressure-sensor-example pressure-sensor.cxx "bmp180;bme280")
|
||||
add_custom_example (co2-sensor-example co2-sensor.cxx "t6713")
|
||||
#add_custom_example (adc-example adc-sensor.cxx ads1015)
|
||||
add_custom_example (light-sensor-example light-sensor.cxx "si1132;max44009")
|
||||
add_custom_example (light-controller-example light-controller.cxx "lp8860;ds1808lc;hlg150h")
|
||||
|
76
examples/c++/adc-sensor.cxx
Normal file
76
examples/c++/adc-sensor.cxx
Normal file
@ -0,0 +1,76 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include "ads1015.h"
|
||||
|
||||
#define EDISON_I2C_BUS 1
|
||||
#define FT4222_I2C_BUS 0
|
||||
|
||||
|
||||
//! [Interesting]
|
||||
// Simple example of using IADC to determine
|
||||
// which sensor is present and return its name.
|
||||
// IADC is then used to get readings from sensor
|
||||
|
||||
|
||||
upm::IADC* getADC()
|
||||
{
|
||||
upm::IADC* adc = NULL;
|
||||
try {
|
||||
adc = new upm::ADS1015(EDISON_I2C_BUS);
|
||||
return adc;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "ADS1015: " << e.what() << std::endl;
|
||||
}
|
||||
return adc;
|
||||
}
|
||||
|
||||
int main ()
|
||||
{
|
||||
upm::IADC* adc = getADC();
|
||||
if (adc == NULL) {
|
||||
std::cout << "ADC not detected" << std::endl;
|
||||
return 1;
|
||||
}
|
||||
std::cout << "ADC " << adc->getModuleName() << " detected. " ;
|
||||
std::cout << adc->getNumInputs() << " inputs available" << std::endl;
|
||||
while (true) {
|
||||
for (int i=0; i<adc->getNumInputs(); ++i) {
|
||||
std::cout << "Input " << i;
|
||||
try {
|
||||
float voltage = adc->getVoltage(i);
|
||||
std::cout << ": Voltage = " << voltage << "V" << std::endl;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << e.what() << std::endl;
|
||||
}
|
||||
}
|
||||
sleep(1);
|
||||
}
|
||||
delete adc;
|
||||
return 0;
|
||||
}
|
||||
|
||||
//! [Interesting]
|
71
examples/c++/co2-sensor.cxx
Normal file
71
examples/c++/co2-sensor.cxx
Normal file
@ -0,0 +1,71 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include "t6713.h"
|
||||
|
||||
#define EDISON_I2C_BUS 1
|
||||
#define FT4222_I2C_BUS 0
|
||||
|
||||
//! [Interesting]
|
||||
// Simple example of using ICO2Sensor to determine
|
||||
// which sensor is present and return its name.
|
||||
// ICO2Sensor is then used to get readings from sensor
|
||||
|
||||
|
||||
upm::ICO2Sensor* getCO2Sensor()
|
||||
{
|
||||
upm::ICO2Sensor* cO2Sensor = NULL;
|
||||
try {
|
||||
cO2Sensor = new upm::T6713(mraa_get_sub_platform_id(FT4222_I2C_BUS));
|
||||
return cO2Sensor;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "T6713: " << e.what() << std::endl;
|
||||
}
|
||||
return cO2Sensor;
|
||||
}
|
||||
|
||||
int main ()
|
||||
{
|
||||
upm::ICO2Sensor* cO2Sensor = getCO2Sensor();
|
||||
if (cO2Sensor == NULL) {
|
||||
std::cout << "CO2 sensor not detected" << std::endl;
|
||||
return 1;
|
||||
}
|
||||
std::cout << "CO2 sensor " << cO2Sensor->getModuleName() << " detected" << std::endl;
|
||||
while (true) {
|
||||
try {
|
||||
uint16_t value = cO2Sensor->getPpm();
|
||||
std::cout << "CO2 level = " << value << " ppm" << std::endl;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << e.what() << std::endl;
|
||||
}
|
||||
sleep(1);
|
||||
}
|
||||
delete cO2Sensor;
|
||||
return 0;
|
||||
}
|
||||
|
||||
//! [Interesting]
|
82
examples/c++/humidity-sensor.cxx
Normal file
82
examples/c++/humidity-sensor.cxx
Normal file
@ -0,0 +1,82 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include "si7005.h"
|
||||
#include "bme280.h"
|
||||
|
||||
#define EDISON_I2C_BUS 1
|
||||
#define FT4222_I2C_BUS 0
|
||||
|
||||
#define EDISON_GPIO_SI7005_CS 20
|
||||
|
||||
//! [Interesting]
|
||||
// Simple example of using ILightSensor to determine
|
||||
// which sensor is present and return its name.
|
||||
// ILightSensor is then used to get readings from sensor
|
||||
|
||||
|
||||
upm::IHumiditySensor* getHumiditySensor()
|
||||
{
|
||||
upm::IHumiditySensor* humiditySensor = NULL;
|
||||
try {
|
||||
humiditySensor = new upm::BME280 (mraa_get_sub_platform_id(FT4222_I2C_BUS));
|
||||
return humiditySensor ;
|
||||
} catch (std::exception& e)
|
||||
{
|
||||
std::cerr <<"BME280: "<<e.what() << std::endl;
|
||||
}
|
||||
|
||||
try {
|
||||
humiditySensor = new upm::SI7005(EDISON_I2C_BUS, EDISON_GPIO_SI7005_CS);
|
||||
return humiditySensor;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "SI7005: " << e.what() << std::endl;
|
||||
}
|
||||
return humiditySensor;
|
||||
}
|
||||
|
||||
int main ()
|
||||
{
|
||||
upm::IHumiditySensor* humiditySensor = getHumiditySensor();
|
||||
if (humiditySensor == NULL) {
|
||||
std::cout << "Humidity sensor not detected" << std::endl;
|
||||
return 1;
|
||||
}
|
||||
std::cout << "Humidity sensor " << humiditySensor->getModuleName() << " detected" << std::endl;
|
||||
while (true) {
|
||||
try {
|
||||
int value = humiditySensor->getHumidityRelative();
|
||||
std::cout << "Humidity = " << value << "%" << std::endl;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << e.what() << std::endl;
|
||||
}
|
||||
sleep(1);
|
||||
}
|
||||
delete humiditySensor;
|
||||
return 0;
|
||||
}
|
||||
|
||||
//! [Interesting]
|
98
examples/c++/light-controller.cxx
Normal file
98
examples/c++/light-controller.cxx
Normal file
@ -0,0 +1,98 @@
|
||||
#include <stdio.h>
|
||||
#include <stdlib.h>
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <string>
|
||||
#include "lp8860.h"
|
||||
#include "ds1808lc.h"
|
||||
#include "hlg150h.h"
|
||||
|
||||
#define EDISON_I2C_BUS 1 // Edison I2C-1
|
||||
#define GPIO_SI7005_CS 20 // Edison GP12
|
||||
#define HLG150H_GPIO_RELAY 21
|
||||
#define HLG150H_GPIO_PWM 22
|
||||
#define LP8860_GPIO_PWR 45 // Edison GP45
|
||||
#define DS1808_GPIO_PWR 15 // Edison GP165
|
||||
#define DS1808_GPIO_EDISON_LIVE 36 // Edison GP14
|
||||
|
||||
//! [Interesting]
|
||||
// Simple example of using ILightController to determine
|
||||
// which controller is present and return its name.
|
||||
// ILightController is then used to get readings from sensor
|
||||
|
||||
upm::ILightController* getLightController()
|
||||
{
|
||||
upm::ILightController* lightController = NULL;
|
||||
try {
|
||||
lightController = new upm::LP8860(LP8860_GPIO_PWR, EDISON_I2C_BUS);
|
||||
return lightController;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "LP8860: " << e.what() << std::endl;
|
||||
}
|
||||
try {
|
||||
lightController = new upm::DS1808LC(DS1808_GPIO_PWR, EDISON_I2C_BUS);
|
||||
return lightController;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "DS1808LC: " << e.what() << std::endl;
|
||||
}
|
||||
try {
|
||||
lightController = new upm::HLG150H(HLG150H_GPIO_RELAY, HLG150H_GPIO_PWM);
|
||||
return lightController;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "HLG150H: " << e.what() << std::endl;
|
||||
}
|
||||
return lightController;
|
||||
}
|
||||
|
||||
|
||||
void printState(upm::ILightController *lightController)
|
||||
{
|
||||
if (lightController->isPowered())
|
||||
{
|
||||
std::cout << "Light is powered, brightness = " << lightController->getBrightness() << std::endl;
|
||||
}
|
||||
else
|
||||
{
|
||||
std::cout << "Light is not powered." << std::endl;
|
||||
}
|
||||
}
|
||||
|
||||
int main( int argc, char **argv )
|
||||
{
|
||||
int status = 0;
|
||||
// MraaUtils::setGpio(GPIO_SI7005_CS, 1);
|
||||
|
||||
upm::ILightController *lightController = getLightController();
|
||||
if (lightController != NULL)
|
||||
{
|
||||
std::cout << "Detected light controller " << lightController->getModuleName() << std::endl;
|
||||
}
|
||||
else
|
||||
{
|
||||
std::cerr << "Error. Unsupported platform." << std::endl;
|
||||
return 1;
|
||||
}
|
||||
|
||||
try {
|
||||
std::cout << "Existing state: "; printState(lightController);
|
||||
if (argc == 2)
|
||||
{
|
||||
std::string arg = argv[1];
|
||||
int brightness = ::atoi(argv[1]);
|
||||
if (brightness > 0) {
|
||||
lightController->setPowerOn();
|
||||
lightController->setBrightness(brightness);
|
||||
} else
|
||||
lightController->setPowerOff();
|
||||
}
|
||||
std::cout << "Now: ";printState(lightController);
|
||||
} catch (std::exception& e) {
|
||||
std::cout << "Error: " << e.what() << std::endl;
|
||||
status = 1;
|
||||
}
|
||||
|
||||
delete lightController;
|
||||
return status;
|
||||
}
|
||||
|
||||
|
78
examples/c++/light-sensor.cxx
Normal file
78
examples/c++/light-sensor.cxx
Normal file
@ -0,0 +1,78 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include "max44009.h"
|
||||
#include "si1132.h"
|
||||
|
||||
#define EDISON_I2C_BUS 1
|
||||
#define FT4222_I2C_BUS 0
|
||||
|
||||
//! [Interesting]
|
||||
// Simple example of using ILightSensor to determine
|
||||
// which sensor is present and return its name.
|
||||
// ILightSensor is then used to get readings from sensor
|
||||
|
||||
|
||||
upm::ILightSensor* getLightSensor()
|
||||
{
|
||||
upm::ILightSensor* lightSensor = NULL;
|
||||
try {
|
||||
lightSensor = new upm::SI1132(mraa_get_sub_platform_id(FT4222_I2C_BUS));
|
||||
return lightSensor;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "SI1132: " << e.what() << std::endl;
|
||||
}
|
||||
try {
|
||||
lightSensor = new upm::MAX44009(EDISON_I2C_BUS);
|
||||
return lightSensor;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "MAX44009: " << e.what() << std::endl;
|
||||
}
|
||||
return lightSensor;
|
||||
}
|
||||
|
||||
int main ()
|
||||
{
|
||||
upm::ILightSensor* lightSensor = getLightSensor();
|
||||
if (lightSensor == NULL) {
|
||||
std::cout << "Light sensor not detected" << std::endl;
|
||||
return 1;
|
||||
}
|
||||
std::cout << "Light sensor " << lightSensor->getModuleName() << " detected" << std::endl;
|
||||
while (true) {
|
||||
try {
|
||||
float value = lightSensor->getVisibleLux();
|
||||
std::cout << "Light level = " << value << " lux" << std::endl;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << e.what() << std::endl;
|
||||
}
|
||||
sleep(1);
|
||||
}
|
||||
delete lightSensor;
|
||||
return 0;
|
||||
}
|
||||
|
||||
//! [Interesting]
|
81
examples/c++/pressure-sensor.cxx
Normal file
81
examples/c++/pressure-sensor.cxx
Normal file
@ -0,0 +1,81 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include "bmp180.h"
|
||||
#include "bme280.h"
|
||||
|
||||
|
||||
#define EDISON_I2C_BUS 1
|
||||
#define FT4222_I2C_BUS 0
|
||||
|
||||
//! [Interesting]
|
||||
// Simple example of using ILightSensor to determine
|
||||
// which sensor is present and return its name.
|
||||
// ILightSensor is then used to get readings from sensor
|
||||
|
||||
|
||||
upm::IPressureSensor* getPressureSensor()
|
||||
{
|
||||
upm::IPressureSensor* pressureSensor = NULL;
|
||||
try {
|
||||
pressureSensor = new upm::BME280 (mraa_get_sub_platform_id(FT4222_I2C_BUS));
|
||||
return pressureSensor ;
|
||||
} catch (std::exception& e)
|
||||
{
|
||||
std::cerr <<"BME280: "<<e.what() << std::endl;
|
||||
}
|
||||
|
||||
try {
|
||||
pressureSensor = new upm::BMP180(EDISON_I2C_BUS);
|
||||
return pressureSensor;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "BMP180: " << e.what() << std::endl;
|
||||
}
|
||||
return pressureSensor;
|
||||
}
|
||||
|
||||
int main ()
|
||||
{
|
||||
upm::IPressureSensor* pressureSensor = getPressureSensor();
|
||||
if (pressureSensor == NULL) {
|
||||
std::cout << "Pressure sensor not detected" << std::endl;
|
||||
return 1;
|
||||
}
|
||||
std::cout << "Pressure sensor " << pressureSensor->getModuleName() << " detected" << std::endl;
|
||||
while (true) {
|
||||
try {
|
||||
int value = pressureSensor->getPressurePa();
|
||||
std::cout << "Pressure = " << value << " Pa" << std::endl;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << e.what() << std::endl;
|
||||
}
|
||||
sleep(1);
|
||||
}
|
||||
delete pressureSensor;
|
||||
return 0;
|
||||
}
|
||||
|
||||
//! [Interesting]
|
90
examples/c++/temperature-sensor.cxx
Normal file
90
examples/c++/temperature-sensor.cxx
Normal file
@ -0,0 +1,90 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include "si7005.h"
|
||||
#include "bmp180.h"
|
||||
#include "bme280.h"
|
||||
|
||||
#define EDISON_I2C_BUS 1
|
||||
#define FT4222_I2C_BUS 0
|
||||
|
||||
#define EDISON_GPIO_SI7005_CS 20
|
||||
|
||||
//! [Interesting]
|
||||
// Simple example of using ITemperatureSensor to determine
|
||||
// which sensor is present and return its name.
|
||||
// ITemperatureSensor is then used to get readings from sensor
|
||||
|
||||
|
||||
upm::ITemperatureSensor* getTemperatureSensor()
|
||||
{
|
||||
upm::ITemperatureSensor* temperatureSensor = NULL;
|
||||
try {
|
||||
temperatureSensor = new upm::BME280 (mraa_get_sub_platform_id(FT4222_I2C_BUS));
|
||||
return temperatureSensor;
|
||||
} catch (std::exception& e)
|
||||
{
|
||||
std::cerr <<"BME280: "<<e.what() << std::endl;
|
||||
}
|
||||
|
||||
|
||||
try {
|
||||
temperatureSensor = new upm::SI7005(EDISON_I2C_BUS, EDISON_GPIO_SI7005_CS);
|
||||
return temperatureSensor;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "SI7005: " << e.what() << std::endl;
|
||||
}
|
||||
try {
|
||||
temperatureSensor = new upm::BMP180(EDISON_I2C_BUS);
|
||||
return temperatureSensor;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << "BMP180: " << e.what() << std::endl;
|
||||
}
|
||||
return temperatureSensor;
|
||||
}
|
||||
|
||||
int main ()
|
||||
{
|
||||
upm::ITemperatureSensor* temperatureSensor = getTemperatureSensor();
|
||||
if (temperatureSensor == NULL) {
|
||||
std::cout << "Temperature sensor not detected" << std::endl;
|
||||
return 1;
|
||||
}
|
||||
std::cout << "Temperature sensor " << temperatureSensor->getModuleName() << " detected" << std::endl;
|
||||
while (true) {
|
||||
try {
|
||||
int value = temperatureSensor->getTemperatureCelcius();
|
||||
std::cout << "Temperature = " << value << "C" << std::endl;
|
||||
} catch (std::exception& e) {
|
||||
std::cerr << e.what() << std::endl;
|
||||
}
|
||||
sleep(1);
|
||||
}
|
||||
delete temperatureSensor;
|
||||
return 0;
|
||||
}
|
||||
|
||||
//! [Interesting]
|
Reference in New Issue
Block a user