mirror of
https://github.com/eclipse/upm.git
synced 2025-07-02 01:41:12 +03:00
eboled: Initial implementation
This driver supports the Sparkfun 64x48 pixel OLED Edison block: https://www.sparkfun.com/products/13035 It is based on an ssd1306, but with some modifications (custom COM pin mapping and a custom column offset). It uses SPI to communicate, and since it is an Edison Block, you don't really have any options for different bus and pin assignments. Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
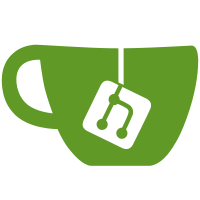
committed by
Mihai Tudor Panu
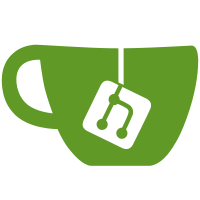
parent
9db2d57de3
commit
55e8076988
@ -132,6 +132,7 @@ add_executable (sainsmartks-example sainsmartks.cxx)
|
||||
add_executable (mpu60x0-example mpu60x0.cxx)
|
||||
add_executable (ak8975-example ak8975.cxx)
|
||||
add_executable (lsm9ds0-example lsm9ds0.cxx)
|
||||
add_executable (eboled-example eboled.cxx)
|
||||
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/hmc5883l)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grove)
|
||||
@ -371,3 +372,4 @@ target_link_libraries (sainsmartks-example i2clcd ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (mpu60x0-example mpu9150 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (ak8975-example mpu9150 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (lsm9ds0-example lsm9ds0 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (eboled-example i2clcd ${CMAKE_THREAD_LIBS_INIT})
|
||||
|
48
examples/c++/eboled.cxx
Normal file
48
examples/c++/eboled.cxx
Normal file
@ -0,0 +1,48 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
#include "eboled.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
int main(int argc, char **argv)
|
||||
{
|
||||
//! [Interesting]
|
||||
// Instantiate an Edison Block OLED using default values
|
||||
upm::EBOLED *lcd = new upm::EBOLED();
|
||||
|
||||
lcd->clear();
|
||||
lcd->setCursor(2, 0);
|
||||
lcd->write("Hello");
|
||||
lcd->setCursor(3, 5);
|
||||
lcd->write("World!");
|
||||
|
||||
cout << "Sleeping for 5 seconds..." << endl;
|
||||
sleep(5);
|
||||
|
||||
delete lcd;
|
||||
//! [Interesting]
|
||||
return 0;
|
||||
}
|
51
examples/javascript/eboled.js
Normal file
51
examples/javascript/eboled.js
Normal file
@ -0,0 +1,51 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
function exit()
|
||||
{
|
||||
lcd = null;
|
||||
lcdObj.cleanUp();
|
||||
lcdObj = null;
|
||||
process.exit(0);
|
||||
}
|
||||
|
||||
// Load i2clcd module
|
||||
var lcdObj = require('jsupm_i2clcd');
|
||||
|
||||
var lcd = new lcdObj.EBOLED();
|
||||
|
||||
lcd.clear();
|
||||
lcd.setCursor(2, 0);
|
||||
lcd.write("Hello");
|
||||
lcd.setCursor(3, 5);
|
||||
lcd.write("World!");
|
||||
|
||||
console.log("Sleeping for 5 seconds...");
|
||||
setTimeout(exit, 5000);
|
||||
|
||||
|
||||
|
40
examples/python/eboled.py
Normal file
40
examples/python/eboled.py
Normal file
@ -0,0 +1,40 @@
|
||||
#!/usr/bin/python
|
||||
# Author: Jon Trulson <jtrulson@ics.com>
|
||||
# Copyright (c) 2015 Intel Corporation.
|
||||
#
|
||||
# Permission is hereby granted, free of charge, to any person obtaining
|
||||
# a copy of this software and associated documentation files (the
|
||||
# "Software"), to deal in the Software without restriction, including
|
||||
# without limitation the rights to use, copy, modify, merge, publish,
|
||||
# distribute, sublicense, and/or sell copies of the Software, and to
|
||||
# permit persons to whom the Software is furnished to do so, subject to
|
||||
# the following conditions:
|
||||
#
|
||||
# The above copyright notice and this permission notice shall be
|
||||
# included in all copies or substantial portions of the Software.
|
||||
#
|
||||
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
||||
import time, sys
|
||||
|
||||
import pyupm_i2clcd as lcdObj
|
||||
|
||||
# setup with default values
|
||||
lcd = lcdObj.EBOLED();
|
||||
|
||||
lcd.clear();
|
||||
lcd.setCursor(2, 0);
|
||||
lcd.write("Hello");
|
||||
lcd.setCursor(3, 5);
|
||||
lcd.write("World!");
|
||||
print "Sleeping for 5 seconds..."
|
||||
time.sleep(5)
|
||||
|
||||
|
||||
|
@ -1,6 +1,6 @@
|
||||
set (libname "i2clcd")
|
||||
set (classname "lcd")
|
||||
set (libdescription "upm lcd/oled displays")
|
||||
set (module_src lcd.cxx lcm1602.cxx jhd1313m1.cxx ssd1308.cxx ssd1327.cxx sainsmartks.cxx)
|
||||
set (module_h lcd.h lcm1602.h jhd1313m1.h ssd1308.h ssd1327.h ssd.h sainsmartks.h)
|
||||
set (module_src lcd.cxx lcm1602.cxx jhd1313m1.cxx ssd1308.cxx eboled.cxx ssd1327.cxx sainsmartks.cxx)
|
||||
set (module_h lcd.h lcm1602.h jhd1313m1.h ssd1308.h eboled.h ssd1327.h ssd.h sainsmartks.h)
|
||||
upm_module_init()
|
||||
|
203
src/lcd/eboled.cxx
Normal file
203
src/lcd/eboled.cxx
Normal file
@ -0,0 +1,203 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
|
||||
#include "eboled.h"
|
||||
|
||||
using namespace upm;
|
||||
using namespace std;
|
||||
|
||||
EBOLED::EBOLED(int spi, int CD, int reset) :
|
||||
m_spi(spi), m_gpioCD(CD), m_gpioRST(reset)
|
||||
{
|
||||
m_name = "EBOLED";
|
||||
|
||||
m_gpioCD.dir(mraa::DIR_OUT);
|
||||
m_gpioRST.dir(mraa::DIR_OUT);
|
||||
|
||||
m_spi.frequency(1000000);
|
||||
|
||||
// reset the device
|
||||
m_gpioRST.write(1);
|
||||
usleep(5000);
|
||||
m_gpioRST.write(0);
|
||||
usleep(10000);
|
||||
m_gpioRST.write(1);
|
||||
|
||||
command(CMD_DISPLAYOFF);
|
||||
|
||||
command(CMD_SETDISPLAYCLOCKDIV);
|
||||
command(0x80);
|
||||
|
||||
command(CMD_SETMULTIPLEX);
|
||||
command(0x2f);
|
||||
|
||||
command(CMD_SETDISPLAYOFFSET);
|
||||
command(0x0); // no offset
|
||||
|
||||
command(CMD_SETSTARTLINE | 0x0); // line #0
|
||||
|
||||
command(CMD_CHARGEPUMP); // enable charge pump
|
||||
command(0x14);
|
||||
|
||||
command(CMD_NORMALDISPLAY);
|
||||
command(CMD_DISPLAYALLONRESUME);
|
||||
|
||||
command(CMD_SEGREMAP | 0x1); // reverse mapping (SEG0==COL127)
|
||||
command(CMD_COMSCANDEC);
|
||||
|
||||
command(CMD_SETCOMPINS); // custom COM PIN mapping
|
||||
command(0x12);
|
||||
|
||||
command(CMD_SETCONTRAST);
|
||||
command(0x8f);
|
||||
|
||||
command(CMD_SETPRECHARGE);
|
||||
command(0xf1);
|
||||
|
||||
command(CMD_SETVCOMDESELECT);
|
||||
command(0x40);
|
||||
|
||||
command(CMD_DISPLAYON);
|
||||
|
||||
|
||||
usleep(4500);
|
||||
|
||||
clear();
|
||||
setAddressingMode(PAGE);
|
||||
}
|
||||
|
||||
EBOLED::~EBOLED()
|
||||
{
|
||||
clear();
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::draw(uint8_t* bdata, int bytes)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
|
||||
setAddressingMode(HORIZONTAL);
|
||||
|
||||
command(CMD_SETCOLUMNADDRESS); // triple-byte cmd
|
||||
command(0x20); // this display has a horizontal offset of 20 columns
|
||||
command(0x20 + 0x40); // 64 columns wide
|
||||
|
||||
for (int idx = 0; idx < bytes; idx++)
|
||||
error = data(bdata[idx]);
|
||||
|
||||
// reset addressing mode
|
||||
setAddressingMode(PAGE);
|
||||
|
||||
return error;
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::write(std::string msg)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
|
||||
setAddressingMode(PAGE);
|
||||
for (std::string::size_type i = 0; i < msg.size(); ++i)
|
||||
error = writeChar(msg[i]);
|
||||
|
||||
return error;
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::setCursor(int row, int column)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
|
||||
// set page address
|
||||
error = command(CMD_SETPAGESTARTADDR | (row & 0x07));
|
||||
|
||||
// set column address
|
||||
error = command(CMD_SETLOWCOLUMN | (column & 0x0f));
|
||||
// ediblock oled starts at col 20 apparently...
|
||||
error = command(CMD_SETHIGHCOLUMN | ((column >> 4) & 0x0f) + 0x02);
|
||||
|
||||
return error;
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::clear()
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
uint8_t columnIdx, rowIdx;
|
||||
|
||||
for (rowIdx = 0; rowIdx < 8; rowIdx++)
|
||||
{
|
||||
setCursor(rowIdx, 0);
|
||||
|
||||
// clear all columns (8 * 8 pixels per char)
|
||||
for (columnIdx = 0; columnIdx < 8; columnIdx++)
|
||||
error = writeChar(' ');
|
||||
}
|
||||
|
||||
home();
|
||||
|
||||
return MRAA_SUCCESS;
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::home()
|
||||
{
|
||||
return setCursor(0, 0);
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::writeChar(uint8_t value)
|
||||
{
|
||||
mraa_result_t rv;
|
||||
|
||||
if (value < 0x20 || value > 0x7F) {
|
||||
value = 0x20; // space
|
||||
}
|
||||
|
||||
for (uint8_t idx = 0; idx < 8; idx++) {
|
||||
rv = data(BasicFont[value - 32][idx]);
|
||||
}
|
||||
|
||||
return rv;
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::setAddressingMode(displayAddressingMode mode)
|
||||
{
|
||||
mraa_result_t rv;
|
||||
|
||||
rv = command(CMD_MEMORYADDRMODE);
|
||||
rv = command(mode);
|
||||
|
||||
return rv;
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::command(uint8_t cmd)
|
||||
{
|
||||
m_gpioCD.write(0); // command mode
|
||||
m_spi.writeByte(cmd);
|
||||
return MRAA_SUCCESS;
|
||||
}
|
||||
|
||||
mraa_result_t EBOLED::data(uint8_t data)
|
||||
{
|
||||
m_gpioCD.write(1); // data mode
|
||||
m_spi.writeByte(data);
|
||||
return MRAA_SUCCESS;
|
||||
}
|
166
src/lcd/eboled.h
Normal file
166
src/lcd/eboled.h
Normal file
@ -0,0 +1,166 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <mraa/spi.hpp>
|
||||
#include <mraa/gpio.hpp>
|
||||
#include "lcd.h"
|
||||
#include "ssd.h"
|
||||
|
||||
#define EBOLED_DEFAULT_SPI_BUS 0
|
||||
#define EBOLED_DEFAULT_CD 36
|
||||
#define EBOLED_DEFAULT_RESET 48
|
||||
|
||||
namespace upm
|
||||
{
|
||||
/**
|
||||
* @library i2clcd
|
||||
* @sensor eboled
|
||||
* @comname Sparkfun Edison Block OLED Display
|
||||
* @altname ssd1306
|
||||
* @type display
|
||||
* @man sparkfun
|
||||
* @web https://www.sparkfun.com/products/13035
|
||||
* @con spi
|
||||
*
|
||||
* @brief API for EBOLED spi controlled OLED display
|
||||
*
|
||||
* The EBOLED is an ssd1306 with some modifications to work as an
|
||||
* Edison Block. It is a 64x48 pixel OLED display that connects
|
||||
* directly to an edison via it's 80-pin connector. Edison Blocks
|
||||
* are stackable modules created by Sparkfun.
|
||||
*
|
||||
* This block has some buttons on it that can be accessed using
|
||||
* standard GPIO -- this driver only concerns itself with the
|
||||
* display.
|
||||
*
|
||||
* @snippet eboled.cxx Interesting
|
||||
*/
|
||||
class EBOLED : public LCD
|
||||
{
|
||||
// SSD commands
|
||||
typedef enum {
|
||||
CMD_SETLOWCOLUMN = 0x00,
|
||||
CMD_EXTERNALVCC = 0x01,
|
||||
CMD_SWITCHCAPVCC = 0x02,
|
||||
CMD_SETHIGHCOLUMN = 0x10,
|
||||
CMD_MEMORYADDRMODE = 0x20,
|
||||
CMD_SETCOLUMNADDRESS = 0x21,
|
||||
CMD_SETPAGEADDRESS = 0x22,
|
||||
CMD_SETSTARTLINE = 0x40, // 0x40 - 0x7f
|
||||
CMD_SETCONTRAST = 0x81,
|
||||
CMD_CHARGEPUMP = 0x8d,
|
||||
CMD_SEGREMAP = 0xa0,
|
||||
CMD_DISPLAYALLONRESUME = 0xa4,
|
||||
CMD_DISPLAYALLON = 0xa5,
|
||||
CMD_NORMALDISPLAY = 0xa6,
|
||||
CMD_INVERTDISPLAY = 0xa7,
|
||||
CMD_SETMULTIPLEX = 0xa8,
|
||||
CMD_DISPLAYOFF = 0xae,
|
||||
CMD_DISPLAYON = 0xaf,
|
||||
CMD_SETPAGESTARTADDR = 0xb0, // 0xb0-0xb7
|
||||
CMD_COMSCANINC = 0xc0,
|
||||
CMD_COMSCANDEC = 0xc8,
|
||||
CMD_SETDISPLAYOFFSET = 0xd3,
|
||||
CMD_SETDISPLAYCLOCKDIV = 0xd5,
|
||||
CMD_SETPRECHARGE = 0xd9,
|
||||
CMD_SETCOMPINS = 0xda,
|
||||
CMD_SETVCOMDESELECT = 0xdb
|
||||
} SSD_CMDS_T;
|
||||
|
||||
public:
|
||||
/**
|
||||
* EBOLED Constructor. Note that you will not have any choice as
|
||||
* to the pins used, so they are all set to default values.
|
||||
*
|
||||
* @param spi spi bus to use
|
||||
* @param CD Command/Data select pin
|
||||
* @param reset reset pin
|
||||
* @param address the slave address the lcd is registered on
|
||||
*/
|
||||
EBOLED(int spi=EBOLED_DEFAULT_SPI_BUS, int CD=EBOLED_DEFAULT_CD,
|
||||
int reset=EBOLED_DEFAULT_RESET);
|
||||
|
||||
/**
|
||||
* EBOLED Destructor
|
||||
*/
|
||||
~EBOLED();
|
||||
|
||||
/**
|
||||
* Draw an image, see examples/python/make_oled_pic.py for an
|
||||
* explanation on how the pixels are mapped to bytes
|
||||
*
|
||||
* @param data the buffer to write
|
||||
* @param bytes the number of bytes to write
|
||||
* @return result of operation
|
||||
*/
|
||||
mraa_result_t draw(uint8_t* data, int bytes);
|
||||
|
||||
/**
|
||||
* Write a string to LCD
|
||||
*
|
||||
* @param msg the std::string to write to display, note only ascii
|
||||
* chars are supported
|
||||
* @return result of operation
|
||||
*/
|
||||
mraa_result_t write(std::string msg);
|
||||
|
||||
/**
|
||||
* Set cursor to a coordinate
|
||||
*
|
||||
* @param row the row to set cursor to. This device supports 6 rows.
|
||||
* @param column the column to set cursor to. This device support
|
||||
* 64 columns
|
||||
* @return result of operation
|
||||
*/
|
||||
mraa_result_t setCursor(int row, int column);
|
||||
|
||||
/**
|
||||
* Clear display
|
||||
*
|
||||
* @return result of operation
|
||||
*/
|
||||
mraa_result_t clear();
|
||||
|
||||
/**
|
||||
* Return to coordinate 0,0
|
||||
*
|
||||
* @return result of operation
|
||||
*/
|
||||
mraa_result_t home();
|
||||
|
||||
protected:
|
||||
mraa_result_t command(uint8_t cmd);
|
||||
mraa_result_t data(uint8_t data);
|
||||
mraa_result_t writeChar(uint8_t value);
|
||||
mraa_result_t setAddressingMode(displayAddressingMode mode);
|
||||
|
||||
private:
|
||||
mraa::Gpio m_gpioCD; // command(0)/data(1)
|
||||
mraa::Gpio m_gpioRST; // reset pin
|
||||
|
||||
mraa::Spi m_spi;
|
||||
};
|
||||
}
|
@ -34,6 +34,11 @@
|
||||
#include "ssd1308.h"
|
||||
%}
|
||||
|
||||
%include "eboled.h"
|
||||
%{
|
||||
#include "eboled.h"
|
||||
%}
|
||||
|
||||
%include "sainsmartks.h"
|
||||
%{
|
||||
#include "sainsmartks.h"
|
||||
|
@ -37,6 +37,11 @@
|
||||
#include "ssd1308.h"
|
||||
%}
|
||||
|
||||
%include "eboled.h"
|
||||
%{
|
||||
#include "eboled.h"
|
||||
%}
|
||||
|
||||
%include "sainsmartks.h"
|
||||
%{
|
||||
#include "sainsmartks.h"
|
||||
|
Reference in New Issue
Block a user