mirror of
https://github.com/eclipse/upm.git
synced 2025-07-02 01:41:12 +03:00
adis16448: initial implementation
Signed-off-by: Juan J Chong juanjchong@gmail.com Signed-off-by: John Van Drasek <john.r.van.drasek@intel.com>
This commit is contained in:
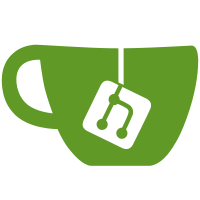
committed by
John Van Drasek
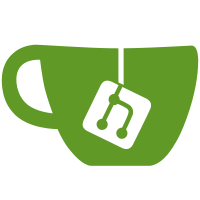
parent
49ce602808
commit
5f3b88b00a
65
examples/c++/adis16448.cxx
Normal file
65
examples/c++/adis16448.cxx
Normal file
@ -0,0 +1,65 @@
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// The MIT License (MIT)
|
||||
//
|
||||
// Submit Date: 03/09/2015
|
||||
// Author: Juan Jose Chong <juanjchong@gmail.com>
|
||||
// Copyright (c) 2015 Juan Jose Chong
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// adis16448.cxx
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
//
|
||||
// This example code runs on an Intel Edison and uses mraa to acquire data
|
||||
// from an ADIS16448. This data is then scaled and printed onto the terminal.
|
||||
//
|
||||
// This software has been tested to connect to an ADIS16448 through a level shifter
|
||||
// such as the TI TXB0104. The SPI lines (DIN, DOUT, SCLK, /CS) are all wired through
|
||||
// the level shifter and the ADIS16448 is also being powered by the Intel Edison.
|
||||
//
|
||||
// Permission is hereby granted, free of charge, to any person obtaining
|
||||
// a copy of this software and associated documentation files (the
|
||||
// "Software"), to deal in the Software without restriction, including
|
||||
// without limitation the rights to use, copy, modify, merge, publish,
|
||||
// distribute, sublicense, and/or sell copies of the Software, and to
|
||||
// permit persons to whom the Software is furnished to do so, subject to
|
||||
// the following conditions:
|
||||
//
|
||||
// The above copyright notice and this permission notice shall be
|
||||
// included in all copies or substantial portions of the Software.
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
// LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
// OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
|
||||
#include "adis16448.h"
|
||||
|
||||
int
|
||||
main(int argc, char **argv)
|
||||
{
|
||||
while(true)
|
||||
{
|
||||
upm::ADIS16448* imu = new upm::ADIS16448(0,3); //upm::ADIS16448(SPI,RST)
|
||||
|
||||
//Read the specified register, scale it, and display it on the screen
|
||||
std::cout << "XGYRO_OUT:" << imu->gyroScale(imu->regRead(XGYRO_OUT)) << std::endl;
|
||||
std::cout << "YGYRO_OUT:" << imu->gyroScale(imu->regRead(YGYRO_OUT)) << std::endl;
|
||||
std::cout << "ZGYRO_OUT:" << imu->gyroScale(imu->regRead(ZGYRO_OUT)) << std::endl;
|
||||
std::cout << " " << std::endl;
|
||||
std::cout << "XACCL_OUT:" << imu->accelScale(imu->regRead(XACCL_OUT)) << std::endl;
|
||||
std::cout << "YACCL_OUT:" << imu->accelScale(imu->regRead(YACCL_OUT)) << std::endl;
|
||||
std::cout << "ZACCL_OUT:" << imu->accelScale(imu->regRead(ZACCL_OUT)) << std::endl;
|
||||
std::cout << " " << std::endl;
|
||||
|
||||
sleep(1);
|
||||
}
|
||||
return (0);
|
||||
}
|
67
examples/javascript/adis16448.js
Normal file
67
examples/javascript/adis16448.js
Normal file
@ -0,0 +1,67 @@
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// The MIT License (MIT)
|
||||
//
|
||||
// Submit Date: 03/09/2015
|
||||
// Author: Juan Jose Chong <juanjchong@gmail.com>
|
||||
// Copyright (c) 2015 Juan Jose Chong
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// adis16448.js
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
//
|
||||
// This example code runs on an Intel Edison and uses mraa to acquire data
|
||||
// from an ADIS16448. This data is then scaled and printed onto the terminal.
|
||||
//
|
||||
// This software has been tested to connect to an ADIS16448 through a level shifter
|
||||
// such as the TI TXB0104. The SPI lines (DIN, DOUT, SCLK, /CS) are all wired through
|
||||
// the level shifter and the ADIS16448 is also being powered by the Intel Edison.
|
||||
//
|
||||
// Permission is hereby granted, free of charge, to any person obtaining
|
||||
// a copy of this software and associated documentation files (the
|
||||
// "Software"), to deal in the Software without restriction, including
|
||||
// without limitation the rights to use, copy, modify, merge, publish,
|
||||
// distribute, sublicense, and/or sell copies of the Software, and to
|
||||
// permit persons to whom the Software is furnished to do so, subject to
|
||||
// the following conditions:
|
||||
//
|
||||
// The above copyright notice and this permission notice shall be
|
||||
// included in all copies or substantial portions of the Software.
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
// LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
// OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
|
||||
//Call the ADIS16448 library
|
||||
var adis16448 = require('jsupm_adis16448');
|
||||
|
||||
//Instantiate SPI and Reset
|
||||
var imu = new adis16448.ADIS16448(0,3);
|
||||
|
||||
periodicActivity(); //Call the periodicActivity function
|
||||
|
||||
function periodicActivity()
|
||||
{
|
||||
//Read & Scale Gyro/Accel Data
|
||||
var xgyro = imu.gyroScale(imu.regRead(0x04));
|
||||
var ygyro = imu.gyroScale(imu.regRead(0x06));
|
||||
var zgyro = imu.gyroScale(imu.regRead(0x08));
|
||||
var xaccl = imu.accelScale(imu.regRead(0x0A));
|
||||
var yaccl = imu.accelScale(imu.regRead(0x0C));
|
||||
var zaccl = imu.accelScale(imu.regRead(0x0E));
|
||||
|
||||
//Display Scaled Data on the Console Log
|
||||
console.log('XGYRO: ' + xgyro);
|
||||
console.log('YGYRO: ' + ygyro);
|
||||
console.log('ZGYRO: ' + zgyro);
|
||||
console.log('XACCL: ' + xaccl);
|
||||
console.log('YACCL: ' + yaccl);
|
||||
console.log('ZACCL: ' + zaccl);
|
||||
console.log(' ');
|
||||
setTimeout(periodicActivity,200); //call the indicated function after 0.2 seconds (200 milliseconds)
|
||||
}
|
5
src/adis16448/CMakeLists.txt
Normal file
5
src/adis16448/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
||||
set (libname "adis16448")
|
||||
set (libdescription "libupm High-Precision IMU")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
223
src/adis16448/adis16448.cxx
Normal file
223
src/adis16448/adis16448.cxx
Normal file
@ -0,0 +1,223 @@
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// The MIT License (MIT)
|
||||
//
|
||||
// Submit Date: 03/09/2015
|
||||
// Author: Juan Jose Chong <juanjchong@gmail.com>
|
||||
// Copyright (c) 2015 Juan Jose Chong
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// adis16448.cxx
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
//
|
||||
// This library runs on an Intel Edison and uses mraa to acquire data
|
||||
// from an ADIS16448. This data is then scaled and printed onto the terminal.
|
||||
//
|
||||
// This software has been tested to connect to an ADIS16448 through a level shifter
|
||||
// such as the TI TXB0104. The SPI lines (DIN, DOUT, SCLK, /CS) are all wired through
|
||||
// the level shifter and the ADIS16448 is also being powered by the Intel Edison.
|
||||
//
|
||||
// Permission is hereby granted, free of charge, to any person obtaining
|
||||
// a copy of this software and associated documentation files (the
|
||||
// "Software"), to deal in the Software without restriction, including
|
||||
// without limitation the rights to use, copy, modify, merge, publish,
|
||||
// distribute, sublicense, and/or sell copies of the Software, and to
|
||||
// permit persons to whom the Software is furnished to do so, subject to
|
||||
// the following conditions:
|
||||
//
|
||||
// The above copyright notice and this permission notice shall be
|
||||
// included in all copies or substantial portions of the Software.
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
// LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
// OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
#include <iostream>
|
||||
#include <unistd.h>
|
||||
#include <stdlib.h>
|
||||
#include <functional>
|
||||
#include <string.h>
|
||||
|
||||
#include "adis16448.h"
|
||||
|
||||
using namespace upm;
|
||||
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
// Constructor with configurable CS, DR, and RST
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
// RST - Hardware reset pin
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
ADIS16448::ADIS16448(int bus, int rst)
|
||||
{
|
||||
// Configure I/O
|
||||
_rst = mraa_gpio_init(rst); //Initialize RST pin
|
||||
mraa_gpio_dir(_rst, MRAA_GPIO_IN); //Set direction as INPUT
|
||||
|
||||
// Configure SPI
|
||||
_spi = mraa_spi_init(bus);
|
||||
mraa_spi_frequency(_spi, 1000000); //Set SPI frequency to 1MHz
|
||||
mraa_spi_mode(_spi, MRAA_SPI_MODE3); //Set SPI mode/polarity
|
||||
mraa_spi_bit_per_word(_spi, 16); //Set # of bits per word
|
||||
}
|
||||
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
// Destructor - Stops SPI and Closes all GPIO used. Reports an error if
|
||||
// unable to close either properly.
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
ADIS16448::~ADIS16448()
|
||||
{
|
||||
// Close SPI bus
|
||||
mraa_result_t error;
|
||||
error = mraa_spi_stop(_spi);
|
||||
if(error != MRAA_SUCCESS)
|
||||
{
|
||||
mraa_result_print(error);
|
||||
}
|
||||
// Close GPIO
|
||||
error = mraa_gpio_close(_rst);
|
||||
if(error != MRAA_SUCCESS)
|
||||
{
|
||||
mraa_result_print(error);
|
||||
}
|
||||
}
|
||||
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
// Performs hardware reset by setting _RST pin low for 2 seconds.
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
void ADIS16448::resetDUT()
|
||||
{
|
||||
mraa_gpio_write(_rst, 0);
|
||||
usleep(100000); //Sleep for 100ms
|
||||
mraa_gpio_write(_rst, 1);
|
||||
usleep(1000000); //Sleep for 1s
|
||||
}
|
||||
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
// Sets SPI bit order, clock divider, and data mode. This function is useful
|
||||
// when there are multiple SPI devices using different settings.
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
void ADIS16448::configSPI() {
|
||||
mraa_spi_frequency(_spi, 1000000); //Set SPI frequency to 1MHz
|
||||
mraa_spi_mode(_spi, MRAA_SPI_MODE3); //Set SPI mode/polarity
|
||||
mraa_spi_bit_per_word(_spi, 16); //Set # of bits per word
|
||||
}
|
||||
|
||||
////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// Reads two bytes (one word) in two sequential registers over SPI
|
||||
////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// regAddr - register address from the lookup table in ADIS16448.h
|
||||
// return - (int) signed 16 bit 2's complement number
|
||||
////////////////////////////////////////////////////////////////////////////////////////////
|
||||
int16_t ADIS16448::regRead(uint8_t regAddr)
|
||||
{
|
||||
configSPI(); //Set up SPI (useful when multiple SPI devices present on bus)
|
||||
// Write register address to be read
|
||||
uint8_t buf[2]; //Allocate write buffer
|
||||
memset(buf, 0, sizeof(uint8_t)*2); //Initialize buffer and write 0s
|
||||
buf[1] = regAddr; //Write the user-requested register address to the buffer
|
||||
mraa_spi_write_buf(_spi, buf, 2); //Write the buffer onto the SPI port
|
||||
|
||||
usleep(20); //Delay to not violate read rate (210us)
|
||||
|
||||
// Read data from register requested
|
||||
buf[1] = 0; //Clear contents of write buffer
|
||||
uint8_t* x = mraa_spi_write_buf(_spi, buf, 2); //Write 0x0000 to SPI and read data requested above
|
||||
int16_t _dataOut = (x[1] << 8) | (x[0] & 0xFF);; //Concatenate upper and lower bytes
|
||||
|
||||
usleep(20); //delay to not violate read rate (210us)
|
||||
return(_dataOut);
|
||||
}
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
// Writes one byte of data to the specified register over SPI
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
// regAddr - register address from the lookup table
|
||||
// regData - data to be written to the register
|
||||
////////////////////////////////////////////////////////////////////////////
|
||||
void ADIS16448::regWrite(uint8_t regAddr,uint16_t regData)
|
||||
{
|
||||
configSPI();
|
||||
// Separate the 16 bit command word into two bytes
|
||||
uint16_t addr = (((regAddr & 0x7F) | 0x80) << 8); //Check that the address is 7 bits, flip the sign bit
|
||||
uint16_t lowWord = (addr | (regData & 0xFF));
|
||||
uint16_t highWord = ((addr | 0x100) | ((regData >> 8) & 0xFF));
|
||||
// Write the low byte to the SPI bus
|
||||
uint8_t lbuf[2]; //Allocate write buffer
|
||||
memset(lbuf, 0, sizeof(uint8_t)*2); //Fill low buffer with 0's
|
||||
lbuf[0] = (lowWord >> 8);
|
||||
lbuf[1] = (lowWord);
|
||||
mraa_spi_write_buf(_spi, lbuf, 2); //Write the buffer to the SPI port
|
||||
|
||||
usleep(20);
|
||||
|
||||
// Write the high byte to the SPI bus
|
||||
uint8_t hbuf[2]; //Allocate write buffer
|
||||
memset(hbuf, 0, sizeof(uint8_t)*2); //Fill high buffer with 0's
|
||||
hbuf[0] = (highWord >> 8);
|
||||
hbuf[1] = (highWord);
|
||||
mraa_spi_write_buf(_spi, hbuf, 2); //Write the buffer to the SPI port
|
||||
|
||||
usleep(20);
|
||||
|
||||
}
|
||||
/////////////////////////////////////////////////////////////////////////////////////////
|
||||
// Converts accelerometer data output from the sensorRead() function and returns
|
||||
// acceleration in g's
|
||||
/////////////////////////////////////////////////////////////////////////////////////////
|
||||
// sensorData - data output from sensorRead()
|
||||
// return - (float) signed/scaled accelerometer in G's
|
||||
/////////////////////////////////////////////////////////////////////////////////////////
|
||||
float ADIS16448::accelScale(int16_t sensorData)
|
||||
{
|
||||
float finalData = sensorData * 0.000833; // multiply by accel sensitivity (250uG/LSB)
|
||||
return finalData;
|
||||
}
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// Converts gyro data output from the sensorRead() function and returns gyro rate in deg/sec
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// sensorData - data output from sensorRead()
|
||||
// return - (float) signed/scaled gyro in degrees/sec
|
||||
/////////////////////////////////////////////////////////////////////////////////////////
|
||||
float ADIS16448::gyroScale(int16_t sensorData)
|
||||
{
|
||||
float finalData = sensorData * 0.04; //multiply by gyro sensitivity (0.005 LSB/dps)
|
||||
return finalData;
|
||||
}
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// Converts temperature data output from the sensorRead() function and returns temperature
|
||||
// in degrees Celcius
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// sensorData - data output from sensorRead()
|
||||
// return - (float) signed/scaled temperature in degrees Celcius
|
||||
/////////////////////////////////////////////////////////////////////////////////////////
|
||||
float ADIS16448::tempScale(int16_t sensorData)
|
||||
{
|
||||
float finalData = (sensorData * 0.07386) + 31; //multiply by temperature scale and add 31 to equal 0x0000
|
||||
return finalData;
|
||||
}
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// Converts barometer data output from sensorRead() function and returns pressure in bar
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// sensorData - data output from sensorRead()
|
||||
// return - (float) signed/scaled temperature in degrees Celcius
|
||||
/////////////////////////////////////////////////////////////////////////////////////////
|
||||
float ADIS16448::pressureScale(int16_t sensorData)
|
||||
{
|
||||
float finalData = (sensorData * 0.02); //multiply by gyro sensitivity (0.005 LSB/dps)
|
||||
return finalData;
|
||||
}
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// Converts magnetometer output from sensorRead() function and returns magnetic field
|
||||
// reading in Gauss
|
||||
/////////////////////////////////////////////////////////////////////////////////////////////
|
||||
// sensorData - data output from sensorRead()
|
||||
// return - (float) signed/scaled temperature in degrees Celcius
|
||||
/////////////////////////////////////////////////////////////////////////////////////////
|
||||
float ADIS16448::magnetometerScale(int16_t sensorData)
|
||||
{
|
||||
float finalData = (sensorData * 0.0001429); //multiply by sensor resolution (142.9uGa LSB/dps)
|
||||
return finalData;
|
||||
}
|
132
src/adis16448/adis16448.h
Normal file
132
src/adis16448/adis16448.h
Normal file
@ -0,0 +1,132 @@
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// The MIT License (MIT)
|
||||
//
|
||||
// Submit Date: 03/09/2015
|
||||
// Author: Juan Jose Chong <juanjchong@gmail.com>
|
||||
// Copyright (c) 2015 Juan Jose Chong
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
// adis16448.h
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
//
|
||||
// This library runs on an Intel Edison and uses mraa to acquire data
|
||||
// from an ADIS16448. This data is then scaled and printed onto the terminal.
|
||||
//
|
||||
// This software has been tested to connect to an ADIS16448 through a level shifter
|
||||
// such as the TI TXB0104. The SPI lines (DIN, DOUT, SCLK, /CS) are all wired through
|
||||
// the level shifter and the ADIS16448 is also being powered by the Intel Edison.
|
||||
//
|
||||
// Permission is hereby granted, free of charge, to any person obtaining
|
||||
// a copy of this software and associated documentation files (the
|
||||
// "Software"), to deal in the Software without restriction, including
|
||||
// without limitation the rights to use, copy, modify, merge, publish,
|
||||
// distribute, sublicense, and/or sell copies of the Software, and to
|
||||
// permit persons to whom the Software is furnished to do so, subject to
|
||||
// the following conditions:
|
||||
//
|
||||
// The above copyright notice and this permission notice shall be
|
||||
// included in all copies or substantial portions of the Software.
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
// LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
// OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
//
|
||||
//////////////////////////////////////////////////////////////////////////////////////
|
||||
#include <string>
|
||||
#include <mraa/spi.h>
|
||||
#include <mraa/gpio.h>
|
||||
|
||||
// User Register Memory Map from Table 6 of the Datasheet
|
||||
#define FLASH_CNT 0x00 //Flash memory write count
|
||||
#define XGYRO_OUT 0x04 //X-axis gyroscope output
|
||||
#define YGYRO_OUT 0x06 //Y-axis gyroscope output
|
||||
#define ZGYRO_OUT 0x08 //Z-axis gyroscope output
|
||||
#define XACCL_OUT 0x0A //X-axis accelerometer output
|
||||
#define YACCL_OUT 0x0C //Y-axis accelerometer output
|
||||
#define ZACCL_OUT 0x0E //Z-axis accelerometer output
|
||||
#define XMAGN_OUT 0X10 //X-axis magnetometer output
|
||||
#define YMAGN_OUT 0x12 //Y-axis magnetometer output
|
||||
#define ZMAGN_OUT 0x14 //Z-axis magnetometer output
|
||||
#define BARO_OUT 0x16 //Barometer pressure measurement, high word
|
||||
#define TEMP_OUT 0x18 //Temperature output
|
||||
#define XGYRO_OFF 0x1A //X-axis gyroscope bias offset factor
|
||||
#define YGYRO_OFF 0x1C //Y-axis gyroscope bias offset factor
|
||||
#define ZGYRO_OFF 0x1E //Z-axis gyroscope bias offset factor
|
||||
#define XACCL_OFF 0x20 //X-axis acceleration bias offset factor
|
||||
#define YACCL_OFF 0x22 //Y-axis acceleration bias offset factor
|
||||
#define ZACCL_OFF 0x24 //Z-axis acceleration bias offset factor
|
||||
#define XMAGN_HIC 0x26 //X-axis magnetometer, hard iron factor
|
||||
#define YMAGN_HIC 0x28 //Y-axis magnetometer, hard iron factor
|
||||
#define ZMAGN_HIC 0x2A //Z-axis magnetometer, hard iron factor
|
||||
#define XMAGN_SIC 0x2C //X-axis magnetometer, soft iron factor
|
||||
#define YMAGN_SIC 0x2E //Y-axis magnetometer, soft iron factor
|
||||
#define ZMAGN_SIC 0x30 //Z-axis magnetometer, soft iron factor
|
||||
#define GPIO_CTRL 0x32 //GPIO control
|
||||
#define MSC_CTRL 0x34 //Misc. control
|
||||
#define SMPL_PRD 0x36 //Sample clock/Decimation filter control
|
||||
#define SENS_AVG 0x38 //Digital filter control
|
||||
#define SEQ_CNT 0x3A //xMAGN_OUT and BARO_OUT counter
|
||||
#define DIAG_STAT 0x3C //System status
|
||||
#define GLOB_CMD 0x3E //System command
|
||||
#define ALM_MAG1 0x40 //Alarm 1 amplitude threshold
|
||||
#define ALM_MAG2 0x42 //Alarm 2 amplitude threshold
|
||||
#define ALM_SMPL1 0x44 //Alarm 1 sample size
|
||||
#define ALM_SMPL2 0x46 //Alarm 2 sample size
|
||||
#define ALM_CTRL 0x48 //Alarm control
|
||||
#define LOT_ID1 0x52 //Lot identification number
|
||||
#define LOT_ID2 0x54 //Lot identification number
|
||||
#define PROD_ID 0x56 //Product identifier
|
||||
#define SERIAL_NUM 0x58 //Lot-specific serial number
|
||||
|
||||
namespace upm {
|
||||
|
||||
// ADIS16448 class definition
|
||||
class ADIS16448{
|
||||
|
||||
public:
|
||||
|
||||
// Constructor with configurable HW Reset
|
||||
ADIS16448(int bus, int rst);
|
||||
|
||||
//Destructor
|
||||
~ADIS16448();
|
||||
|
||||
//Performs hardware reset by sending the specified pin low for 2 seconds
|
||||
void resetDUT();
|
||||
|
||||
//Sets SPI frequency, mode, and bits/word
|
||||
void configSPI();
|
||||
|
||||
//Read specified register and return data
|
||||
int16_t regRead(uint8_t regAddr);
|
||||
|
||||
//Write to specified register
|
||||
void regWrite(uint8_t regAddr, uint16_t regData);
|
||||
|
||||
//Scale accelerometer data
|
||||
float accelScale(int16_t sensorData);
|
||||
|
||||
//Scale gyro data
|
||||
float gyroScale(int16_t sensorData);
|
||||
|
||||
//Scale temperature data
|
||||
float tempScale(int16_t sensorData);
|
||||
|
||||
//Scale pressure data
|
||||
float pressureScale(int16_t sensorData);
|
||||
|
||||
//Scale magnetometer data
|
||||
float magnetometerScale(int16_t sensorData);
|
||||
|
||||
private:
|
||||
|
||||
mraa_spi_context _spi;
|
||||
mraa_gpio_context _rst;
|
||||
|
||||
};
|
||||
}
|
||||
|
8
src/adis16448/jsupm_adis16448.i
Normal file
8
src/adis16448/jsupm_adis16448.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module jsupm_adis16448
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "adis16448.h"
|
||||
%}
|
||||
|
||||
%include "adis16448.h"
|
8
src/adis16448/pyupm_adis16448.i
Normal file
8
src/adis16448/pyupm_adis16448.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module pyupm_adis16448
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "adis16448.h"
|
||||
%}
|
||||
|
||||
%include "adis16448.h"
|
Reference in New Issue
Block a user