mirror of
https://github.com/eclipse/upm.git
synced 2025-07-13 07:11:14 +03:00
i2clcd: improve error handling in the I2CLcd and Jhd1313m1 classes
Signed-off-by: Wouter van Verre <wouter.van.verre@intel.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
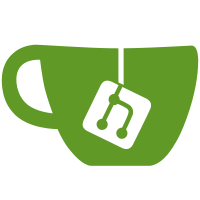
committed by
Mihai Tudor Panu
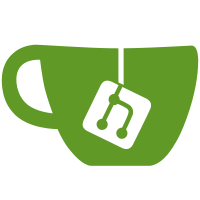
parent
dea82728af
commit
67bd592ed9
@ -26,6 +26,7 @@
|
||||
#include <unistd.h>
|
||||
|
||||
#include "i2clcd.h"
|
||||
#include "i2clcd_private.h"
|
||||
|
||||
using namespace upm;
|
||||
|
||||
@ -79,25 +80,29 @@ I2CLcd::name()
|
||||
mraa_result_t
|
||||
I2CLcd::i2cReg(mraa_i2c_context ctx, int deviceAdress, int addr, uint8_t value)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
mraa_result_t ret;
|
||||
|
||||
uint8_t data[2] = { addr, value };
|
||||
error = mraa_i2c_address(ctx, deviceAdress);
|
||||
error = mraa_i2c_write(ctx, data, 2);
|
||||
ret = mraa_i2c_address(ctx, deviceAdress);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
ret = mraa_i2c_write(ctx, data, 2);
|
||||
|
||||
return error;
|
||||
beach:
|
||||
return ret;
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
I2CLcd::i2Cmd(mraa_i2c_context ctx, uint8_t value)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
|
||||
mraa_result_t ret;
|
||||
uint8_t data[2] = { LCD_CMD, value };
|
||||
error = mraa_i2c_address(ctx, m_lcd_control_address);
|
||||
error = mraa_i2c_write(ctx, data, 2);
|
||||
|
||||
return error;
|
||||
ret = mraa_i2c_address(ctx, m_lcd_control_address);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
ret = mraa_i2c_write(ctx, data, 2);
|
||||
|
||||
beach:
|
||||
return ret;
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
|
@ -23,16 +23,16 @@
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
#include <stdexcept>
|
||||
#include <unistd.h>
|
||||
|
||||
#include "i2clcd_private.h"
|
||||
#include "jhd1313m1.h"
|
||||
|
||||
using namespace upm;
|
||||
|
||||
Jhd1313m1::Jhd1313m1(int bus, int lcdAddress, int rgbAddress) : I2CLcd(bus, lcdAddress)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
|
||||
m_rgb_address = rgbAddress;
|
||||
m_i2c_lcd_rgb = mraa_i2c_init(m_bus);
|
||||
|
||||
@ -42,22 +42,33 @@ Jhd1313m1::Jhd1313m1(int bus, int lcdAddress, int rgbAddress) : I2CLcd(bus, lcdA
|
||||
}
|
||||
|
||||
usleep(50000);
|
||||
i2Cmd(m_i2c_lcd_control, LCD_FUNCTIONSET | LCD_2LINE);
|
||||
ret = i2Cmd(m_i2c_lcd_control, LCD_FUNCTIONSET | LCD_2LINE);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
|
||||
usleep(4500);
|
||||
ret = i2Cmd(m_i2c_lcd_control, LCD_DISPLAYCONTROL | LCD_DISPLAYON);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
|
||||
ret = clear();
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
|
||||
i2Cmd(m_i2c_lcd_control, LCD_DISPLAYCONTROL | LCD_DISPLAYON);
|
||||
clear();
|
||||
usleep(4500);
|
||||
ret = i2Cmd(m_i2c_lcd_control, LCD_ENTRYMODESET | LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
|
||||
i2Cmd(m_i2c_lcd_control, LCD_ENTRYMODESET | LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT);
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0, 0);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the RGB controller");
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 1, 0);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the RGB controller");
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x08, 0xAA);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the RGB controller");
|
||||
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0, 0);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 1, 0);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x08, 0xAA);
|
||||
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x04, 255);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x03, 255);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x02, 255);
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x04, 255);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the RGB controller");
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x03, 255);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the RGB controller");
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x02, 255);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the RGB controller");
|
||||
}
|
||||
|
||||
Jhd1313m1::~Jhd1313m1()
|
||||
@ -67,15 +78,23 @@ Jhd1313m1::~Jhd1313m1()
|
||||
mraa_result_t
|
||||
Jhd1313m1::setColor(uint8_t r, uint8_t g, uint8_t b)
|
||||
{
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0, 0);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 1, 0);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x08, 0xAA);
|
||||
mraa_result_t ret;
|
||||
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x04, r);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x03, g);
|
||||
i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x02, b);
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0, 0);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 1, 0);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x08, 0xAA);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
|
||||
return MRAA_SUCCESS;
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x04, r);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x03, g);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
ret = i2cReg(m_i2c_lcd_rgb, m_rgb_address, 0x02, b);
|
||||
|
||||
beach:
|
||||
return ret;
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
@ -94,24 +113,30 @@ Jhd1313m1::scroll(bool direction)
|
||||
mraa_result_t
|
||||
Jhd1313m1::write(std::string msg)
|
||||
{
|
||||
mraa_result_t ret = MRAA_SUCCESS;
|
||||
|
||||
usleep(1000);
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
|
||||
for (std::string::size_type i = 0; i < msg.size(); ++i) {
|
||||
error = i2cData(m_i2c_lcd_control, msg[i]);
|
||||
ret = i2cData(m_i2c_lcd_control, msg[i]);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
}
|
||||
|
||||
return error;
|
||||
beach:
|
||||
return ret;
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
Jhd1313m1::setCursor(int row, int column)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
mraa_result_t ret;
|
||||
|
||||
int row_addr[] = { 0x80, 0xc0, 0x14, 0x54 };
|
||||
uint8_t offset = ((column % 16) + row_addr[row]);
|
||||
error = i2Cmd(m_i2c_lcd_control, offset);
|
||||
|
||||
return error;
|
||||
ret = i2Cmd(m_i2c_lcd_control, offset);
|
||||
|
||||
return ret;
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
|
Reference in New Issue
Block a user