mirror of
https://github.com/eclipse/upm.git
synced 2025-07-01 01:11:10 +03:00
lm35: Initial implementation
Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
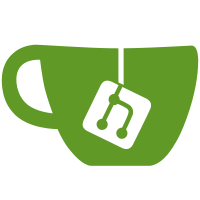
committed by
Mihai Tudor Panu
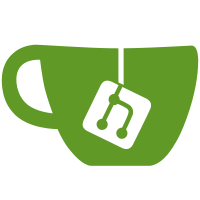
parent
a0af1c01bd
commit
7935ef1622
@ -140,6 +140,7 @@ add_executable (mg811-example mg811.cxx)
|
||||
add_executable (wheelencoder-example wheelencoder.cxx)
|
||||
add_executable (sm130-example sm130.cxx)
|
||||
add_executable (grovegprs-example grovegprs.cxx)
|
||||
add_executable (lm35-example lm35.cxx)
|
||||
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/hmc5883l)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grove)
|
||||
@ -251,6 +252,7 @@ include_directories (${PROJECT_SOURCE_DIR}/src/mg811)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/wheelencoder)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/sm130)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grovegprs)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/lm35)
|
||||
|
||||
target_link_libraries (hmc5883l-example hmc5883l ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (groveled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||
@ -392,3 +394,4 @@ target_link_libraries (mg811-example mg811 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (wheelencoder-example wheelencoder ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (sm130-example sm130 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (grovegprs-example grovegprs ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (lm35-example lm35 ${CMAKE_THREAD_LIBS_INIT})
|
||||
|
65
examples/c++/lm35.cxx
Normal file
65
examples/c++/lm35.cxx
Normal file
@ -0,0 +1,65 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
#include "lm35.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
bool shouldRun = true;
|
||||
|
||||
void sig_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
shouldRun = false;
|
||||
}
|
||||
|
||||
int main()
|
||||
{
|
||||
signal(SIGINT, sig_handler);
|
||||
|
||||
//! [Interesting]
|
||||
|
||||
// Instantiate a LM35 on analog pin A0, with a default analog
|
||||
// reference voltage of 5.0
|
||||
upm::LM35 *sensor = new upm::LM35(0);
|
||||
|
||||
// Every half second, sample the sensor and output the temperature
|
||||
|
||||
while (shouldRun)
|
||||
{
|
||||
cout << "Temperature: " << sensor->getTemperature() << " C" << endl;
|
||||
|
||||
usleep(500000);
|
||||
}
|
||||
|
||||
//! [Interesting]
|
||||
|
||||
cout << "Exiting" << endl;
|
||||
|
||||
delete sensor;
|
||||
return 0;
|
||||
}
|
51
examples/javascript/lm35.js
Normal file
51
examples/javascript/lm35.js
Normal file
@ -0,0 +1,51 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
|
||||
var sensorObj = require('jsupm_lm35');
|
||||
|
||||
// Instantiate a LM35 on analog pin A0, with a default analog
|
||||
// reference voltage of 5.0
|
||||
var sensor = new sensorObj.LM35(0);
|
||||
|
||||
// Every half second, sample the sensor and output the temperature
|
||||
|
||||
setInterval(function()
|
||||
{
|
||||
console.log("Temperature: " + sensor.getTemperature() + " C");
|
||||
}, 500);
|
||||
|
||||
// exit on ^C
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
sensor = null;
|
||||
sensorObj.cleanUp();
|
||||
sensorObj = null;
|
||||
console.log("Exiting.");
|
||||
process.exit(0);
|
||||
});
|
||||
|
49
examples/python/lm35.py
Normal file
49
examples/python/lm35.py
Normal file
@ -0,0 +1,49 @@
|
||||
#!/usr/bin/python
|
||||
# Author: Jon Trulson <jtrulson@ics.com>
|
||||
# Copyright (c) 2015 Intel Corporation.
|
||||
#
|
||||
# Permission is hereby granted, free of charge, to any person obtaining
|
||||
# a copy of this software and associated documentation files (the
|
||||
# "Software"), to deal in the Software without restriction, including
|
||||
# without limitation the rights to use, copy, modify, merge, publish,
|
||||
# distribute, sublicense, and/or sell copies of the Software, and to
|
||||
# permit persons to whom the Software is furnished to do so, subject to
|
||||
# the following conditions:
|
||||
#
|
||||
# The above copyright notice and this permission notice shall be
|
||||
# included in all copies or substantial portions of the Software.
|
||||
#
|
||||
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
||||
import time, sys, signal, atexit
|
||||
import pyupm_lm35 as sensorObj
|
||||
|
||||
# Instantiate a LM35 on analog pin A0, with a default analog
|
||||
# reference voltage of 5.0
|
||||
sensor = sensorObj.LM35(0)
|
||||
|
||||
## Exit handlers ##
|
||||
# This function stops python from printing a stacktrace when you hit control-C
|
||||
def SIGINTHandler(signum, frame):
|
||||
raise SystemExit
|
||||
|
||||
# This function lets you run code on exit
|
||||
def exitHandler():
|
||||
print "Exiting"
|
||||
sys.exit(0)
|
||||
|
||||
# Register exit handlers
|
||||
atexit.register(exitHandler)
|
||||
signal.signal(signal.SIGINT, SIGINTHandler)
|
||||
|
||||
# Every half second, sample the sensor and output the temperature
|
||||
|
||||
while (1):
|
||||
print "Temperature:", sensor.getTemperature(), "C"
|
||||
time.sleep(.5)
|
5
src/lm35/CMakeLists.txt
Normal file
5
src/lm35/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
||||
set (libname "lm35")
|
||||
set (libdescription "upm DFRobot LM35 temperature sensor")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
8
src/lm35/javaupm_lm35.i
Normal file
8
src/lm35/javaupm_lm35.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module javaupm_lm35
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "lm35.h"
|
||||
%}
|
||||
|
||||
%include "lm35.h"
|
8
src/lm35/jsupm_lm35.i
Normal file
8
src/lm35/jsupm_lm35.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module jsupm_lm35
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "lm35.h"
|
||||
%}
|
||||
|
||||
%include "lm35.h"
|
51
src/lm35/lm35.cxx
Normal file
51
src/lm35/lm35.cxx
Normal file
@ -0,0 +1,51 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
#include "lm35.h"
|
||||
|
||||
using namespace std;
|
||||
using namespace upm;
|
||||
|
||||
LM35::LM35(int pin, float aref) :
|
||||
m_aio(pin)
|
||||
{
|
||||
m_aRes = m_aio.getBit();
|
||||
m_aref = aref;
|
||||
}
|
||||
|
||||
LM35::~LM35()
|
||||
{
|
||||
}
|
||||
|
||||
float LM35::getTemperature()
|
||||
{
|
||||
int val = m_aio.read();
|
||||
|
||||
// convert to mV
|
||||
float temp = (float(val) * (m_aref / float(1 << m_aRes))) * 1000.0;
|
||||
|
||||
// 10mV/degree C
|
||||
return(temp / 10.0);
|
||||
}
|
93
src/lm35/lm35.h
Normal file
93
src/lm35/lm35.h
Normal file
@ -0,0 +1,93 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <iostream>
|
||||
#include <string>
|
||||
#include <mraa/aio.hpp>
|
||||
|
||||
namespace upm {
|
||||
/**
|
||||
* @brief DFRobot LM35 Linear Temperature Sensor
|
||||
* @defgroup lm35 libupm-lm35
|
||||
* @ingroup dfrobot analog temp
|
||||
*/
|
||||
|
||||
/**
|
||||
* @library lm35
|
||||
* @sensor lm35
|
||||
* @comname DFRobot LM35 Linear Temperature Sensor
|
||||
* @altname LM35
|
||||
* @type temp
|
||||
* @man dfrobot
|
||||
* @web http://www.dfrobot.com/index.php?route=product/product&product_id=76
|
||||
* @con analog
|
||||
*
|
||||
* @brief API for the DFRobot LM35 Linear Temperature Sensor
|
||||
*
|
||||
* This sensor returns an analog voltage proportional to the
|
||||
* temperature of the ambient environment.
|
||||
*
|
||||
* It has a range of 2C to 150C.
|
||||
*
|
||||
* This driver was developed using the DFRobot LM35 Linear
|
||||
* Temperature Sensor
|
||||
*
|
||||
* @snippet lm35.cxx Interesting
|
||||
*/
|
||||
|
||||
class LM35 {
|
||||
public:
|
||||
|
||||
/**
|
||||
* LM35 constructor
|
||||
*
|
||||
* @param pin Analog pin to use
|
||||
* @param aref Analog reference voltage; default is 5.0 V
|
||||
*/
|
||||
LM35(int pin, float aref=5.0);
|
||||
|
||||
/**
|
||||
* LM35 destructor
|
||||
*/
|
||||
~LM35();
|
||||
|
||||
/**
|
||||
* Returns the temperature in degrees Celcius
|
||||
*
|
||||
* @return The Temperature in degrees Celcius
|
||||
*/
|
||||
float getTemperature();
|
||||
|
||||
protected:
|
||||
mraa::Aio m_aio;
|
||||
|
||||
private:
|
||||
float m_aref;
|
||||
// ADC resolution
|
||||
int m_aRes;
|
||||
};
|
||||
}
|
||||
|
||||
|
9
src/lm35/pyupm_lm35.i
Normal file
9
src/lm35/pyupm_lm35.i
Normal file
@ -0,0 +1,9 @@
|
||||
%module pyupm_lm35
|
||||
%include "../upm.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%include "lm35.h"
|
||||
%{
|
||||
#include "lm35.h"
|
||||
%}
|
Reference in New Issue
Block a user