mirror of
https://github.com/eclipse/upm.git
synced 2025-07-01 01:11:10 +03:00
apds9930: Initial implementation
Signed-off-by: Lay, Kuan Loon <kuan.loon.lay@intel.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
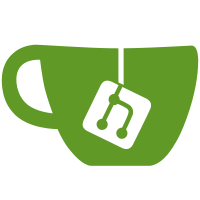
committed by
Mihai Tudor Panu
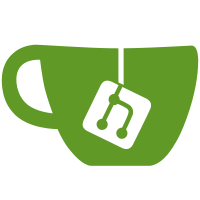
parent
8cec2ad473
commit
828a9ce7d8
@ -240,6 +240,7 @@ if (MODBUS_FOUND)
|
||||
endif()
|
||||
add_example (hdxxvxta)
|
||||
add_example (rhusb)
|
||||
add_example (apds9930)
|
||||
|
||||
# These are special cases where you specify example binary, source file and module(s)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src)
|
||||
|
63
examples/c++/apds9930.cxx
Normal file
63
examples/c++/apds9930.cxx
Normal file
@ -0,0 +1,63 @@
|
||||
/*
|
||||
* Author: Lay, Kuan Loon <kuan.loon.lay@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
#include "apds9930.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
int shouldRun = true;
|
||||
|
||||
void
|
||||
sig_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
shouldRun = false;
|
||||
}
|
||||
|
||||
int
|
||||
main()
|
||||
{
|
||||
signal(SIGINT, sig_handler);
|
||||
//! [Interesting]
|
||||
// Instantiate a Digital Proximity and Ambient Light sensor on iio device 4
|
||||
upm::APDS9930* light_proximity = new upm::APDS9930(4);
|
||||
|
||||
while (shouldRun) {
|
||||
float lux = light_proximity->getAmbient();
|
||||
cout << "Luminance value is " << lux << endl;
|
||||
float proximity = light_proximity->getProximity();
|
||||
cout << "Proximity value is " << proximity << endl;
|
||||
sleep(1);
|
||||
}
|
||||
//! [Interesting]
|
||||
|
||||
cout << "Exiting" << endl;
|
||||
|
||||
delete light_proximity;
|
||||
|
||||
return 0;
|
||||
}
|
5
src/apds9930/CMakeLists.txt
Executable file
5
src/apds9930/CMakeLists.txt
Executable file
@ -0,0 +1,5 @@
|
||||
set (libname "apds9930")
|
||||
set (libdescription "upm apds9930 sensor module")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
61
src/apds9930/apds9930.cxx
Normal file
61
src/apds9930/apds9930.cxx
Normal file
@ -0,0 +1,61 @@
|
||||
/*
|
||||
* Author: Lay, Kuan Loon <kuan.loon.lay@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
#include <string>
|
||||
#include <stdexcept>
|
||||
|
||||
#include "apds9930.h"
|
||||
|
||||
using namespace upm;
|
||||
|
||||
APDS9930::APDS9930(int device)
|
||||
{
|
||||
if (!(m_iio = mraa_iio_init(device))) {
|
||||
throw std::invalid_argument(std::string(__FUNCTION__) +
|
||||
": mraa_iio_init() failed, invalid device?");
|
||||
return;
|
||||
}
|
||||
}
|
||||
|
||||
APDS9930::~APDS9930()
|
||||
{
|
||||
// mraa_iio_stop(m_iio);
|
||||
}
|
||||
|
||||
int
|
||||
APDS9930::getAmbient()
|
||||
{
|
||||
int iio_value = 0;
|
||||
mraa_iio_read_integer(m_iio, "in_illuminance_input", &iio_value);
|
||||
return iio_value;
|
||||
}
|
||||
|
||||
int
|
||||
APDS9930::getProximity()
|
||||
{
|
||||
int iio_value = 0;
|
||||
mraa_iio_read_integer(m_iio, "in_proximity_raw", &iio_value);
|
||||
return iio_value;
|
||||
}
|
82
src/apds9930/apds9930.h
Normal file
82
src/apds9930/apds9930.h
Normal file
@ -0,0 +1,82 @@
|
||||
/*
|
||||
* Author: Lay, Kuan Loon <kuan.loon.lay@intel.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <mraa/iio.h>
|
||||
|
||||
namespace upm
|
||||
{
|
||||
/**
|
||||
* @brief APDS9930 Digital Proximity and Ambient Light Sensor library
|
||||
* @defgroup apds9930 libupm-apds9930
|
||||
* @ingroup Avago Technologies iio i2c proximity and ambient light sensor
|
||||
*/
|
||||
|
||||
/**
|
||||
* @library apds9930
|
||||
* @sensor apds9930
|
||||
* @comname APDS9930 Digital Proximity and Ambient Light Sensor
|
||||
* @type light proximity
|
||||
* @man Avago Technologies
|
||||
* @con iio i2c
|
||||
*
|
||||
* @brief APDS9930 Digital Proximity and Ambient Light Sensor
|
||||
*
|
||||
* This sensor provides digital ambient light sensing (ALS),
|
||||
* IR LED and a complete proximity detection system.
|
||||
*
|
||||
* @snippet apds9930.cxx Interesting
|
||||
*/
|
||||
|
||||
class APDS9930
|
||||
{
|
||||
public:
|
||||
/**
|
||||
* APDS-9930 digital proximity and ambient light sensor constructor
|
||||
*
|
||||
* @param iio device number
|
||||
*/
|
||||
APDS9930(int device);
|
||||
/**
|
||||
* APDS9930 destructor
|
||||
*/
|
||||
~APDS9930();
|
||||
/**
|
||||
* Gets the ambient luminance value from the sensor
|
||||
*
|
||||
* @return Ambient Luminance value
|
||||
*/
|
||||
int getAmbient();
|
||||
/**
|
||||
* Gets the proximity value from the sensor
|
||||
*
|
||||
* @return Proximity value
|
||||
*/
|
||||
int getProximity();
|
||||
|
||||
private:
|
||||
mraa_iio_context m_iio;
|
||||
};
|
||||
}
|
14
src/apds9930/javaupm_apds9930.i
Executable file
14
src/apds9930/javaupm_apds9930.i
Executable file
@ -0,0 +1,14 @@
|
||||
%module javaupm_apds9930
|
||||
%include "../upm.i"
|
||||
%include "stdint.i"
|
||||
%include "typemaps.i"
|
||||
|
||||
%feature("director") IsrCallback;
|
||||
|
||||
%ignore generic_callback_isr;
|
||||
%include "../IsrCallback.h"
|
||||
|
||||
%{
|
||||
#include "apds9930.h"
|
||||
%}
|
||||
%include "apds9930.h"
|
8
src/apds9930/jsupm_apds9930.i
Executable file
8
src/apds9930/jsupm_apds9930.i
Executable file
@ -0,0 +1,8 @@
|
||||
%module jsupm_apds9930
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "apds9930.h"
|
||||
%}
|
||||
|
||||
%include "apds9930.h"
|
9
src/apds9930/pyupm_apds9930.i
Executable file
9
src/apds9930/pyupm_apds9930.i
Executable file
@ -0,0 +1,9 @@
|
||||
%module pyupm_apds9930
|
||||
%include "../upm.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%include "apds9930.h"
|
||||
%{
|
||||
#include "apds9930.h"
|
||||
%}
|
Reference in New Issue
Block a user