mirror of
https://github.com/eclipse/upm.git
synced 2025-07-03 02:11:15 +03:00
lsm303: python example and js modification for lsm303 accelerometer/compass
Signed-off-by: Zion Orent <zorent@ics.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
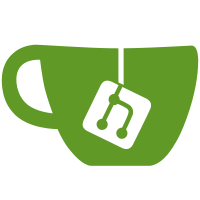
committed by
Mihai Tudor Panu
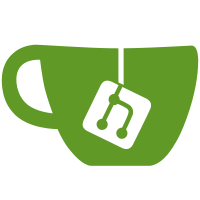
parent
f9d0e4621b
commit
94fe1174c6
@ -1,6 +1,5 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
/*global */
|
||||
/*
|
||||
* Author: Zion Orent <zorent@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
@ -30,16 +29,17 @@ var accelrCompassSensor = require('jsupm_lsm303');
|
||||
// Instantiate LSM303 compass on I2C
|
||||
var myAccelrCompass = new accelrCompassSensor.LSM303(0);
|
||||
|
||||
setInterval(function()
|
||||
var successFail, coords, outputStr, accel;
|
||||
var myInterval = setInterval(function()
|
||||
{
|
||||
// Load coordinates into LSM303 object
|
||||
var successFail = myAccelrCompass.getCoordinates();
|
||||
successFail = myAccelrCompass.getCoordinates();
|
||||
// in XYZ order. The sensor returns XZY,
|
||||
// but the driver compensates and makes it XYZ
|
||||
var coords = myAccelrCompass.getRawCoorData();
|
||||
coords = myAccelrCompass.getRawCoorData();
|
||||
|
||||
// Print out the X, Y, and Z coordinate data using two different methods
|
||||
var outputStr = "coor: rX " + coords.getitem(0)
|
||||
outputStr = "coor: rX " + coords.getitem(0)
|
||||
+ " - rY " + coords.getitem(1)
|
||||
+ " - rZ " + coords.getitem(2);
|
||||
console.log(outputStr);
|
||||
@ -53,7 +53,7 @@ setInterval(function()
|
||||
|
||||
// Get the acceleration
|
||||
myAccelrCompass.getAcceleration();
|
||||
var accel = myAccelrCompass.getRawAccelData();
|
||||
accel = myAccelrCompass.getRawAccelData();
|
||||
// Print out the X, Y, and Z acceleration data using two different methods
|
||||
outputStr = "acc: rX " + accel.getitem(0)
|
||||
+ " - rY " + accel.getitem(1)
|
||||
@ -65,3 +65,14 @@ setInterval(function()
|
||||
console.log(outputStr);
|
||||
console.log(" ");
|
||||
}, 1000);
|
||||
|
||||
// Print message when exiting
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
clearInterval(myInterval);
|
||||
myAccelrCompass = null;
|
||||
accelrCompassSensor.cleanUp();
|
||||
accelrCompassSensor = null;
|
||||
console.log("Exiting");
|
||||
process.exit(0);
|
||||
});
|
||||
|
@ -1,6 +1,7 @@
|
||||
#!/usr/bin/env python
|
||||
#!/usr/bin/python
|
||||
|
||||
# Author: Brendan Le Foll <brendan.le.foll@intel.com>
|
||||
# Contributions: Zion Orent <zorent@ics.com>
|
||||
# Copyright (c) 2014 Intel Corporation.
|
||||
#
|
||||
# Permission is hereby granted, free of charge, to any person obtaining
|
||||
@ -22,8 +23,65 @@
|
||||
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE
|
||||
|
||||
import time, sys, signal, atexit
|
||||
import pyupm_lsm303 as lsm303
|
||||
|
||||
x = lsm303.LSM303(0)
|
||||
x.getCoordinates()
|
||||
x.getHeading()
|
||||
# Instantiate LSM303 compass on I2C
|
||||
myAccelrCompass = lsm303.LSM303(0)
|
||||
|
||||
|
||||
## Exit handlers ##
|
||||
# This stops python from printing a stacktrace when you hit control-C
|
||||
def SIGINTHandler(signum, frame):
|
||||
raise SystemExit
|
||||
|
||||
# This lets you run code on exit,
|
||||
# including functions from myAccelrCompass
|
||||
def exitHandler():
|
||||
print "Exiting"
|
||||
sys.exit(0)
|
||||
|
||||
# Register exit handlers
|
||||
atexit.register(exitHandler)
|
||||
signal.signal(signal.SIGINT, SIGINTHandler)
|
||||
|
||||
|
||||
while(1):
|
||||
# Load coordinates into LSM303 object
|
||||
successFail = myAccelrCompass.getCoordinates()
|
||||
# in XYZ order. The sensor returns XZY,
|
||||
# but the driver compensates and makes it XYZ
|
||||
coords = myAccelrCompass.getRawCoorData()
|
||||
|
||||
# Print out the X, Y, and Z coordinate data
|
||||
# using two different methods
|
||||
outputStr = "coor: rX {0} - rY {1} - rZ {2}".format(
|
||||
coords.__getitem__(0), coords.__getitem__(1),
|
||||
coords.__getitem__(2))
|
||||
print outputStr
|
||||
|
||||
outputStr = "coor: gX {0} - gY {1} - gZ {2}".format(
|
||||
myAccelrCompass.getCoorX(), myAccelrCompass.getCoorY(),
|
||||
myAccelrCompass.getCoorZ())
|
||||
print outputStr
|
||||
|
||||
# Get and print out the heading
|
||||
print "heading:", myAccelrCompass.getHeading()
|
||||
|
||||
# Get the acceleration
|
||||
myAccelrCompass.getAcceleration();
|
||||
accel = myAccelrCompass.getRawAccelData();
|
||||
|
||||
# Print out the X, Y, and Z acceleration data
|
||||
# using two different methods
|
||||
outputStr = "acc: rX {0} - rY {1} - Z {2}".format(
|
||||
accel.__getitem__(0), accel.__getitem__(1), accel.__getitem__(2))
|
||||
print outputStr
|
||||
|
||||
outputStr = "acc: gX {0} - gY {1} - gZ {2}".format(
|
||||
myAccelrCompass.getAccelX(), myAccelrCompass.getAccelY(),
|
||||
myAccelrCompass.getAccelZ())
|
||||
print outputStr
|
||||
|
||||
print " "
|
||||
time.sleep(1)
|
||||
|
@ -1,8 +1,15 @@
|
||||
%module pyupm_lsm303
|
||||
%include "../upm.i"
|
||||
%include "../carrays_int16_t.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
// Adding this typemap because SWIG is converting int16 into a short by default
|
||||
// This forces SWIG to convert it correctly
|
||||
%typemap(out) int16_t* {
|
||||
resultobj = SWIG_NewPointerObj(SWIG_as_voidptr(result), SWIGTYPE_p_int16Array, 0 | 0 );
|
||||
}
|
||||
|
||||
%include "lsm303.h"
|
||||
%{
|
||||
#include "lsm303.h"
|
||||
|
Reference in New Issue
Block a user