mirror of
https://github.com/eclipse/upm.git
synced 2025-07-02 01:41:12 +03:00
bh1749: Add examples for all languages
* Examples for C/C++/Python/Java/JavaScript * Add Java example to CMakeLists Signed-off-by: Assam Boudjelthia <assam.boudjelthia@fi.rohmeurope.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
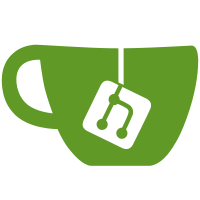
committed by
Mihai Tudor Panu
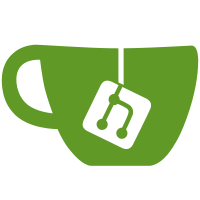
parent
5f2557b159
commit
9ef90ccb81
69
examples/c++/bh1749.cxx
Executable file
69
examples/c++/bh1749.cxx
Executable file
@ -0,0 +1,69 @@
|
||||
/*
|
||||
* The MIT License (MIT)
|
||||
*
|
||||
* Author: Assam Boudjelthia
|
||||
* Copyright (c) 2018 Rohm Semiconductor.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
* this software and associated documentation files (the "Software"), to deal in
|
||||
* the Software without restriction, including without limitation the rights to
|
||||
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
* the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
* subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
#include <unistd.h>
|
||||
#include <stdexcept>
|
||||
#include <signal.h>
|
||||
#include <vector>
|
||||
#include "bh1749.hpp"
|
||||
#include "upm_utilities.h"
|
||||
|
||||
bool isStopped;
|
||||
upm::BH1749 dev;
|
||||
|
||||
void signal_int_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
isStopped = true;
|
||||
}
|
||||
|
||||
void PrintData(void *args)
|
||||
{
|
||||
std::vector<uint16_t> result = dev.GetMeasurements();
|
||||
std::cout << "R: " << result.at(0) <<
|
||||
", G: " << result.at(1) <<
|
||||
", B: " << result.at(2) <<
|
||||
", IR: " << result.at(3) <<
|
||||
", G2: " << result.at(4) << std::endl;
|
||||
dev.ResetInterrupt();
|
||||
}
|
||||
|
||||
int main(int argc, char **argv)
|
||||
{
|
||||
signal(SIGINT, signal_int_handler);
|
||||
dev.SoftReset();
|
||||
dev.SensorInit(INT_JUDGE_1, MEAS_240MS, RGB_GAIN_1X, IR_GAIN_1X, RED);
|
||||
dev.SetThresholdHigh(511);
|
||||
std::cout << "Installing ISR" << std::endl;
|
||||
dev.InstallISR(MRAA_GPIO_EDGE_FALLING, 33, &PrintData, NULL);
|
||||
dev.EnableInterrupt();
|
||||
dev.Enable();
|
||||
|
||||
while(!isStopped) {
|
||||
upm_delay_ms(1000);
|
||||
}
|
||||
|
||||
return 0;
|
||||
}
|
73
examples/c/bh1749.c
Executable file
73
examples/c/bh1749.c
Executable file
@ -0,0 +1,73 @@
|
||||
/*
|
||||
* The MIT License (MIT)
|
||||
*
|
||||
* Author: Assam Boudjelthia
|
||||
* Copyright (c) 2018 Rohm Semiconductor.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
* this software and associated documentation files (the "Software"), to deal in
|
||||
* the Software without restriction, including without limitation the rights to
|
||||
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
* the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
* subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <stdio.h>
|
||||
#include <signal.h>
|
||||
#include "bh1749.h"
|
||||
#include "upm_utilities.h"
|
||||
|
||||
bool isStopped;
|
||||
#define SENSOR_ADDR 0x39
|
||||
#define I2C_BUS 0
|
||||
|
||||
void signal_int_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
isStopped = true;
|
||||
}
|
||||
|
||||
void print_data(void *dev)
|
||||
{
|
||||
uint16_t result[5];
|
||||
bh1749_get_measurements((bh1749_context)dev, result);
|
||||
printf("R: %d, G: %d, B: %d, IR: %d, G2: %d\n", result[0],
|
||||
result[1], result[2], result[3], result[4]);
|
||||
bh1749_reset_interrupt(dev);
|
||||
}
|
||||
|
||||
int main(int argc, char **argv)
|
||||
{
|
||||
signal(SIGINT, signal_int_handler);
|
||||
|
||||
bh1749_context dev = bh1749_init(I2C_BUS, SENSOR_ADDR);
|
||||
if (!dev) {
|
||||
printf("bh1749_init() failed.\n");
|
||||
return -1;
|
||||
}
|
||||
|
||||
bh1749_soft_reset(dev);
|
||||
bh1749_sensor_init(dev, INT_JUDGE_1, MEAS_240MS, RGB_GAIN_1X, IR_GAIN_1X, RED);
|
||||
bh1749_set_threshold_high(dev, 511);
|
||||
bh1749_enable_interrupt(dev);
|
||||
printf("Installing ISR\n");
|
||||
bh1749_install_isr(dev, MRAA_GPIO_EDGE_FALLING, 33, &print_data, (void *)dev);
|
||||
bh1749_enable(dev);
|
||||
|
||||
while(!isStopped) {
|
||||
upm_delay_ms(1000);
|
||||
}
|
||||
|
||||
bh1749_close(dev);
|
||||
return 0;
|
||||
}
|
51
examples/java/BH1749_Example.java
Executable file
51
examples/java/BH1749_Example.java
Executable file
@ -0,0 +1,51 @@
|
||||
/*
|
||||
* The MIT License (MIT)
|
||||
*
|
||||
* Author: Assam Boudjelthia
|
||||
* Copyright (c) 2018 Rohm Semiconductor.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
* this software and associated documentation files (the "Software"), to deal in
|
||||
* the Software without restriction, including without limitation the rights to
|
||||
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
* the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
* subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
public class BH1749_Example {
|
||||
|
||||
public static void main(String[] args) throws InterruptedException {
|
||||
int sampleCounter = 10;
|
||||
long waitTime;
|
||||
upm_bh1749.BH1749 bh1749;
|
||||
upm_bh1749.floatVector result;
|
||||
|
||||
bh1749 = new upm_bh1749.BH1749();
|
||||
bh1749.SensorInit(upm_bh1749.OPERATING_MODES.INT_JUDGE_1,
|
||||
upm_bh1749.MEAS_TIMES.MEAS_240MS, upm_bh1749.RGB_GAINS.RGB_GAIN_1X,
|
||||
upm_bh1749.IR_GAINS.IR_GAIN_1X, upm_bh1749.INT_SOURCES.RED);
|
||||
bh1749.SetThresholdHigh(511);
|
||||
bh1749.Enable();
|
||||
|
||||
waitTime = bh1749.GetMeasurementTime();
|
||||
System.out.println("Color readings");
|
||||
while (sampleCounter-- > 0) {
|
||||
result = bh1749.GetMeasurements();
|
||||
System.out.println("R: " + result.get(0) + ", G: " + result.get(1) +
|
||||
", B: " + result.get(2) + ", IR: " + result.get(3) +
|
||||
", G2: " + result.get(4));
|
||||
|
||||
Thread.sleep((long) waitTime);
|
||||
}
|
||||
}
|
||||
}
|
@ -74,6 +74,7 @@ add_example(Adxl345_Example adxl345)
|
||||
add_example(AM2315_Example am2315)
|
||||
add_example(APA102_Example apa102)
|
||||
add_example(Apds9002_Example apds9002)
|
||||
add_example(BH1749_Example bh1749)
|
||||
add_example(BH1750_Example bh1750)
|
||||
add_example(BH1792_Example bh1792)
|
||||
add_example(BISS0001_Example biss0001)
|
||||
|
57
examples/javascript/bh1749.js
Executable file
57
examples/javascript/bh1749.js
Executable file
@ -0,0 +1,57 @@
|
||||
/*
|
||||
* The MIT License (MIT)
|
||||
*
|
||||
* Author: Assam Boudjelthia
|
||||
* Copyright (c) 2018 Rohm Semiconductor.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
* this software and associated documentation files (the "Software"), to deal in
|
||||
* the Software without restriction, including without limitation the rights to
|
||||
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
* the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
* subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in all
|
||||
* copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
var bh1749 = require("jsupm_bh1749");
|
||||
|
||||
var bh1749_sensor = new bh1749.BH1749();
|
||||
|
||||
bh1749_sensor.SensorInit(
|
||||
bh1749.INT_JUDGE_1,
|
||||
bh1749.MEAS_240MS,
|
||||
bh1749.RGB_GAIN_1X,
|
||||
bh1749.IR_GAIN_1X,
|
||||
bh1749.RED);
|
||||
bh1749_sensor.SetThresholdHigh(511);
|
||||
bh1749_sensor.Enable();
|
||||
|
||||
var waitTime = bh1749_sensor.GetMeasurementTime();
|
||||
var counter = 10;
|
||||
console.log("Color readings: ");
|
||||
var interval = setInterval(function() {
|
||||
data = bh1749_sensor.GetMeasurements();
|
||||
console.log(
|
||||
"R: " + data.get(0) + ", G: " + data.get(1) + ", B: " + data.get(2) +
|
||||
", IR: " + data.get(3) + ", G2: " + data.get(4)
|
||||
);
|
||||
counter--;
|
||||
if (counter == 0) {
|
||||
clearInterval(interval);
|
||||
}
|
||||
}, waitTime);
|
||||
|
||||
process.on("SIGINT", function() {
|
||||
clearInterval(interval);
|
||||
console.log("Exiting...");
|
||||
process.exit(0);
|
||||
});
|
60
examples/python/bh1749.py
Executable file
60
examples/python/bh1749.py
Executable file
@ -0,0 +1,60 @@
|
||||
# The MIT License (MIT)
|
||||
#
|
||||
# Author: Assam Boudjelthia
|
||||
# Copyright (c) 2018 Rohm Semiconductor.
|
||||
#
|
||||
# Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
# this software and associated documentation files (the "Software"), to deal in
|
||||
# the Software without restriction, including without limitation the rights to
|
||||
# use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
|
||||
# the Software, and to permit persons to whom the Software is furnished to do so,
|
||||
# subject to the following conditions:
|
||||
#
|
||||
# The above copyright notice and this permission notice shall be included in all
|
||||
# copies or substantial portions of the Software.
|
||||
#
|
||||
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
|
||||
# FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
|
||||
# COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
|
||||
# IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
||||
# CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
||||
import time, sys, signal, atexit
|
||||
from upm import pyupm_bh1749
|
||||
|
||||
|
||||
def main():
|
||||
bh1749_sensor = pyupm_bh1749.BH1749()
|
||||
|
||||
bh1749_sensor.SensorInit(pyupm_bh1749.INT_JUDGE_1,
|
||||
pyupm_bh1749.MEAS_240MS,
|
||||
pyupm_bh1749.RGB_GAIN_1X,
|
||||
pyupm_bh1749.IR_GAIN_1X,
|
||||
pyupm_bh1749.RED)
|
||||
bh1749_sensor.SetThresholdHigh(511)
|
||||
|
||||
# Prevent stack printing on CTRL^C
|
||||
def SIGINTHandler(signum, frame):
|
||||
raise SystemExit
|
||||
|
||||
def exitHandler():
|
||||
print("Exiting")
|
||||
sys.exit(0)
|
||||
|
||||
atexit.register(exitHandler)
|
||||
signal.signal(signal.SIGINT, SIGINTHandler)
|
||||
|
||||
sampleCounter = 10
|
||||
waitTime = bh1749_sensor.GetMeasurementTime()
|
||||
print("Color readings: ")
|
||||
while sampleCounter > 0:
|
||||
[r, g, b, ir, g2] = bh1749_sensor.GetMeasurements()
|
||||
print ("R: %d, y: %d, G: %d, IR: %d, G2: %d" % (r, g, b, ir, g2))
|
||||
|
||||
time.sleep(waitTime / 1000.0)
|
||||
sampleCounter -= 1
|
||||
|
||||
|
||||
if __name__ == '__main__':
|
||||
main()
|
Reference in New Issue
Block a user