mirror of
https://github.com/eclipse/upm.git
synced 2025-07-05 19:31:15 +03:00
ds1808lc: Initial Implementation Maxim DS1808 based LED lighting controller
DS1808 is a general purpose potentiometer but this implementation is limited to controlling brightness of custom LED lighting hardware. Ideally there should be a DS1808 module used by a seperate lighting module. Signed-off-by: Henry Bruce <henry.bruce@intel.com> Signed-off-by: Abhishek Malik <abhishek.malik@intel.com>
This commit is contained in:
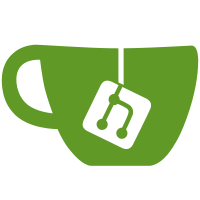
committed by
Abhishek Malik
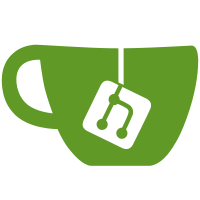
parent
63066bcc0b
commit
d183c9574d
6
src/ds1808lc/CMakeLists.txt
Normal file
6
src/ds1808lc/CMakeLists.txt
Normal file
@ -0,0 +1,6 @@
|
||||
include_directories(..)
|
||||
set (libname "ds1808lc")
|
||||
set (libdescription “DS1808 lighting controller”)
|
||||
set (module_src ${libname}.cxx mraa-utils.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
139
src/ds1808lc/ds1808lc.cxx
Normal file
139
src/ds1808lc/ds1808lc.cxx
Normal file
@ -0,0 +1,139 @@
|
||||
#include <iostream>
|
||||
#include <unistd.h>
|
||||
#include <string.h>
|
||||
#include <cmath>
|
||||
#include "ds1808lc.h"
|
||||
#include "mraa-utils.h"
|
||||
|
||||
#define DS1808_I2C_ADDR 0x28
|
||||
#define DS1808_POT2_OFFSET 0x40
|
||||
#define DS1808_MAX_POT_VALUE 0x20
|
||||
#define DS1808_LOW_VALUE 32 // Lowest pot value that the eye can differentiate from 0
|
||||
#define DS1808_HIGH_VALUE 51 // Highest pot value that the eye can differentiate from full
|
||||
|
||||
|
||||
namespace upm {
|
||||
DS1808LC::DS1808LC(int gpioPower, int i2cBus)
|
||||
{
|
||||
mraa_set_log_level(7);
|
||||
pinPower = gpioPower;
|
||||
i2c = new mraa::I2c(i2cBus);
|
||||
status = i2c->address(DS1808_I2C_ADDR);
|
||||
getBrightness();
|
||||
}
|
||||
|
||||
DS1808LC::~DS1808LC()
|
||||
{
|
||||
}
|
||||
|
||||
|
||||
bool DS1808LC::isConfigured()
|
||||
{
|
||||
return status == mraa::SUCCESS;
|
||||
}
|
||||
|
||||
bool DS1808LC::isPowered()
|
||||
{
|
||||
return static_cast<bool>(MraaUtils::getGpio(pinPower));
|
||||
}
|
||||
|
||||
void DS1808LC::setPowerOn()
|
||||
{
|
||||
if (!isPowered())
|
||||
{
|
||||
MraaUtils::setGpio(pinPower, 1);
|
||||
setBrightness(0);
|
||||
}
|
||||
}
|
||||
|
||||
void DS1808LC::setPowerOff()
|
||||
{
|
||||
MraaUtils::setGpio(pinPower, 0);
|
||||
}
|
||||
|
||||
|
||||
int DS1808LC::getBrightness()
|
||||
{
|
||||
uint8_t values[2];
|
||||
|
||||
if (i2c->read(values, 2) == 2) {
|
||||
return getPercentBrightness(values[0], values[1]);
|
||||
}
|
||||
else
|
||||
UPM_THROW("i2c read error");
|
||||
}
|
||||
|
||||
|
||||
void DS1808LC::setBrightness(int dutyPercent)
|
||||
{
|
||||
uint8_t values[2];
|
||||
values[0] = getPot1Value(dutyPercent);
|
||||
values[1] = getPot2Value(dutyPercent);
|
||||
status = i2c->write(values, 2);
|
||||
if (status != mraa::SUCCESS)
|
||||
UPM_THROW("i2c write error");
|
||||
}
|
||||
|
||||
//
|
||||
// Helper Function
|
||||
//
|
||||
|
||||
uint8_t DS1808LC::getPot1Value(int dutyPercent) {
|
||||
uint8_t result = 0;
|
||||
int scaledResistance = getScaledResistance(dutyPercent);
|
||||
|
||||
if (scaledResistance > DS1808_MAX_POT_VALUE)
|
||||
result = DS1808_MAX_POT_VALUE;
|
||||
else {
|
||||
result = scaledResistance;
|
||||
}
|
||||
|
||||
return result;
|
||||
}
|
||||
|
||||
uint8_t DS1808LC::getPot2Value(int dutyPercent) {
|
||||
uint8_t result = 0;
|
||||
int scaledResistance = getScaledResistance(dutyPercent);
|
||||
|
||||
if (scaledResistance <= DS1808_MAX_POT_VALUE)
|
||||
result = 0;
|
||||
else {
|
||||
result = scaledResistance - DS1808_MAX_POT_VALUE;
|
||||
}
|
||||
|
||||
if (result > DS1808_MAX_POT_VALUE)
|
||||
result = DS1808_MAX_POT_VALUE;
|
||||
|
||||
return result | DS1808_POT2_OFFSET;
|
||||
}
|
||||
|
||||
int DS1808LC::getPercentBrightness(uint8_t val1, uint8_t val2) {
|
||||
val2 = val2 & (~DS1808_POT2_OFFSET);
|
||||
int scaledResistance = val1 + val2;
|
||||
int percent;
|
||||
|
||||
if (scaledResistance < DS1808_LOW_VALUE)
|
||||
percent = 100;
|
||||
else if (scaledResistance > DS1808_HIGH_VALUE)
|
||||
percent = 0;
|
||||
else
|
||||
percent = 100 - (((scaledResistance - DS1808_LOW_VALUE) * 100) / (DS1808_HIGH_VALUE - DS1808_LOW_VALUE));
|
||||
return percent;
|
||||
}
|
||||
|
||||
int DS1808LC::getScaledResistance(int dutyPercent) {
|
||||
int scaledResistance;
|
||||
|
||||
if (dutyPercent == 0)
|
||||
scaledResistance = 2 * DS1808_MAX_POT_VALUE;
|
||||
else if (dutyPercent == 100)
|
||||
scaledResistance = 0;
|
||||
else
|
||||
scaledResistance = (((100 - dutyPercent) * (DS1808_HIGH_VALUE - DS1808_LOW_VALUE)) / 100) + DS1808_LOW_VALUE;
|
||||
return scaledResistance;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
|
||||
|
81
src/ds1808lc/ds1808lc.h
Normal file
81
src/ds1808lc/ds1808lc.h
Normal file
@ -0,0 +1,81 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include "upm/iLightController.h"
|
||||
#include "mraa/i2c.hpp"
|
||||
|
||||
namespace upm
|
||||
{
|
||||
/**
|
||||
* @brief DS1808LC lighting controller library
|
||||
* @defgroup DS1808LC libupm-ds1808lc
|
||||
* @ingroup ds1808lc i2c maxim light ilightcontroller
|
||||
*/
|
||||
|
||||
/**
|
||||
* @brief API for DS1808 Dual Log Digital Potentiometer as a Light Controller
|
||||
*
|
||||
* The Maxim Integrated
|
||||
* [DS1808](http://www.maximintegrated.com/en/products/analog/data-converters/digital-potentiometers/DS1808.html)
|
||||
* Dual Log Digital Potentiometer
|
||||
*
|
||||
*
|
||||
* @library ds1808lc
|
||||
* @sensor ds1808lc
|
||||
* @comname Maxim DS1808 as lighting controller
|
||||
* @altname DS1808LC
|
||||
* @type light
|
||||
* @man maxim
|
||||
* @con i2c
|
||||
* @if ilightcontroller
|
||||
*/
|
||||
class DS1808LC : public upm::ILightController
|
||||
{
|
||||
public:
|
||||
DS1808LC(int gpioPower, int i2cBus);
|
||||
~DS1808LC();
|
||||
|
||||
protected:
|
||||
bool isConfigured();
|
||||
const char* getModuleName() { return "ds1808lc"; }
|
||||
bool isPowered();
|
||||
void setPowerOn();
|
||||
void setPowerOff();
|
||||
int getBrightness();
|
||||
void setBrightness(int dutyPercent);
|
||||
|
||||
private:
|
||||
int getPercentBrightness(uint8_t val1, uint8_t val2);
|
||||
uint8_t getPot1Value(int dutyPercent);
|
||||
uint8_t getPot2Value(int dutyPercent);
|
||||
int getScaledResistance(int dutyPercent);
|
||||
|
||||
mraa::Result status;
|
||||
mraa::I2c* i2c;
|
||||
int pinPower;
|
||||
};
|
||||
|
||||
|
||||
}
|
||||
|
8
src/ds1808lc/jsupm_ds1808lc.i
Normal file
8
src/ds1808lc/jsupm_ds1808lc.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module jsupm_tubelight
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "tubelight.h"
|
||||
%}
|
||||
|
||||
%include "tubelight.h"
|
72
src/ds1808lc/mraa-utils.cxx
Normal file
72
src/ds1808lc/mraa-utils.cxx
Normal file
@ -0,0 +1,72 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include "mraa-utils.h"
|
||||
#include "mraa/gpio.hpp"
|
||||
|
||||
#define UPM_THROW(msg) throw std::runtime_error(std::string(__FUNCTION__) + ": " + (msg))
|
||||
|
||||
void MraaUtils::setGpio(int pin, int level)
|
||||
{
|
||||
/*
|
||||
mraa_result_t status = MRAA_ERROR_NO_RESOURCES;
|
||||
mraa_gpio_context gpio = mraa_gpio_init(pin);
|
||||
if (gpio != NULL)
|
||||
{
|
||||
mraa_gpio_dir(gpio, MRAA_GPIO_OUT);
|
||||
status = mraa_gpio_write(gpio, level);
|
||||
mraa_gpio_close(gpio);
|
||||
}
|
||||
return status;
|
||||
*/
|
||||
mraa::Gpio gpio(pin);
|
||||
gpio.dir(mraa::DIR_OUT);
|
||||
if (gpio.write(level) != mraa::SUCCESS)
|
||||
UPM_THROW("gpio write failed");
|
||||
}
|
||||
|
||||
|
||||
int MraaUtils::getGpio(int pin)
|
||||
{
|
||||
/*
|
||||
mraa_result_t status = MRAA_ERROR_NO_RESOURCES;
|
||||
mraa_gpio_context gpio = mraa_gpio_init(pin);
|
||||
if (gpio != NULL)
|
||||
{
|
||||
status = mraa_gpio_dir(gpio, MRAA_GPIO_IN);
|
||||
int value = mraa_gpio_read(gpio);
|
||||
if (value != -1)
|
||||
*level = value;
|
||||
else
|
||||
status = MRAA_ERROR_NO_RESOURCES;
|
||||
mraa_gpio_close(gpio);
|
||||
}
|
||||
return status;
|
||||
*/
|
||||
mraa::Gpio gpio(pin);
|
||||
gpio.dir(mraa::DIR_IN);
|
||||
return gpio.read();
|
||||
}
|
||||
|
||||
|
37
src/ds1808lc/mraa-utils.h
Normal file
37
src/ds1808lc/mraa-utils.h
Normal file
@ -0,0 +1,37 @@
|
||||
/*
|
||||
* Author: Henry Bruce <henry.bruce@intel.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "mraa/types.h"
|
||||
|
||||
class __attribute__ ((visibility("hidden"))) MraaUtils
|
||||
{
|
||||
public:
|
||||
static void setGpio(int pin, int level);
|
||||
static int getGpio(int pin);
|
||||
};
|
||||
|
||||
|
||||
|
9
src/ds1808lc/pyupm_ds1808lc.i
Normal file
9
src/ds1808lc/pyupm_ds1808lc.i
Normal file
@ -0,0 +1,9 @@
|
||||
%module pyupm_tubelight
|
||||
%include "../upm.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%include "tubelight.h"
|
||||
%{
|
||||
#include "tubelight.h"
|
||||
%}
|
Reference in New Issue
Block a user