mirror of
https://github.com/eclipse/upm.git
synced 2025-07-06 03:41:13 +03:00
rotaryencoder: Initial implementation
This module implements supoort for the Grove Rotary Encoder, though it should function with any Rotary Encoder utilizing two GPIOs. Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Zion Orent <zorent@ics.com> Signed-off-by: John Van Drasek <john.r.van.drasek@intel.com>
This commit is contained in:
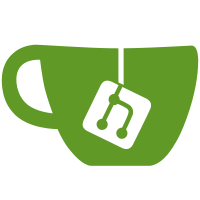
committed by
John Van Drasek
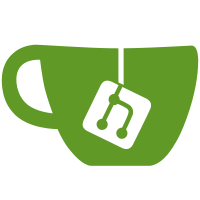
parent
6135e932e4
commit
d307376abc
@ -76,6 +76,7 @@ add_executable (rfr359f-example rfr359f.cxx)
|
||||
add_executable (biss0001-example biss0001.cxx)
|
||||
add_executable (my9221-example my9221.cxx)
|
||||
add_executable (grove_mcfled-example grove_mcfled.cxx)
|
||||
add_executable (rotaryencoder-example rotaryencoder.cxx)
|
||||
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/hmc5883l)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grove)
|
||||
@ -138,6 +139,7 @@ include_directories (${PROJECT_SOURCE_DIR}/src/grovespeaker)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/rfr359f)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/biss0001)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/my9221)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/rotaryencoder)
|
||||
|
||||
target_link_libraries (hmc5883l-example hmc5883l ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (groveled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||
@ -217,3 +219,4 @@ target_link_libraries (rfr359f-example rfr359f ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (biss0001-example biss0001 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (my9221-example my9221 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (grove_mcfled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (rotaryencoder-example rotaryencoder ${CMAKE_THREAD_LIBS_INIT})
|
||||
|
43
examples/javascript/rotaryencoder.js
Normal file
43
examples/javascript/rotaryencoder.js
Normal file
@ -0,0 +1,43 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
/*
|
||||
* Author: Zion Orent <zorent@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
var rotaryEncoder = require("jsupm_rotaryencoder");
|
||||
|
||||
// Instantiate a Grove Rotary Encoder, using signal pins D2 and D3
|
||||
var myRotaryEncoder = new rotaryEncoder.RotaryEncoder(2, 3);
|
||||
|
||||
var myInterval = setInterval(function()
|
||||
{
|
||||
console.log("Position: " + myRotaryEncoder.position());
|
||||
}, 100);
|
||||
|
||||
// When exiting: clear interval and print message
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
clearInterval(myInterval);
|
||||
console.log("Exiting...");
|
||||
process.exit(0);
|
||||
});
|
61
examples/rotaryencoder.cxx
Normal file
61
examples/rotaryencoder.cxx
Normal file
@ -0,0 +1,61 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
#include "rotaryencoder.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
int shouldRun = true;
|
||||
|
||||
void sig_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
shouldRun = false;
|
||||
}
|
||||
|
||||
|
||||
int main()
|
||||
{
|
||||
signal(SIGINT, sig_handler);
|
||||
|
||||
//! [Interesting]
|
||||
// Instantiate a Grove Rotary Encoder, using signal pins D2 and D3
|
||||
upm::RotaryEncoder* rotaryencoder = new upm::RotaryEncoder(2, 3);
|
||||
|
||||
while (shouldRun)
|
||||
{
|
||||
cout << "Position: " << rotaryencoder->position() << endl;
|
||||
usleep(100000);
|
||||
}
|
||||
|
||||
//! [Interesting]
|
||||
|
||||
cout << "Exiting..." << endl;
|
||||
|
||||
delete rotaryencoder;
|
||||
return 0;
|
||||
}
|
5
src/rotaryencoder/CMakeLists.txt
Normal file
5
src/rotaryencoder/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
||||
set (libname "rotaryencoder")
|
||||
set (libdescription "upm grove rotary encoder module")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
8
src/rotaryencoder/jsupm_rotaryencoder.i
Normal file
8
src/rotaryencoder/jsupm_rotaryencoder.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module jsupm_rotaryencoder
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "rotaryencoder.h"
|
||||
%}
|
||||
|
||||
%include "rotaryencoder.h"
|
9
src/rotaryencoder/pyupm_rotaryencoder.i
Normal file
9
src/rotaryencoder/pyupm_rotaryencoder.i
Normal file
@ -0,0 +1,9 @@
|
||||
%module pyupm_rotaryencoder
|
||||
%include "../upm.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%include "rotaryencoder.h"
|
||||
%{
|
||||
#include "rotaryencoder.h"
|
||||
%}
|
91
src/rotaryencoder/rotaryencoder.cxx
Normal file
91
src/rotaryencoder/rotaryencoder.cxx
Normal file
@ -0,0 +1,91 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
|
||||
#include "rotaryencoder.h"
|
||||
|
||||
using namespace upm;
|
||||
using namespace std;
|
||||
|
||||
RotaryEncoder::RotaryEncoder(int pinA, int pinB)
|
||||
{
|
||||
if ( !(m_gpioA = mraa_gpio_init(pinA)) )
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_gpio_init() failed" << endl;
|
||||
return;
|
||||
}
|
||||
|
||||
mraa_gpio_dir(m_gpioA, MRAA_GPIO_IN);
|
||||
|
||||
if ( !(m_gpioB = mraa_gpio_init(pinB)) )
|
||||
{
|
||||
cerr << __FUNCTION__ << ": mraa_gpio_init() failed" << endl;
|
||||
return;
|
||||
}
|
||||
|
||||
mraa_gpio_dir(m_gpioB, MRAA_GPIO_IN);
|
||||
|
||||
m_position = 0;
|
||||
|
||||
// setup the ISR
|
||||
|
||||
// We would prefer to use MRAA_GPIO_EDGE_BOTH for better resolution,
|
||||
// but that does not appear to be supported
|
||||
mraa_gpio_isr(m_gpioA, MRAA_GPIO_EDGE_RISING,
|
||||
&signalAISR, this);
|
||||
}
|
||||
|
||||
RotaryEncoder::~RotaryEncoder()
|
||||
{
|
||||
mraa_gpio_isr_exit(m_gpioA);
|
||||
|
||||
mraa_gpio_close(m_gpioA);
|
||||
mraa_gpio_close(m_gpioB);
|
||||
}
|
||||
|
||||
void RotaryEncoder::initPosition(int count)
|
||||
{
|
||||
m_position = count;
|
||||
}
|
||||
|
||||
int RotaryEncoder::position()
|
||||
{
|
||||
return m_position;
|
||||
}
|
||||
|
||||
void RotaryEncoder::signalAISR(void *ctx)
|
||||
{
|
||||
upm::RotaryEncoder *This = (upm::RotaryEncoder *)ctx;
|
||||
|
||||
if (mraa_gpio_read(This->m_gpioA))
|
||||
{
|
||||
if (mraa_gpio_read(This->m_gpioB))
|
||||
This->m_position++; // CW
|
||||
else
|
||||
This->m_position--; // CCW
|
||||
}
|
||||
}
|
||||
|
||||
|
91
src/rotaryencoder/rotaryencoder.h
Normal file
91
src/rotaryencoder/rotaryencoder.h
Normal file
@ -0,0 +1,91 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <stdint.h>
|
||||
#include <sys/time.h>
|
||||
#include <mraa/gpio.h>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
* @brief C++ API for the Grove Rotary Encoder
|
||||
*
|
||||
* UPM module for the Grove Rotary Encoder. A Rotary Encoder
|
||||
* encodes a rotation signal into electronic pulses that can be used
|
||||
* to measure rotation and direction. It is useful in cases where a
|
||||
* rotary knob is required, but using a potentiometer is not
|
||||
* desireable. A rotary encoder can turn a full 360 degrees+
|
||||
* without a stop and does not place a resistive load on the
|
||||
* circuit, as is the case with a potentiometer.
|
||||
*
|
||||
* This module maintains a position that is incremented or
|
||||
* decremented according to the rotation on the encoder.
|
||||
*
|
||||
* @ingroup gpio
|
||||
* @snippet rotaryencoder.cxx Interesting
|
||||
*/
|
||||
class RotaryEncoder {
|
||||
public:
|
||||
/**
|
||||
* RotaryEncoder constructor
|
||||
*
|
||||
* @param pinA digital pin to use for signal A
|
||||
* @param pinB digital pin to use for signal B
|
||||
*/
|
||||
RotaryEncoder(int pinA, int pinB);
|
||||
/**
|
||||
* RotaryEncoder Destructor
|
||||
*/
|
||||
~RotaryEncoder();
|
||||
|
||||
/**
|
||||
* Reset the position to a given number, default is 0.
|
||||
*
|
||||
* @param count integer to initialize the position to
|
||||
*/
|
||||
void initPosition(int count=0);
|
||||
|
||||
/**
|
||||
* Get the position value
|
||||
*
|
||||
*/
|
||||
int position();
|
||||
|
||||
/**
|
||||
* ISR for signal A
|
||||
*
|
||||
* @param ctx user context for the ISR (*this pointer)
|
||||
*/
|
||||
static void signalAISR(void *ctx);
|
||||
|
||||
private:
|
||||
volatile int m_position;
|
||||
mraa_gpio_context m_gpioA;
|
||||
mraa_gpio_context m_gpioB;
|
||||
};
|
||||
}
|
||||
|
||||
|
Reference in New Issue
Block a user