mirror of
https://github.com/eclipse/upm.git
synced 2025-07-03 02:11:15 +03:00
grovespeaker: Initial implementation of Grove Speaker
Signed-off-by: Zion Orent <zorent@ics.com> Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Sarah Knepper <sarah.knepper@intel.com>
This commit is contained in:
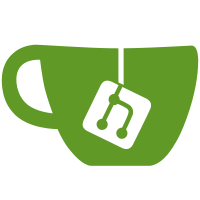
committed by
Sarah Knepper
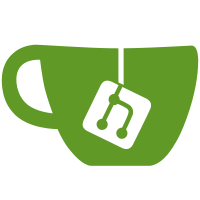
parent
1ac1760691
commit
db1ca3d08a
@ -71,6 +71,7 @@ add_executable (yg1006-example yg1006.cxx)
|
||||
add_executable (wt5001-example wt5001.cxx)
|
||||
add_executable (ppd42ns-example ppd42ns.cxx)
|
||||
add_executable (mq303a-example mq303a.cxx)
|
||||
add_executable (grovespeaker-example grovespeaker.cxx)
|
||||
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/hmc5883l)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grove)
|
||||
@ -129,6 +130,7 @@ include_directories (${PROJECT_SOURCE_DIR}/src/yg1006)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/wt5001)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/ppd42ns)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/mq303a)
|
||||
include_directories (${PROJECT_SOURCE_DIR}/src/grovespeaker)
|
||||
|
||||
target_link_libraries (hmc5883l-example hmc5883l ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (groveled-example grove ${CMAKE_THREAD_LIBS_INIT})
|
||||
@ -203,3 +205,4 @@ target_link_libraries (yg1006-example yg1006 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (wt5001-example wt5001 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (ppd42ns-example ppd42ns ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (mq303a-example mq303a ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (grovespeaker-example grovespeaker ${CMAKE_THREAD_LIBS_INIT})
|
||||
|
49
examples/grovespeaker.cxx
Normal file
49
examples/grovespeaker.cxx
Normal file
@ -0,0 +1,49 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
#include "grovespeaker.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
int main ()
|
||||
{
|
||||
//! [Interesting]
|
||||
// Instantiate a Grove Speaker on digital pin D2
|
||||
upm::GroveSpeaker* speaker = new upm::GroveSpeaker(2);
|
||||
|
||||
// Play all 7 of the lowest notes
|
||||
speaker->playAll();
|
||||
|
||||
// Play a medium C-sharp
|
||||
speaker->playSound('c', true, "med");
|
||||
//! [Interesting]
|
||||
|
||||
cout << "Exiting" << endl;
|
||||
|
||||
delete speaker;
|
||||
return 0;
|
||||
}
|
44
examples/javascript/grovespeaker.js
Normal file
44
examples/javascript/grovespeaker.js
Normal file
@ -0,0 +1,44 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
/*global */
|
||||
/*
|
||||
* Author: Zion Orent <zorent@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
//Load Grove Speaker module
|
||||
var groveSpeaker = require('jsupm_grovespeaker');
|
||||
// Instantiate a Grove Speaker on digital pin D2
|
||||
var mySpeaker = new groveSpeaker.GroveSpeaker(2);
|
||||
|
||||
// Play all 7 of the lowest notes
|
||||
mySpeaker.playAll();
|
||||
|
||||
// Play a medium C-sharp
|
||||
mySpeaker.playSound('c', true, "med");
|
||||
|
||||
// Print message when exiting
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
console.log("Exiting...");
|
||||
process.exit(0);
|
||||
});
|
5
src/grovespeaker/CMakeLists.txt
Normal file
5
src/grovespeaker/CMakeLists.txt
Normal file
@ -0,0 +1,5 @@
|
||||
set (libname "grovespeaker")
|
||||
set (libdescription "upm grovespeaker speaker module")
|
||||
set (module_src ${libname}.cxx)
|
||||
set (module_h ${libname}.h)
|
||||
upm_module_init()
|
145
src/grovespeaker/grovespeaker.cxx
Normal file
145
src/grovespeaker/grovespeaker.cxx
Normal file
@ -0,0 +1,145 @@
|
||||
/*
|
||||
* Author: Zion Orent <sorent@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
|
||||
#include "grovespeaker.h"
|
||||
|
||||
using namespace upm;
|
||||
|
||||
GroveSpeaker::GroveSpeaker(int pin)
|
||||
{
|
||||
m_gpio = mraa_gpio_init(pin);
|
||||
mraa_gpio_dir(m_gpio, MRAA_GPIO_OUT);
|
||||
m_note_list['a'] = storeNote(1136, 1073, 568, 536, 284, 268);
|
||||
m_note_list['b'] = storeNote(1012, 0, 506, 0, 253, 0);
|
||||
m_note_list['c'] = storeNote(1911, 1804, 956, 902, 478, 451);
|
||||
m_note_list['d'] = storeNote(1703, 1607, 851, 804, 426, 402);
|
||||
m_note_list['e'] = storeNote(1517, 0, 758, 0, 379, 0);
|
||||
m_note_list['f'] = storeNote(1432, 1351, 716, 676, 358, 338);
|
||||
m_note_list['g'] = storeNote(1276, 1204, 638, 602, 319, 301);
|
||||
}
|
||||
|
||||
GroveSpeaker::~GroveSpeaker()
|
||||
{
|
||||
mraa_gpio_close(m_gpio);
|
||||
}
|
||||
|
||||
NoteData GroveSpeaker::storeNote(int noteDelayLow, int noteDelayLowSharp,
|
||||
int noteDelayMed, int noteDelayMedSharp,
|
||||
int noteDelayHigh, int noteDelayHighSharp)
|
||||
{
|
||||
NoteData note;
|
||||
note.delayTimeLow = noteDelayLow;
|
||||
note.delayTimeLowSharp = noteDelayLowSharp;
|
||||
note.delayTimeMed = noteDelayMed;
|
||||
note.delayTimeMedSharp = noteDelayMedSharp;
|
||||
note.delayTimeHigh = noteDelayHigh;
|
||||
note.delayTimeHighSharp = noteDelayHighSharp;
|
||||
return note;
|
||||
}
|
||||
|
||||
void GroveSpeaker::playAll()
|
||||
{
|
||||
playSound('c', false, "low");
|
||||
usleep(200000);
|
||||
playSound('d', false, "low");
|
||||
usleep(200000);
|
||||
playSound('e', false, "low");
|
||||
usleep(200000);
|
||||
playSound('f', false, "low");
|
||||
usleep(200000);
|
||||
playSound('g', false, "low");
|
||||
usleep(500000);
|
||||
playSound('a', false, "low");
|
||||
usleep(500000);
|
||||
playSound('b', false, "low");
|
||||
usleep(500000);
|
||||
}
|
||||
|
||||
void GroveSpeaker::playSound(char letter, bool sharp, std::string vocalWeight)
|
||||
{
|
||||
std::map<char, NoteData>::iterator it = m_note_list.find(letter);
|
||||
if(it == m_note_list.end())
|
||||
{
|
||||
std::cout << "The key " << letter << " doesn't exist." << std::endl;
|
||||
return;
|
||||
}
|
||||
NoteData nd = it->second;
|
||||
int delayTime;
|
||||
if (sharp)
|
||||
{
|
||||
if (vocalWeight.compare("low") == 0)
|
||||
delayTime = nd.delayTimeLowSharp;
|
||||
else if (vocalWeight.compare("med") == 0)
|
||||
delayTime = nd.delayTimeMedSharp;
|
||||
else if (vocalWeight.compare("high") == 0)
|
||||
delayTime = nd.delayTimeHighSharp;
|
||||
else
|
||||
{
|
||||
std::cout << "Correct voice weight values are low, med, or high"
|
||||
<< std::endl;
|
||||
return;
|
||||
}
|
||||
}
|
||||
else
|
||||
{
|
||||
if (vocalWeight.compare("low") == 0)
|
||||
delayTime = nd.delayTimeLow;
|
||||
else if (vocalWeight.compare("med") == 0)
|
||||
delayTime = nd.delayTimeMed;
|
||||
else if (vocalWeight.compare("high") == 0)
|
||||
delayTime = nd.delayTimeHigh;
|
||||
else
|
||||
{
|
||||
std::cout << "Correct voice weight values are low, med, or high"
|
||||
<< std::endl;
|
||||
return;
|
||||
}
|
||||
}
|
||||
// If delayTime is zero, that means you tried to choose a sharp note
|
||||
// for a note that has no sharp
|
||||
if (sharp && !delayTime)
|
||||
{
|
||||
std::cout << "The key " << letter << " doesn't have a sharp note."
|
||||
<< std::endl;
|
||||
return;
|
||||
}
|
||||
sound(delayTime);
|
||||
}
|
||||
|
||||
void GroveSpeaker::sound(int note_delay)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
for (int i = 0; i < 100; i++)
|
||||
{
|
||||
error = mraa_gpio_write (m_gpio, HIGH);
|
||||
usleep(note_delay);
|
||||
error = mraa_gpio_write (m_gpio, LOW);
|
||||
usleep(note_delay);
|
||||
}
|
||||
if (error != MRAA_SUCCESS)
|
||||
mraa_result_print(error);
|
||||
}
|
||||
|
94
src/grovespeaker/grovespeaker.h
Normal file
94
src/grovespeaker/grovespeaker.h
Normal file
@ -0,0 +1,94 @@
|
||||
/*
|
||||
* Author: Zion Orent <sorent@ics.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <map>
|
||||
#include <unistd.h>
|
||||
#include <mraa/gpio.h>
|
||||
|
||||
#define HIGH 1
|
||||
#define LOW 0
|
||||
|
||||
namespace upm {
|
||||
|
||||
typedef struct
|
||||
{
|
||||
int delayTimeLow;
|
||||
int delayTimeLowSharp;
|
||||
int delayTimeMed;
|
||||
int delayTimeMedSharp;
|
||||
int delayTimeHigh;
|
||||
int delayTimeHighSharp;
|
||||
} NoteData;
|
||||
|
||||
/**
|
||||
* @brief C++ API for the GroveSpeaker speaker
|
||||
*
|
||||
* UPM module for the GroveSpeaker.
|
||||
* This sensor can generate different tones and sounds depending on the
|
||||
* frequency of the input signal.
|
||||
*
|
||||
* @ingroup gpio
|
||||
* @snippet grovespeaker.cxx Interesting
|
||||
*/
|
||||
class GroveSpeaker {
|
||||
public:
|
||||
/**
|
||||
* GroveSpeaker Constructor
|
||||
*
|
||||
* @param pin digital pin to use
|
||||
*/
|
||||
GroveSpeaker(int pin);
|
||||
/**
|
||||
* GroveSpeaker Destructor
|
||||
*/
|
||||
~GroveSpeaker();
|
||||
/**
|
||||
* Play all alto notes (lowest notes)
|
||||
*
|
||||
*/
|
||||
void playAll();
|
||||
/**
|
||||
* Play a sound and note whether it's sharp or not
|
||||
*
|
||||
* @param letter character name of note
|
||||
* ('a', 'b', 'c', 'd', 'e', 'f', or 'g')
|
||||
* @param sharp if true, play sharp version of note; otherwise, do not
|
||||
* @param vocalWeight string to determine whether to play low ("low"),
|
||||
* medium ("med"), or high ("high") note
|
||||
*/
|
||||
void playSound(char letter, bool sharp, std::string vocalWeight);
|
||||
|
||||
private:
|
||||
mraa_gpio_context m_gpio;
|
||||
std::map <char, NoteData> m_note_list;
|
||||
void sound(int note_delay);
|
||||
NoteData storeNote(int noteDelayLow, int noteDelayLowSharp,
|
||||
int noteDelayMed, int noteDelayMedSharp,
|
||||
int noteDelayHigh, int noteDelayHighSharp);
|
||||
};
|
||||
}
|
||||
|
||||
|
8
src/grovespeaker/jsupm_grovespeaker.i
Normal file
8
src/grovespeaker/jsupm_grovespeaker.i
Normal file
@ -0,0 +1,8 @@
|
||||
%module jsupm_grovespeaker
|
||||
%include "../upm.i"
|
||||
|
||||
%{
|
||||
#include "grovespeaker.h"
|
||||
%}
|
||||
|
||||
%include "grovespeaker.h"
|
9
src/grovespeaker/pyupm_grovespeaker.i
Normal file
9
src/grovespeaker/pyupm_grovespeaker.i
Normal file
@ -0,0 +1,9 @@
|
||||
%module pyupm_grovespeaker
|
||||
%include "../upm.i"
|
||||
|
||||
%feature("autodoc", "3");
|
||||
|
||||
%include "grovespeaker.h"
|
||||
%{
|
||||
#include "grovespeaker.h"
|
||||
%}
|
Reference in New Issue
Block a user