mirror of
https://github.com/eclipse/upm.git
synced 2025-07-02 01:41:12 +03:00
lcd: jhd1313m1: rewrite to use Lcm1602 as a base class
Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
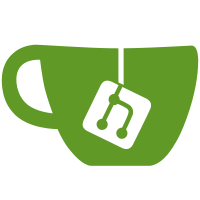
committed by
Mihai Tudor Panu
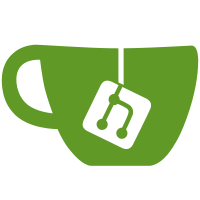
parent
cb62f06197
commit
f133b6eb4c
@ -2,6 +2,8 @@
|
||||
* Author: Yevgeniy Kiveisha <yevgeniy.kiveisha@intel.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Contributions: Jon Trulson <jtrulson@ics.com>
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
@ -33,33 +35,27 @@
|
||||
using namespace upm;
|
||||
|
||||
Jhd1313m1::Jhd1313m1(int bus, int lcdAddress, int rgbAddress)
|
||||
: m_i2c_lcd_rgb(bus), m_i2c_lcd_control(bus)
|
||||
: m_i2c_lcd_rgb(bus), Lcm1602(bus, lcdAddress, false)
|
||||
{
|
||||
m_rgb_address = rgbAddress;
|
||||
m_name = "Jhd1313m1";
|
||||
|
||||
m_lcd_control_address = lcdAddress;
|
||||
|
||||
mraa_result_t ret = m_i2c_lcd_rgb.address(m_rgb_address);
|
||||
if (ret != MRAA_SUCCESS) {
|
||||
fprintf(stderr, "Messed up i2c bus\n");
|
||||
}
|
||||
|
||||
ret = m_i2c_lcd_control.address(m_lcd_control_address);
|
||||
if (ret != MRAA_SUCCESS) {
|
||||
fprintf(stderr, "Messed up i2c bus\n");
|
||||
}
|
||||
|
||||
usleep(50000);
|
||||
ret = m_i2c_lcd_control.writeReg(LCD_CMD, LCD_FUNCTIONSET | LCD_2LINE);
|
||||
ret = command(LCD_FUNCTIONSET | LCD_2LINE);
|
||||
|
||||
if (!ret) {
|
||||
ret = m_i2c_lcd_control.writeReg(LCD_CMD, LCD_FUNCTIONSET | LCD_2LINE);
|
||||
ret = command(LCD_FUNCTIONSET | LCD_2LINE);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
}
|
||||
|
||||
usleep(100);
|
||||
ret = m_i2c_lcd_control.writeReg(LCD_CMD, LCD_DISPLAYCONTROL | LCD_DISPLAYON);
|
||||
ret = displayOn();
|
||||
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
|
||||
usleep(100);
|
||||
@ -67,8 +63,7 @@ Jhd1313m1::Jhd1313m1(int bus, int lcdAddress, int rgbAddress)
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
|
||||
usleep(2000);
|
||||
ret =
|
||||
m_i2c_lcd_control.writeReg(LCD_CMD, LCD_ENTRYMODESET | LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT);
|
||||
ret = command(LCD_ENTRYMODESET | LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT);
|
||||
UPM_CHECK_MRAA_SUCCESS(ret, "Unable to initialise the LCD controller");
|
||||
|
||||
ret = m_i2c_lcd_rgb.writeReg(0, 0);
|
||||
@ -118,71 +113,18 @@ mraa_result_t
|
||||
Jhd1313m1::scroll(bool direction)
|
||||
{
|
||||
if (direction) {
|
||||
return m_i2c_lcd_control.writeReg(LCD_CMD, LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVELEFT);
|
||||
return scrollDisplayLeft();
|
||||
} else {
|
||||
return m_i2c_lcd_control.writeReg(LCD_CMD,
|
||||
LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVERIGHT);
|
||||
return scrollDisplayRight();
|
||||
}
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
Jhd1313m1::createChar(uint8_t charSlot, uint8_t charData[])
|
||||
mraa_result_t Jhd1313m1::command(uint8_t cmd)
|
||||
{
|
||||
mraa_result_t error = MRAA_SUCCESS;
|
||||
charSlot &= 0x07; // only have 8 positions we can set
|
||||
error = m_i2c_lcd_control.writeReg(LCD_CMD, LCD_SETCGRAMADDR | (charSlot << 3));
|
||||
if (error == MRAA_SUCCESS) {
|
||||
for (int i = 0; i < 8; i++) {
|
||||
error = m_i2c_lcd_control.writeReg(LCD_DATA, charData[i]);
|
||||
}
|
||||
}
|
||||
return m_i2c_lcd_control->writeReg(LCD_CMD, cmd);
|
||||
|
||||
return error;
|
||||
}
|
||||
|
||||
/*
|
||||
* **************
|
||||
* virtual area
|
||||
* **************
|
||||
*/
|
||||
mraa_result_t
|
||||
Jhd1313m1::write(std::string msg)
|
||||
mraa_result_t Jhd1313m1::data(uint8_t cmd)
|
||||
{
|
||||
mraa_result_t ret = MRAA_SUCCESS;
|
||||
|
||||
// This usleep fixes an odd bug where the clear function doesn't always work properly
|
||||
usleep(1000);
|
||||
|
||||
for (std::string::size_type i = 0; i < msg.size(); ++i) {
|
||||
ret = m_i2c_lcd_control.writeReg(LCD_DATA, msg[i]);
|
||||
UPM_GOTO_ON_MRAA_FAIL(ret, beach);
|
||||
}
|
||||
|
||||
beach:
|
||||
return ret;
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
Jhd1313m1::setCursor(int row, int column)
|
||||
{
|
||||
mraa_result_t ret;
|
||||
|
||||
int row_addr[] = { 0x80, 0xc0, 0x14, 0x54 };
|
||||
uint8_t offset = ((column % 16) + row_addr[row]);
|
||||
|
||||
ret = m_i2c_lcd_control.writeReg(LCD_CMD, offset);
|
||||
|
||||
return ret;
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
Jhd1313m1::clear()
|
||||
{
|
||||
return m_i2c_lcd_control.writeReg(LCD_CMD, LCD_CLEARDISPLAY);
|
||||
}
|
||||
|
||||
mraa_result_t
|
||||
Jhd1313m1::home()
|
||||
{
|
||||
return m_i2c_lcd_control.writeReg(LCD_CMD, LCD_RETURNHOME);
|
||||
return m_i2c_lcd_control->writeReg(LCD_DATA, cmd);
|
||||
}
|
||||
|
@ -2,6 +2,8 @@
|
||||
* Author: Yevgeniy Kiveisha <yevgeniy.kiveisha@intel.com>
|
||||
* Copyright (c) 2014 Intel Corporation.
|
||||
*
|
||||
* Contributions: Jon Trulson <jtrulson@ics.com>
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
@ -24,8 +26,7 @@
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <mraa/i2c.hpp>
|
||||
#include "lcd.h"
|
||||
#include "lcm1602.h"
|
||||
|
||||
namespace upm
|
||||
{
|
||||
@ -51,7 +52,7 @@ namespace upm
|
||||
* @image html grovergblcd.jpg
|
||||
* @snippet jhd1313m1-lcd.cxx Interesting
|
||||
*/
|
||||
class Jhd1313m1 : public LCD
|
||||
class Jhd1313m1 : public Lcm1602
|
||||
{
|
||||
public:
|
||||
/**
|
||||
@ -82,48 +83,13 @@ class Jhd1313m1 : public LCD
|
||||
* @return Result of operation
|
||||
*/
|
||||
mraa_result_t setColor(uint8_t r, uint8_t g, uint8_t b);
|
||||
/**
|
||||
* Write a string to LCD
|
||||
*
|
||||
* @param msg The std::string to write to display, note only ascii
|
||||
* chars are supported
|
||||
* @return Result of operation
|
||||
*/
|
||||
mraa_result_t write(std::string msg);
|
||||
/**
|
||||
* Set cursor to a coordinate
|
||||
*
|
||||
* @param row The row to set cursor to
|
||||
* @param column The column to set cursor to
|
||||
* @return Result of operation
|
||||
*/
|
||||
mraa_result_t setCursor(int row, int column);
|
||||
/**
|
||||
* Clear display from characters
|
||||
*
|
||||
* @return Result of operatio
|
||||
*/
|
||||
mraa_result_t clear();
|
||||
/**
|
||||
* Return to coordinate 0,0
|
||||
*
|
||||
* @return Result of operation
|
||||
*/
|
||||
mraa_result_t home();
|
||||
|
||||
/**
|
||||
* Create a custom character
|
||||
*
|
||||
* @param charSlot the character slot to write, only 8 are available
|
||||
* @param charData The character data (8 bytes) making up the character
|
||||
* @return Result of operation
|
||||
*/
|
||||
mraa_result_t createChar(uint8_t charSlot, uint8_t charData[]);
|
||||
protected:
|
||||
virtual mraa_result_t command(uint8_t cmd);
|
||||
virtual mraa_result_t data(uint8_t data);
|
||||
|
||||
private:
|
||||
int m_rgb_address;
|
||||
mraa::I2c m_i2c_lcd_rgb;
|
||||
int m_lcd_control_address;
|
||||
mraa::I2c m_i2c_lcd_control;
|
||||
};
|
||||
}
|
||||
|
@ -14,16 +14,16 @@
|
||||
#include "lcd.h"
|
||||
%}
|
||||
|
||||
%include "jhd1313m1.h"
|
||||
%{
|
||||
#include "jhd1313m1.h"
|
||||
%}
|
||||
|
||||
%include "lcm1602.h"
|
||||
%{
|
||||
#include "lcm1602.h"
|
||||
%}
|
||||
|
||||
%include "jhd1313m1.h"
|
||||
%{
|
||||
#include "jhd1313m1.h"
|
||||
%}
|
||||
|
||||
%include "ssd1327.h"
|
||||
%{
|
||||
#include "ssd1327.h"
|
||||
|
@ -55,6 +55,9 @@ Lcm1602::Lcm1602(int bus_in, int addr_in, bool isExpander) :
|
||||
return;
|
||||
}
|
||||
|
||||
// default display control
|
||||
m_displayControl = LCD_DISPLAYON | LCD_CURSOROFF | LCD_BLINKOFF;
|
||||
|
||||
// if we are not dealing with an expander (say via a derived class
|
||||
// like Jhd1313m1), then we do not want to execute the rest of the
|
||||
// code below. Rather, the derived class's constructor should
|
||||
|
@ -230,10 +230,11 @@ class Lcm1602 : public LCD
|
||||
virtual mraa_result_t command(uint8_t cmd);
|
||||
virtual mraa_result_t data(uint8_t data);
|
||||
|
||||
private:
|
||||
int m_lcd_control_address;
|
||||
mraa::I2c* m_i2c_lcd_control;
|
||||
|
||||
private:
|
||||
|
||||
// true if using i2c, false otherwise (gpio)
|
||||
bool m_isI2C;
|
||||
|
||||
|
@ -17,16 +17,16 @@
|
||||
#include "lcd.h"
|
||||
%}
|
||||
|
||||
%include "jhd1313m1.h"
|
||||
%{
|
||||
#include "jhd1313m1.h"
|
||||
%}
|
||||
|
||||
%include "lcm1602.h"
|
||||
%{
|
||||
#include "lcm1602.h"
|
||||
%}
|
||||
|
||||
%include "jhd1313m1.h"
|
||||
%{
|
||||
#include "jhd1313m1.h"
|
||||
%}
|
||||
|
||||
%include "ssd1327.h"
|
||||
%{
|
||||
#include "ssd1327.h"
|
||||
|
Reference in New Issue
Block a user