mirror of
https://github.com/eclipse/upm.git
synced 2025-07-11 22:31:14 +03:00
Initial implementation of iAcceleration
Signed-off-by: Serban Waltter <serban.waltter@rinftech.com> Signed-off-by: Mihai Tudor Panu <mihai.tudor.panu@intel.com>
This commit is contained in:
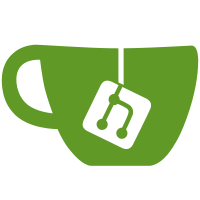
committed by
Mihai Tudor Panu
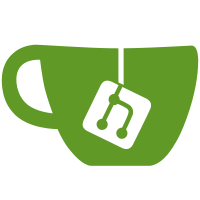
parent
90524273ec
commit
f992876461
@ -24,6 +24,7 @@ set (module_hpp iClock.hpp
|
||||
iMotion.hpp
|
||||
iPressure.hpp
|
||||
iTemperature.hpp
|
||||
iAcceleration.hpp
|
||||
)
|
||||
# Install interfaces headers a bit differently
|
||||
install (FILES ${module_hpp} DESTINATION include/upm/interfaces COMPONENT ${libname})
|
||||
|
47
include/interfaces/iAcceleration.hpp
Normal file
47
include/interfaces/iAcceleration.hpp
Normal file
@ -0,0 +1,47 @@
|
||||
/*
|
||||
* Author: Serban Waltter <serban.waltter@rinftech.com>
|
||||
* Copyright (c) 2018 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <vector>
|
||||
|
||||
namespace upm
|
||||
{
|
||||
/**
|
||||
* @brief Interface for acceleration sensors
|
||||
*/
|
||||
class iAcceleration
|
||||
{
|
||||
public:
|
||||
virtual ~iAcceleration() {}
|
||||
|
||||
/**
|
||||
* Get acceleration values on X, Y and Z axis.
|
||||
* v[0] = X, v[1] = Y, v[2] = Z
|
||||
*
|
||||
* @return vector of 3 floats containing acceleration on each axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration() = 0;
|
||||
};
|
||||
} // upm
|
@ -13,3 +13,4 @@
|
||||
#include "iMotion.hpp"
|
||||
#include "iPressure.hpp"
|
||||
#include "iTemperature.hpp"
|
||||
#include "iAcceleration.hpp"
|
||||
|
@ -20,6 +20,7 @@
|
||||
%interface_impl (upm::iMotion);
|
||||
%interface_impl (upm::iPressure);
|
||||
%interface_impl (upm::iTemperature);
|
||||
%interface_impl (upm::iAcceleration);
|
||||
#endif
|
||||
|
||||
%{
|
||||
@ -37,6 +38,7 @@
|
||||
#include "iMotion.hpp"
|
||||
#include "iPressure.hpp"
|
||||
#include "iTemperature.hpp"
|
||||
#include "iAcceleration.hpp"
|
||||
%}
|
||||
|
||||
%include "iClock.hpp"
|
||||
@ -53,6 +55,7 @@
|
||||
%include "iMotion.hpp"
|
||||
%include "iPressure.hpp"
|
||||
%include "iTemperature.hpp"
|
||||
%include "iAcceleration.hpp"
|
||||
|
||||
/* Java-specific SWIG syntax */
|
||||
#ifdef SWIGJAVA
|
||||
|
@ -106,6 +106,28 @@ std::vector<float> ADXL335::acceleration()
|
||||
return v;
|
||||
}
|
||||
|
||||
std::vector<float> ADXL335::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
int x, y, z;
|
||||
float xVolts, yVolts, zVolts;
|
||||
|
||||
x = mraa_aio_read(m_aioX);
|
||||
y = mraa_aio_read(m_aioY);
|
||||
z = mraa_aio_read(m_aioZ);
|
||||
|
||||
xVolts = float(x) * m_aref / 1024.0;
|
||||
yVolts = float(y) * m_aref / 1024.0;
|
||||
zVolts = float(z) * m_aref / 1024.0;
|
||||
|
||||
v[0] = (xVolts - m_zeroX) / ADXL335_SENSITIVITY;
|
||||
v[1] = (yVolts - m_zeroY) / ADXL335_SENSITIVITY;
|
||||
v[2] = (zVolts - m_zeroZ) / ADXL335_SENSITIVITY;
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
void ADXL335::calibrate()
|
||||
{
|
||||
// make sure the sensor is still before running calibration.
|
||||
|
@ -30,6 +30,8 @@
|
||||
#include <vector>
|
||||
#include <mraa/aio.h>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define ADXL335_DEFAULT_AREF 5.0
|
||||
#define ADXL335_SENSITIVITY 0.25 // 0.25v/g
|
||||
|
||||
@ -60,7 +62,7 @@ namespace upm {
|
||||
* @image html adxl335.jpg
|
||||
* @snippet adxl335.cxx Interesting
|
||||
*/
|
||||
class ADXL335 {
|
||||
class ADXL335: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
* ADXL335 constructor
|
||||
@ -130,6 +132,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> acceleration();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* While the sensor is still, measures the X-axis, Y-axis, and Z-axis
|
||||
* values and uses those values as the zero values.
|
||||
|
@ -131,6 +131,21 @@ Adxl345::getAcceleration()
|
||||
return &m_accel[0];
|
||||
}
|
||||
|
||||
// std::vector<float>
|
||||
// Adxl345::getAcceleration()
|
||||
// {
|
||||
// update();
|
||||
|
||||
// std::vector<float> v(3);
|
||||
|
||||
// for(int i = 0; i < 3; i++)
|
||||
// {
|
||||
// v[i] = m_rawaccel[i] * m_offsets[i];
|
||||
// }
|
||||
|
||||
// return v;
|
||||
// }
|
||||
|
||||
int16_t*
|
||||
Adxl345::getRawValues()
|
||||
{
|
||||
|
@ -25,6 +25,8 @@
|
||||
|
||||
#include <mraa/i2c.hpp>
|
||||
|
||||
// #include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define READ_BUFFER_LENGTH 6
|
||||
|
||||
namespace upm {
|
||||
@ -57,6 +59,7 @@ namespace upm {
|
||||
* @image html adxl345.jpeg
|
||||
* @snippet adxl345.cxx Interesting
|
||||
*/
|
||||
// class Adxl345: virtual public iAcceleration {
|
||||
class Adxl345 {
|
||||
public:
|
||||
/**
|
||||
@ -78,6 +81,13 @@ public:
|
||||
*/
|
||||
float* getAcceleration();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
// virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Returns a pointer to an int[3] that contains the raw register values
|
||||
* for X, Y, and Z
|
||||
|
@ -174,6 +174,18 @@ std::vector<float> BMA220::getAccelerometer()
|
||||
return v;
|
||||
}
|
||||
|
||||
std::vector<float> BMA220::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
update();
|
||||
v[0] = m_accelX / m_accelScale;
|
||||
v[1] = m_accelY / m_accelScale;
|
||||
v[2] = m_accelZ / m_accelScale;
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
uint8_t BMA220::getChipID()
|
||||
{
|
||||
return readReg(REG_CHIPID);
|
||||
|
@ -29,6 +29,8 @@
|
||||
#include <mraa/i2c.hpp>
|
||||
#include <mraa/gpio.hpp>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define BMA220_I2C_BUS 0
|
||||
#define BMA220_DEFAULT_ADDR 0x0a
|
||||
|
||||
@ -68,7 +70,7 @@ namespace upm {
|
||||
* @snippet bma220.cxx Interesting
|
||||
*/
|
||||
|
||||
class BMA220 {
|
||||
class BMA220: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
// NOTE: reserved registers must not be written into or read from.
|
||||
@ -547,6 +549,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* set the filtering configuration
|
||||
*
|
||||
|
@ -114,6 +114,14 @@ std::vector<float> BMA250E::getAccelerometer()
|
||||
return std::vector<float>(v, v+3);
|
||||
}
|
||||
|
||||
std::vector<float> BMA250E::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
bma250e_get_accelerometer(m_bma250e, &v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
float BMA250E::getTemperature(bool fahrenheit)
|
||||
{
|
||||
float temperature = bma250e_get_temperature(m_bma250e);
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include <mraa/gpio.hpp>
|
||||
#include "bma250e.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -73,7 +75,7 @@ namespace upm {
|
||||
* @snippet bma250e.cxx Interesting
|
||||
*/
|
||||
|
||||
class BMA250E {
|
||||
class BMA250E: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
/**
|
||||
@ -138,6 +140,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return the current measured temperature. Note, this is not
|
||||
* ambient temperature. update() must have been called prior to
|
||||
|
@ -84,6 +84,14 @@ float *BMI160::getAccelerometer()
|
||||
return values;
|
||||
}
|
||||
|
||||
std::vector<float> BMI160::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
bmi160_get_accelerometer(m_bmi160, &v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
float *BMI160::getGyroscope()
|
||||
{
|
||||
static float values[3]; // x, y, and then z
|
||||
|
@ -25,6 +25,8 @@
|
||||
#include <string>
|
||||
#include "bmi160.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define BMI160_I2C_BUS 0
|
||||
#define BMI160_DEFAULT_I2C_ADDR 0x69
|
||||
|
||||
@ -72,7 +74,7 @@ namespace upm {
|
||||
*
|
||||
* @snippet bmi160.cxx Interesting
|
||||
*/
|
||||
class BMI160 {
|
||||
class BMI160: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
/**
|
||||
@ -142,6 +144,13 @@ namespace upm {
|
||||
*/
|
||||
void getAccelerometer(float *x, float *y, float *z);
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Get the Gyroscope values. This function returns a pointer to 3
|
||||
* floating point values: X, Y, and Z, in that order. The values
|
||||
|
@ -101,6 +101,14 @@ std::vector<float> BMC150::getAccelerometer()
|
||||
return {0, 0, 0};
|
||||
}
|
||||
|
||||
std::vector<float> BMC150::getAcceleration()
|
||||
{
|
||||
if (m_accel)
|
||||
return m_accel->getAcceleration();
|
||||
else
|
||||
return {0, 0, 0};
|
||||
}
|
||||
|
||||
void BMC150::getMagnetometer(float *x, float *y, float *z)
|
||||
{
|
||||
if (m_mag)
|
||||
|
@ -29,6 +29,8 @@
|
||||
#include "bma250e.hpp"
|
||||
#include "bmm150.hpp"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define BMC150_DEFAULT_BUS 0
|
||||
#define BMC150_DEFAULT_ACC_ADDR 0x10
|
||||
#define BMC150_DEFAULT_MAG_ADDR 0x12
|
||||
@ -70,7 +72,7 @@ namespace upm {
|
||||
* @snippet bmx055-bmc150.cxx Interesting
|
||||
*/
|
||||
|
||||
class BMC150 {
|
||||
class BMC150: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
* BMC150 constructor.
|
||||
@ -164,6 +166,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return magnetometer data in micro-Teslas (uT). update() must
|
||||
* have been called prior to calling this method.
|
||||
|
@ -103,6 +103,14 @@ std::vector<float> BMI055::getAccelerometer()
|
||||
return {0, 0, 0};
|
||||
}
|
||||
|
||||
std::vector<float> BMI055::getAcceleration()
|
||||
{
|
||||
if (m_accel)
|
||||
return m_accel->getAcceleration();
|
||||
else
|
||||
return {0, 0, 0};
|
||||
}
|
||||
|
||||
void BMI055::getGyroscope(float *x, float *y, float *z)
|
||||
{
|
||||
if (m_gyro)
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include "bma250e.hpp"
|
||||
#include "bmg160.hpp"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -64,7 +66,7 @@ namespace upm {
|
||||
* @snippet bmx055-bmi055.cxx Interesting
|
||||
*/
|
||||
|
||||
class BMI055 {
|
||||
class BMI055: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
* BMI055 constructor.
|
||||
@ -152,6 +154,13 @@ namespace upm {
|
||||
*/
|
||||
void getAccelerometer(float *x, float *y, float *z);
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return accelerometer data in gravities in the form of a
|
||||
* floating point vector. update() must have been called prior to
|
||||
|
@ -121,6 +121,14 @@ std::vector<float> BMX055::getAccelerometer()
|
||||
return {0, 0, 0};
|
||||
}
|
||||
|
||||
std::vector<float> BMX055::getAcceleration()
|
||||
{
|
||||
if (m_accel)
|
||||
return m_accel->getAcceleration();
|
||||
else
|
||||
return {0, 0, 0};
|
||||
}
|
||||
|
||||
void BMX055::getGyroscope(float *x, float *y, float *z)
|
||||
{
|
||||
if (m_gyro)
|
||||
|
@ -32,6 +32,8 @@
|
||||
#include "bmg160.hpp"
|
||||
#include "bmm150.hpp"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define BMX055_DEFAULT_MAG_I2C_ADDR 0x12
|
||||
|
||||
namespace upm {
|
||||
@ -76,7 +78,7 @@ namespace upm {
|
||||
* @snippet bmx055.cxx Interesting
|
||||
*/
|
||||
|
||||
class BMX055 {
|
||||
class BMX055: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
* BMX055 constructor.
|
||||
@ -195,6 +197,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return gyroscope data in degrees per second. update() must
|
||||
* have been called prior to calling this method.
|
||||
|
@ -388,6 +388,14 @@ vector<float> BNO055::getAccelerometer()
|
||||
return vector<float>(v, v+3);
|
||||
}
|
||||
|
||||
std::vector<float> BNO055::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
bno055_get_accelerometer(m_bno055, &v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
void BNO055::getMagnetometer(float *x, float *y, float *z)
|
||||
{
|
||||
bno055_get_magnetometer(m_bno055, x, y, z);
|
||||
|
@ -28,6 +28,8 @@
|
||||
#include <vector>
|
||||
#include "bno055.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -100,7 +102,7 @@ namespace upm {
|
||||
* @snippet bno055.cxx Interesting
|
||||
*/
|
||||
|
||||
class BNO055 {
|
||||
class BNO055: virtual public iAcceleration {
|
||||
|
||||
public:
|
||||
/**
|
||||
@ -420,6 +422,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return uncompensated magnetometer data (non-fusion). In fusion
|
||||
* modes, this data will be of little value. The returned values
|
||||
|
@ -602,8 +602,14 @@ void H3LIS331DL::getXYZ(int *x, int *y, int*z)
|
||||
|
||||
std::vector<float> H3LIS331DL::getAcceleration()
|
||||
{
|
||||
update();
|
||||
std::vector<float> v(3);
|
||||
getAcceleration(&v[0], &v[1], &v[2]);
|
||||
|
||||
const float gains = 0.003; // Seeed magic number?
|
||||
|
||||
v[0] = float(m_rawX - m_adjX) * gains;
|
||||
v[1] = float(m_rawY - m_adjY) * gains;
|
||||
v[2] = float(m_rawZ - m_adjZ) * gains;
|
||||
return v;
|
||||
}
|
||||
|
||||
|
@ -28,6 +28,8 @@
|
||||
#include <mraa/common.hpp>
|
||||
#include <mraa/i2c.hpp>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define H3LIS331DL_I2C_BUS 0
|
||||
#define H3LIS331DL_DEFAULT_I2C_ADDR 0x18
|
||||
|
||||
@ -56,7 +58,7 @@ namespace upm {
|
||||
* @image html h3lis331dl.jpg
|
||||
* @snippet h3lis331dl.cxx Interesting
|
||||
*/
|
||||
class H3LIS331DL {
|
||||
class H3LIS331DL: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
/**
|
||||
|
@ -108,6 +108,14 @@ std::vector<float> LIS2DS12::getAccelerometer()
|
||||
return std::vector<float>(v, v+3);
|
||||
}
|
||||
|
||||
std::vector<float> LIS2DS12::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
lis2ds12_get_accelerometer(m_lis2ds12, &v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
float LIS2DS12::getTemperature(bool fahrenheit)
|
||||
{
|
||||
float temperature = lis2ds12_get_temperature(m_lis2ds12);
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include <mraa/gpio.hpp>
|
||||
#include "lis2ds12.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -70,7 +72,7 @@ namespace upm {
|
||||
* @snippet lis2ds12.cxx Interesting
|
||||
*/
|
||||
|
||||
class LIS2DS12 {
|
||||
class LIS2DS12: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
/**
|
||||
@ -135,6 +137,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return the current measured temperature. Note, this is not
|
||||
* ambient temperature. update() must have been called prior to
|
||||
|
@ -234,6 +234,15 @@ LIS3DH::getAccelerometer()
|
||||
return std::vector<float>(v, v + 3);
|
||||
}
|
||||
|
||||
std::vector<float>
|
||||
LIS3DH::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
lis3dh_get_accelerometer(m_lis3dh, &v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
float
|
||||
LIS3DH::getTemperature(bool fahrenheit)
|
||||
{
|
||||
|
@ -35,6 +35,8 @@
|
||||
#include "lis3dh.h"
|
||||
#include <mraa/gpio.hpp>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm
|
||||
{
|
||||
/**
|
||||
@ -72,7 +74,7 @@ namespace upm
|
||||
* @snippet lis3dh.cxx Interesting
|
||||
*/
|
||||
|
||||
class LIS3DH
|
||||
class LIS3DH: virtual public iAcceleration
|
||||
{
|
||||
public:
|
||||
/**
|
||||
@ -304,6 +306,13 @@ class LIS3DH
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return the current measured temperature. Note, this is not
|
||||
* ambient temperature. update() must have been called prior to
|
||||
|
@ -109,6 +109,14 @@ std::vector<float> LSM303AGR::getAccelerometer()
|
||||
return std::vector<float>(v, v+3);
|
||||
}
|
||||
|
||||
std::vector<float> LSM303AGR::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
lsm303agr_get_accelerometer(m_lsm303agr, &v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
float LSM303AGR::getTemperature()
|
||||
{
|
||||
return lsm303agr_get_temperature(m_lsm303agr);
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include <mraa/gpio.hpp>
|
||||
#include "lsm303agr.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -65,7 +67,7 @@ namespace upm {
|
||||
* @snippet lsm303agr.cxx Interesting
|
||||
*/
|
||||
|
||||
class LSM303AGR {
|
||||
class LSM303AGR: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
* LSM303AGR constructor
|
||||
@ -149,6 +151,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return temperature data in degrees Celsius. NOTE: This is
|
||||
* not the ambient room temperature. update() must have been
|
||||
|
@ -109,6 +109,15 @@ std::vector<float> LSM303D::getAccelerometer()
|
||||
return std::vector<float>(v, v+3);
|
||||
}
|
||||
|
||||
std::vector<float> LSM303D::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
lsm303d_get_accelerometer(m_lsm303d, &v[0], &v[1], &v[2]);
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
float LSM303D::getTemperature()
|
||||
{
|
||||
return lsm303d_get_temperature(m_lsm303d);
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include <mraa/gpio.hpp>
|
||||
#include "lsm303d.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -65,7 +67,7 @@ namespace upm {
|
||||
* @snippet lsm303d.cxx Interesting
|
||||
*/
|
||||
|
||||
class LSM303D {
|
||||
class LSM303D: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
* LSM303D constructor
|
||||
@ -142,6 +144,13 @@ namespace upm {
|
||||
* that order
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return temperature data in degrees Celsius. NOTE: This is
|
||||
|
@ -109,6 +109,14 @@ std::vector<float> LSM6DS3H::getAccelerometer()
|
||||
return std::vector<float>(v, v+3);
|
||||
}
|
||||
|
||||
std::vector<float> LSM6DS3H::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
lsm6ds3h_get_accelerometer(m_lsm6ds3h, &v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
void LSM6DS3H::getGyroscope(float *x, float *y, float *z)
|
||||
{
|
||||
lsm6ds3h_get_gyroscope(m_lsm6ds3h, x, y, z);
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include <mraa/gpio.hpp>
|
||||
#include "lsm6ds3h.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -64,7 +66,7 @@ namespace upm {
|
||||
* @snippet lsm6ds3h.cxx Interesting
|
||||
*/
|
||||
|
||||
class LSM6DS3H {
|
||||
class LSM6DS3H: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
/**
|
||||
@ -129,6 +131,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return gyroscope data in degrees per second (DPS).
|
||||
* update() must have been called prior to calling this
|
||||
|
@ -109,6 +109,15 @@ std::vector<float> LSM6DSL::getAccelerometer()
|
||||
return std::vector<float>(v, v+3);
|
||||
}
|
||||
|
||||
std::vector<float> LSM6DSL::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
lsm6dsl_get_accelerometer(m_lsm6dsl, &v[0], &v[1], &v[2]);
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
void LSM6DSL::getGyroscope(float *x, float *y, float *z)
|
||||
{
|
||||
lsm6dsl_get_gyroscope(m_lsm6dsl, x, y, z);
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include <mraa/gpio.hpp>
|
||||
#include "lsm6dsl.h"
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
@ -63,7 +65,7 @@ namespace upm {
|
||||
* @snippet lsm6dsl.cxx Interesting
|
||||
*/
|
||||
|
||||
class LSM6DSL {
|
||||
class LSM6DSL: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
/**
|
||||
@ -128,6 +130,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Return gyroscope data in degrees per second (DPS).
|
||||
* update() must have been called prior to calling this
|
||||
|
@ -629,6 +629,13 @@ std::vector<float> LSM9DS0::getAccelerometer()
|
||||
return v;
|
||||
}
|
||||
|
||||
std::vector<float> LSM9DS0::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
getAccelerometer(&v[0], &v[1], &v[2]);
|
||||
return v;
|
||||
}
|
||||
|
||||
std::vector<float> LSM9DS0::getGyroscope()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
@ -30,6 +30,8 @@
|
||||
|
||||
#include <mraa/gpio.hpp>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define LSM9DS0_I2C_BUS 1
|
||||
#define LSM9DS0_DEFAULT_XM_ADDR 0x1d
|
||||
#define LSM9DS0_DEFAULT_GYRO_ADDR 0x6b
|
||||
@ -77,7 +79,7 @@ namespace upm {
|
||||
* @snippet lsm9ds0.cxx Interesting
|
||||
*/
|
||||
|
||||
class LSM9DS0 {
|
||||
class LSM9DS0: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
// NOTE: reserved registers must not be written into or permanent
|
||||
@ -1274,6 +1276,13 @@ namespace upm {
|
||||
*/
|
||||
std::vector<float> getAccelerometer();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* get the gyroscope values in degrees per second
|
||||
*
|
||||
|
@ -112,6 +112,15 @@ void MMA7361::getAcceleration(float *x, float *y, float *z)
|
||||
mma7361_get_acceleration(m_mma7361, x, y, z);
|
||||
}
|
||||
|
||||
// std::vector<float> MMA7361::getAcceleration()
|
||||
// {
|
||||
// std::vector<float> v(3);
|
||||
|
||||
// mma7361_get_acceleration(m_mma7361, &v[0], &v[1], &v[2]);
|
||||
|
||||
// return v;
|
||||
// }
|
||||
|
||||
float *MMA7361::getAcceleration()
|
||||
{
|
||||
static float data[3];
|
||||
|
@ -32,6 +32,8 @@
|
||||
|
||||
#include "mma7361.h"
|
||||
|
||||
// #include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
/**
|
||||
* @brief MMA7361 Analog Accelerometer
|
||||
@ -57,6 +59,7 @@ namespace upm {
|
||||
* @snippet mma7361.cxx Interesting
|
||||
*/
|
||||
|
||||
// class MMA7361: virtual public iAcceleration {
|
||||
class MMA7361 {
|
||||
public:
|
||||
|
||||
@ -150,6 +153,13 @@ namespace upm {
|
||||
*/
|
||||
void getAcceleration(float *x, float *y, float *z);
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
// virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Get computed acceleration from the sensor. update() must have
|
||||
* been called prior to calling this function.
|
||||
|
@ -140,6 +140,46 @@ std::vector<short> MMA7455::readData() {
|
||||
return v;
|
||||
}
|
||||
|
||||
std::vector<float> MMA7455::getAcceleration() {
|
||||
std::vector<float> v(3);
|
||||
accelData xyz;
|
||||
int nBytes = 0;
|
||||
|
||||
/*do {
|
||||
nBytes = i2cReadReg (MMA7455_STATUS, &data, 0x1);
|
||||
} while ( !(data & MMA7455_DRDY) && nBytes == mraa::SUCCESS);
|
||||
|
||||
if (nBytes == mraa::SUCCESS) {
|
||||
std::cout << "NO_GDB :: 1" << std::endl;
|
||||
return mraa::SUCCESS;
|
||||
}*/
|
||||
|
||||
nBytes = i2cReadReg (MMA7455_XOUTL, (unsigned char *) &xyz, 0x6);
|
||||
if (nBytes == 0) {
|
||||
std::cout << "NO_GDB :: 2" << std::endl;
|
||||
//return mraa::ERROR_UNSPECIFIED;
|
||||
}
|
||||
|
||||
if (xyz.reg.x_msb & 0x02) {
|
||||
xyz.reg.x_msb |= 0xFC;
|
||||
}
|
||||
|
||||
if (xyz.reg.y_msb & 0x02) {
|
||||
xyz.reg.y_msb |= 0xFC;
|
||||
}
|
||||
|
||||
if (xyz.reg.z_msb & 0x02) {
|
||||
xyz.reg.z_msb |= 0xFC;
|
||||
}
|
||||
|
||||
// The result is the g-force in units of 64 per 'g'.
|
||||
v[0] = (float)xyz.value.x;
|
||||
v[1] = (float)xyz.value.y;
|
||||
v[2] = (float)xyz.value.z;
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
int
|
||||
MMA7455::i2cReadReg (unsigned char reg, uint8_t *buffer, int len) {
|
||||
if (mraa::SUCCESS != m_i2ControlCtx.writeByte(reg)) {
|
||||
|
@ -27,6 +27,8 @@
|
||||
#include <vector>
|
||||
#include <mraa/i2c.hpp>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define ADDR 0x1D // device address
|
||||
|
||||
// Register names according to the datasheet.
|
||||
@ -171,7 +173,7 @@ typedef union {
|
||||
* @image html mma7455.jpg
|
||||
* @snippet mma7455.cxx Interesting
|
||||
*/
|
||||
class MMA7455 {
|
||||
class MMA7455: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
* Instantiates an MMA7455 object
|
||||
@ -216,6 +218,13 @@ class MMA7455 {
|
||||
*/
|
||||
std::vector<short> readData ();
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Internal function for reading I2C data
|
||||
*
|
||||
|
@ -190,3 +190,14 @@ void MMA7660::getAcceleration(float *ax, float *ay, float *az)
|
||||
": mma7660_get_acceleration() failed");
|
||||
}
|
||||
|
||||
std::vector<float> MMA7660::getAcceleration()
|
||||
{
|
||||
std::vector<float> v(3);
|
||||
|
||||
if (mma7660_get_acceleration(m_mma7660, &v[0], &v[1], &v[2]))
|
||||
throw std::runtime_error(std::string(__FUNCTION__) +
|
||||
": mma7660_get_acceleration() failed");
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
|
@ -26,6 +26,7 @@
|
||||
#include <vector>
|
||||
|
||||
#include "mma7660.h"
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
namespace upm {
|
||||
|
||||
@ -58,7 +59,7 @@ namespace upm {
|
||||
* @image html mma7660.jpg
|
||||
* @snippet mma7660.cxx Interesting
|
||||
*/
|
||||
class MMA7660 {
|
||||
class MMA7660: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
/**
|
||||
@ -109,6 +110,13 @@ namespace upm {
|
||||
*/
|
||||
void getAcceleration(float *ax, float *ay, float *az);
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Reads an axis, verifying its validity. The value passed must
|
||||
* be one of REG_XOUT, REG_YOUT, or REG_ZOUT.
|
||||
@ -199,20 +207,6 @@ namespace upm {
|
||||
*/
|
||||
bool setSampleRate(MMA7660_AUTOSLEEP_T sr);
|
||||
|
||||
/**
|
||||
* Reads the current acceleration values. The returned memory
|
||||
* is statically allocated and will be overwritten on each
|
||||
* call.
|
||||
*
|
||||
* @return std::vector containing x, y, z.
|
||||
*/
|
||||
std::vector<float> getAcceleration()
|
||||
{
|
||||
std::vector<float> values(3);
|
||||
getAcceleration(&values[0], &values[1], &values[2]);
|
||||
return values;
|
||||
}
|
||||
|
||||
/**
|
||||
* Reads the current value of conversion. The returned memory
|
||||
* is statically allocated and will be overwritten on each
|
||||
|
@ -328,6 +328,19 @@ MMA8X5X::getZ(int bSampleData)
|
||||
return s_data->z;
|
||||
}
|
||||
|
||||
std::vector<float>
|
||||
MMA8X5X::getAcceleration()
|
||||
{
|
||||
sampleData();
|
||||
|
||||
std::vector<float> v(3);
|
||||
v[0] = s_data->x;
|
||||
v[1] = s_data->y;
|
||||
v[2] = s_data->z;
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
int
|
||||
MMA8X5X::getData(mma8x5x_data_t* data, int bSampleData)
|
||||
{
|
||||
|
@ -31,6 +31,8 @@
|
||||
#include <stdint.h>
|
||||
#include <stdbool.h>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
/* Supported devices by this driver */
|
||||
#define MMA8X5X_DEVICE_ID_MMA8652 0x4a
|
||||
#define MMA8X5X_DEVICE_ID_MMA8653 0x5a
|
||||
@ -341,7 +343,7 @@ typedef struct {
|
||||
*
|
||||
* @snippet mma8x5x.cxx Interesting
|
||||
*/
|
||||
class MMA8X5X {
|
||||
class MMA8X5X: virtual public iAcceleration {
|
||||
public:
|
||||
/**
|
||||
*
|
||||
@ -448,6 +450,13 @@ class MMA8X5X {
|
||||
*/
|
||||
int16_t getZ(int bSampleData = 0);
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* Get sensor values
|
||||
*
|
||||
|
@ -301,6 +301,18 @@ void MPU60X0::getAccelerometer(float *x, float *y, float *z)
|
||||
*z = m_accelZ / m_accelScale;
|
||||
}
|
||||
|
||||
std::vector<float> MPU60X0::getAcceleration()
|
||||
{
|
||||
update();
|
||||
|
||||
std::vector<float> v(3);
|
||||
v[0] = m_accelX / m_accelScale;
|
||||
v[1] = m_accelY / m_accelScale;
|
||||
v[2] = m_accelZ / m_accelScale;
|
||||
|
||||
return v;
|
||||
}
|
||||
|
||||
void MPU60X0::getGyroscope(float *x, float *y, float *z)
|
||||
{
|
||||
if (x)
|
||||
|
@ -24,11 +24,14 @@
|
||||
#pragma once
|
||||
|
||||
#include <string>
|
||||
#include <vector>
|
||||
#include <mraa/common.hpp>
|
||||
#include <mraa/i2c.hpp>
|
||||
|
||||
#include <mraa/gpio.hpp>
|
||||
|
||||
#include <interfaces/iAcceleration.hpp>
|
||||
|
||||
#define MPU60X0_I2C_BUS 0
|
||||
#define MPU60X0_DEFAULT_I2C_ADDR 0x68
|
||||
|
||||
@ -59,7 +62,7 @@ namespace upm {
|
||||
* @image html mpu60x0.jpg
|
||||
* @snippet mpu9150-mpu60x0.cxx Interesting
|
||||
*/
|
||||
class MPU60X0 {
|
||||
class MPU60X0: virtual public iAcceleration {
|
||||
public:
|
||||
|
||||
// NOTE: These enums were composed from both the mpu6050 and
|
||||
@ -791,6 +794,13 @@ namespace upm {
|
||||
*/
|
||||
void getAccelerometer(float *x, float *y, float *z);
|
||||
|
||||
/**
|
||||
* get acceleration values
|
||||
*
|
||||
* @return stl vector of size 3 representing the 3 axis
|
||||
*/
|
||||
virtual std::vector<float> getAcceleration();
|
||||
|
||||
/**
|
||||
* get the gyroscope values
|
||||
*
|
||||
|
Reference in New Issue
Block a user