mirror of
https://github.com/eclipse/upm.git
synced 2025-07-08 04:41:12 +03:00
mpu9250: Initial implementation
This driver was implemented using a Grove IMU 9DOF V2.0 (mpu9250). Signed-off-by: Jon Trulson <jtrulson@ics.com> Signed-off-by: sisinty sasmita patra <sisinty.s.patra@intel.com>
This commit is contained in:
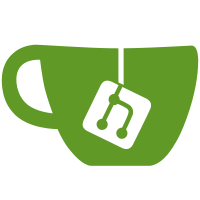
committed by
sisinty sasmita patra
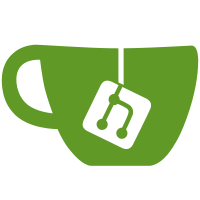
parent
1e5b755b99
commit
76f7abb112
@ -133,6 +133,7 @@ add_executable (mpu60x0-example mpu60x0.cxx)
|
||||
add_executable (ak8975-example ak8975.cxx)
|
||||
add_executable (lsm9ds0-example lsm9ds0.cxx)
|
||||
add_executable (eboled-example eboled.cxx)
|
||||
add_executable (mpu9250-example mpu9250.cxx)
|
||||
add_executable (hyld9767-example hyld9767.cxx)
|
||||
add_executable (mg811-example mg811.cxx)
|
||||
add_executable (wheelencoder-example wheelencoder.cxx)
|
||||
@ -379,6 +380,7 @@ target_link_libraries (mpu60x0-example mpu9150 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (ak8975-example mpu9150 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (lsm9ds0-example lsm9ds0 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (eboled-example i2clcd ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (mpu9250-example mpu9150 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (hyld9767-example hyld9767 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (mg811-example mg811 ${CMAKE_THREAD_LIBS_INIT})
|
||||
target_link_libraries (wheelencoder-example wheelencoder ${CMAKE_THREAD_LIBS_INIT})
|
||||
|
81
examples/c++/mpu9250.cxx
Normal file
81
examples/c++/mpu9250.cxx
Normal file
@ -0,0 +1,81 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <unistd.h>
|
||||
#include <iostream>
|
||||
#include <signal.h>
|
||||
#include "mpu9250.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
int shouldRun = true;
|
||||
|
||||
void sig_handler(int signo)
|
||||
{
|
||||
if (signo == SIGINT)
|
||||
shouldRun = false;
|
||||
}
|
||||
|
||||
|
||||
int main(int argc, char **argv)
|
||||
{
|
||||
signal(SIGINT, sig_handler);
|
||||
//! [Interesting]
|
||||
|
||||
upm::MPU9250 *sensor = new upm::MPU9250();
|
||||
|
||||
sensor->init();
|
||||
|
||||
while (shouldRun)
|
||||
{
|
||||
sensor->update();
|
||||
|
||||
float x, y, z;
|
||||
|
||||
sensor->getAccelerometer(&x, &y, &z);
|
||||
cout << "Accelerometer: ";
|
||||
cout << "AX: " << x << " AY: " << y << " AZ: " << z << endl;
|
||||
|
||||
sensor->getGyroscope(&x, &y, &z);
|
||||
cout << "Gryoscope: ";
|
||||
cout << "GX: " << x << " GY: " << y << " GZ: " << z << endl;
|
||||
|
||||
sensor->getMagnetometer(&x, &y, &z);
|
||||
cout << "Magnetometer: ";
|
||||
cout << "MX = " << x << " MY = " << y << " MZ = " << z << endl;
|
||||
|
||||
cout << "Temperature: " << sensor->getTemperature() << endl;
|
||||
cout << endl;
|
||||
|
||||
usleep(500000);
|
||||
}
|
||||
|
||||
//! [Interesting]
|
||||
|
||||
cout << "Exiting..." << endl;
|
||||
|
||||
delete sensor;
|
||||
|
||||
return 0;
|
||||
}
|
76
examples/javascript/mpu9250.js
Normal file
76
examples/javascript/mpu9250.js
Normal file
@ -0,0 +1,76 @@
|
||||
/*jslint node:true, vars:true, bitwise:true, unparam:true */
|
||||
/*jshint unused:true */
|
||||
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
|
||||
var sensorObj = require('jsupm_mpu9150');
|
||||
|
||||
// Instantiate an MPU9105 on default I2C bus and address
|
||||
var sensor = new sensorObj.MPU9250();
|
||||
|
||||
// Initialize the device with default values
|
||||
sensor.init();
|
||||
|
||||
var x = new sensorObj.new_floatp();
|
||||
var y = new sensorObj.new_floatp();
|
||||
var z = new sensorObj.new_floatp();
|
||||
|
||||
// Output data every half second until interrupted
|
||||
setInterval(function()
|
||||
{
|
||||
sensor.update();
|
||||
|
||||
sensor.getAccelerometer(x, y, z);
|
||||
console.log("Accelerometer: AX: " + sensorObj.floatp_value(x) +
|
||||
" AY: " + sensorObj.floatp_value(y) +
|
||||
" AZ: " + sensorObj.floatp_value(z));
|
||||
|
||||
sensor.getGyroscope(x, y, z);
|
||||
console.log("Gyroscope: GX: " + sensorObj.floatp_value(x) +
|
||||
" AY: " + sensorObj.floatp_value(y) +
|
||||
" AZ: " + sensorObj.floatp_value(z));
|
||||
|
||||
sensor.getMagnetometer(x, y, z);
|
||||
console.log("Magnetometer: MX: " + sensorObj.floatp_value(x) +
|
||||
" MY: " + sensorObj.floatp_value(y) +
|
||||
" MZ: " + sensorObj.floatp_value(z));
|
||||
|
||||
console.log("Temperature: " + sensor.getTemperature());
|
||||
|
||||
console.log();
|
||||
|
||||
}, 500);
|
||||
|
||||
// exit on ^C
|
||||
process.on('SIGINT', function()
|
||||
{
|
||||
sensor = null;
|
||||
sensorObj.cleanUp();
|
||||
sensorObj = null;
|
||||
console.log("Exiting.");
|
||||
process.exit(0);
|
||||
});
|
||||
|
70
examples/python/mpu9250.py
Normal file
70
examples/python/mpu9250.py
Normal file
@ -0,0 +1,70 @@
|
||||
#!/usr/bin/python
|
||||
# Author: Jon Trulson <jtrulson@ics.com>
|
||||
# Copyright (c) 2015 Intel Corporation.
|
||||
#
|
||||
# Permission is hereby granted, free of charge, to any person obtaining
|
||||
# a copy of this software and associated documentation files (the
|
||||
# "Software"), to deal in the Software without restriction, including
|
||||
# without limitation the rights to use, copy, modify, merge, publish,
|
||||
# distribute, sublicense, and/or sell copies of the Software, and to
|
||||
# permit persons to whom the Software is furnished to do so, subject to
|
||||
# the following conditions:
|
||||
#
|
||||
# The above copyright notice and this permission notice shall be
|
||||
# included in all copies or substantial portions of the Software.
|
||||
#
|
||||
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
|
||||
import time, sys, signal, atexit
|
||||
import pyupm_mpu9150 as sensorObj
|
||||
|
||||
# Instantiate an MPU9250 on I2C bus 0
|
||||
sensor = sensorObj.MPU9250()
|
||||
|
||||
## Exit handlers ##
|
||||
# This function stops python from printing a stacktrace when you hit control-C
|
||||
def SIGINTHandler(signum, frame):
|
||||
raise SystemExit
|
||||
|
||||
# This function lets you run code on exit
|
||||
def exitHandler():
|
||||
print "Exiting"
|
||||
sys.exit(0)
|
||||
|
||||
# Register exit handlers
|
||||
atexit.register(exitHandler)
|
||||
signal.signal(signal.SIGINT, SIGINTHandler)
|
||||
|
||||
sensor.init()
|
||||
|
||||
x = sensorObj.new_floatp()
|
||||
y = sensorObj.new_floatp()
|
||||
z = sensorObj.new_floatp()
|
||||
|
||||
while (1):
|
||||
sensor.update()
|
||||
sensor.getAccelerometer(x, y, z)
|
||||
print "Accelerometer: AX: ", sensorObj.floatp_value(x),
|
||||
print " AY: ", sensorObj.floatp_value(y),
|
||||
print " AZ: ", sensorObj.floatp_value(z)
|
||||
|
||||
sensor.getGyroscope(x, y, z)
|
||||
print "Gyroscope: GX: ", sensorObj.floatp_value(x),
|
||||
print " GY: ", sensorObj.floatp_value(y),
|
||||
print " GZ: ", sensorObj.floatp_value(z)
|
||||
|
||||
sensor.getMagnetometer(x, y, z)
|
||||
print "Magnetometer: MX: ", sensorObj.floatp_value(x),
|
||||
print " MY: ", sensorObj.floatp_value(y),
|
||||
print " MZ: ", sensorObj.floatp_value(z)
|
||||
|
||||
print "Temperature: ", sensor.getTemperature()
|
||||
print
|
||||
|
||||
time.sleep(.5)
|
@ -1,5 +1,5 @@
|
||||
set (libname "mpu9150")
|
||||
set (libdescription "gyro, acceleromter and magnometer sensor based on mpu9150")
|
||||
set (module_src ${libname}.cxx ak8975.cxx mpu60x0.cxx)
|
||||
set (module_h ${libname}.h ak8975.h mpu60x0.h)
|
||||
set (module_src ${libname}.cxx ak8975.cxx mpu60x0.cxx mpu9250.cxx)
|
||||
set (module_h ${libname}.h ak8975.h mpu60x0.h mpu9250.h)
|
||||
upm_module_init()
|
||||
|
@ -23,3 +23,8 @@
|
||||
#include "mpu9150.h"
|
||||
%}
|
||||
|
||||
%include "mpu9250.h"
|
||||
%{
|
||||
#include "mpu9250.h"
|
||||
%}
|
||||
|
||||
|
@ -830,7 +830,7 @@ namespace upm {
|
||||
*
|
||||
* @return the temperature value in degrees Celcius
|
||||
*/
|
||||
float getTemperature();
|
||||
virtual float getTemperature();
|
||||
|
||||
/**
|
||||
* enable onboard temperature measurement sensor
|
||||
|
50
src/mpu9150/mpu9250.cxx
Normal file
50
src/mpu9150/mpu9250.cxx
Normal file
@ -0,0 +1,50 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include <iostream>
|
||||
#include <unistd.h>
|
||||
#include <stdlib.h>
|
||||
|
||||
#include "mpu9250.h"
|
||||
|
||||
using namespace upm;
|
||||
using namespace std;
|
||||
|
||||
MPU9250::MPU9250 (int bus, int address, int magAddress) :
|
||||
MPU9150(bus, address, magAddress)
|
||||
{
|
||||
}
|
||||
|
||||
MPU9250::~MPU9250()
|
||||
{
|
||||
}
|
||||
|
||||
float MPU9250::getTemperature()
|
||||
{
|
||||
// this equation is taken from the datasheet. The 333.87 value was
|
||||
// taken from the adafruit code (it is referenced as
|
||||
// Temp_Sensitivity in the datasheet, but no value is provided there).
|
||||
return (m_temp / 333.87) + 21.0;
|
||||
}
|
||||
|
82
src/mpu9150/mpu9250.h
Normal file
82
src/mpu9150/mpu9250.h
Normal file
@ -0,0 +1,82 @@
|
||||
/*
|
||||
* Author: Jon Trulson <jtrulson@ics.com>
|
||||
* Copyright (c) 2015 Intel Corporation.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining
|
||||
* a copy of this software and associated documentation files (the
|
||||
* "Software"), to deal in the Software without restriction, including
|
||||
* without limitation the rights to use, copy, modify, merge, publish,
|
||||
* distribute, sublicense, and/or sell copies of the Software, and to
|
||||
* permit persons to whom the Software is furnished to do so, subject to
|
||||
* the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be
|
||||
* included in all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
||||
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
||||
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "mpu9150.h"
|
||||
|
||||
#define MPU9250_I2C_BUS 0
|
||||
#define MPU9250_DEFAULT_I2C_ADDR MPU9150_DEFAULT_I2C_ADDR
|
||||
|
||||
|
||||
namespace upm {
|
||||
|
||||
/**
|
||||
* @library mpu9150
|
||||
* @sensor mpu9250
|
||||
* @comname MPU9250 Inertial Measurement Unit
|
||||
* @altname Grove IMU 9DOF V2
|
||||
* @type accelerometer compass
|
||||
* @man seeed
|
||||
* @web http://www.seeedstudio.com/wiki/Grove_-_IMU_9DOF_v2.0
|
||||
* @con i2c gpio
|
||||
*
|
||||
* @brief API for MPU9250 chip (Accelerometer, Gyro and Magnometer Sensor)
|
||||
*
|
||||
* This file defines the MPU9250 interface for libmpu9150
|
||||
*
|
||||
* @snippet mpu9250.cxx Interesting
|
||||
*/
|
||||
|
||||
class MPU9250: public MPU9150
|
||||
{
|
||||
public:
|
||||
/**
|
||||
* MPU9250 constructor
|
||||
*
|
||||
* @param bus I2C bus to use
|
||||
* @param address The address for this device
|
||||
* @param magAddress The address of the connected magnetometer
|
||||
*/
|
||||
MPU9250 (int bus=MPU9250_I2C_BUS, int address=MPU9250_DEFAULT_I2C_ADDR,
|
||||
int magAddress=AK8975_DEFAULT_I2C_ADDR);
|
||||
|
||||
/**
|
||||
* MPU9250 destructor
|
||||
*/
|
||||
~MPU9250 ();
|
||||
|
||||
/**
|
||||
* get the temperature value
|
||||
*
|
||||
* @return the temperature value in degrees Celcius
|
||||
*/
|
||||
float getTemperature();
|
||||
|
||||
protected:
|
||||
|
||||
private:
|
||||
};
|
||||
|
||||
}
|
@ -23,3 +23,8 @@
|
||||
#include "mpu9150.h"
|
||||
%}
|
||||
|
||||
%include "mpu9250.h"
|
||||
%{
|
||||
#include "mpu9250.h"
|
||||
%}
|
||||
|
||||
|
Reference in New Issue
Block a user